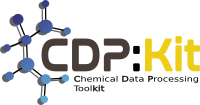 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
31 #ifndef CDPL_DESCR_PATHFINGERPRINTGENERATOR_HPP
32 #define CDPL_DESCR_PATHFINGERPRINTGENERATOR_HPP
39 #include <boost/random/linear_congruential.hpp>
73 static constexpr
unsigned int DEF_ATOM_PROPERTY_FLAGS =
81 static constexpr
unsigned int DEF_BOND_PROPERTY_FLAGS =
265 std::size_t calcBitIndex();
267 typedef std::vector<std::size_t> IndexList;
268 typedef std::vector<std::uint64_t> UInt64Array;
272 std::size_t minPathLength;
273 std::size_t maxPathLength;
277 UInt64Array atomDescriptors;
278 UInt64Array bondDescriptors;
281 UInt64Array fwdPathDescriptor;
282 UInt64Array revPathDescriptor;
283 boost::rand48 randGenerator;
288 #endif // CDPL_DESCR_PATHFINGERPRINTGENERATOR_HPP
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
void setMaxPathLength(std::size_t max_length)
Allows to specify the maximum considered path length.
void setAtomDescriptorFunction(const AtomDescriptorFunction &func)
Allows to specify a custom function for the generation of atom descriptors.
Bond.
Definition: Bond.hpp:50
std::size_t getNumBits() const
Returns the size of the generated fingerprints.
void setMinPathLength(std::size_t min_length)
Allows to specify the minimum length a path must have to contribute to the generated fingerprint.
std::size_t getMaxPathLength() const
Returns the maximum considered path length.
The default functor for the generation of atom descriptors.
Definition: PathFingerprintGenerator.hpp:88
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
PathFingerprintGenerator(const Chem::MolecularGraph &molgraph, Util::BitSet &fp)
Constructs the PathFingerprintGenerator instance and generates the fingerprint of the molecular graph...
void setBondDescriptorFunction(const BondDescriptorFunction &func)
Allows to specify a custom function for the generation of bond descriptors.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
The default functor for the generation of bond descriptors.
Definition: PathFingerprintGenerator.hpp:125
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
DefBondDescriptorFunctor(unsigned int flags=DEF_BOND_PROPERTY_FLAGS)
Constructs the bond descriptor functor object for the specified set of bond properties.
Definition: PathFingerprintGenerator.hpp:139
const unsigned int TOPOLOGY
Specifies the ring/chain topology of a bond.
Definition: BondPropertyFlag.hpp:68
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
void setNumBits(std::size_t num_bits)
Allows to specify the desired fingerprint size.
The namespace of the Chemical Data Processing Library.
std::uint64_t operator()(const Chem::Bond &bond) const
Generates a descriptor for the argument bond.
std::function< std::uint64_t(const Chem::Bond &)> BondDescriptorFunction
Type of the generic functor class used to store user-defined functions or function objects for the ge...
Definition: PathFingerprintGenerator.hpp:175
DefAtomDescriptorFunctor(unsigned int flags=DEF_ATOM_PROPERTY_FLAGS)
Constructs the atom descriptor functor object for the specified set of atomic properties.
Definition: PathFingerprintGenerator.hpp:103
const unsigned int AROMATICITY
Specifies the membership of an atom in aromatic rings.
Definition: Chem/AtomPropertyFlag.hpp:93
void generate(const Chem::MolecularGraph &molgraph, Util::BitSet &fp)
Generates the fingerprint of the molecular graph molgraph.
PathFingerprintGenerator.
Definition: PathFingerprintGenerator.hpp:66
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
Definition of the preprocessor macro CDPL_DESCR_API.
#define CDPL_DESCR_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::function< std::uint64_t(const Chem::Atom &)> AtomDescriptorFunction
Type of the generic functor class used to store user-defined functions or function objects for the ge...
Definition: PathFingerprintGenerator.hpp:165
std::uint64_t operator()(const Chem::Atom &atom) const
Generates a descriptor for the argument atom.
std::size_t getMinPathLength() const
Returns the minimum length a path must have to contribute to the generated fingerprint.
PathFingerprintGenerator()
Constructs the PathFingerprintGenerator instance.