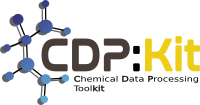 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_FEATURESET_HPP
30 #define CDPL_PHARM_FEATURESET_HPP
33 #include <unordered_map>
36 #include <boost/iterator/indirect_iterator.hpp>
54 typedef std::vector<Feature*> FeatureList;
225 typedef std::unordered_map<const Feature*, std::size_t> FeatureIndexMap;
227 FeatureList features;
228 FeatureIndexMap featureIndices;
233 #endif // CDPL_PHARM_FEATURESET_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
Feature & getFeature(std::size_t idx)
Returns a non-const reference to the feature at index idx.
FeatureIterator removeFeature(const FeatureIterator &it)
Removes the feature specified by the iterator it.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::size_t getNumFeatures() const
Returns the number of features.
void clear()
Removes all features and properties.
FeatureSet()
Constructs an empty FeatureSet instance.
boost::indirect_iterator< FeatureList::iterator, Feature > FeatureIterator
A mutable random access iterator used to iterate over the stored const Pharm::Feature objects.
Definition: FeatureSet.hpp:70
bool containsFeature(const Feature &ftr) const
Tells whether the specified feature is part of this feature set.
boost::indirect_iterator< FeatureList::const_iterator, const Feature > ConstFeatureIterator
A constant random access iterator used to iterate over the stored const Pharm::Feature objects.
Definition: FeatureSet.hpp:65
Definition of the class CDPL::Pharm::FeatureContainer.
FeatureSet(const FeatureSet &ftr_set)
Constructs a copy of the FeatureSet instance ftr_set.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
FeatureSet & operator=(const FeatureContainer &cntnr)
Replaces the current set of features and properties by the features and properties of the feature con...
void removeFeature(std::size_t idx)
Removes the feature at the specified index.
FeatureSet(const FeatureContainer &cntnr)
Constructs a FeatureSet instance storing the features and properties of the feature container cntnr.
FeatureContainer.
Definition: FeatureContainer.hpp:53
FeatureIterator getFeaturesBegin()
Returns a mutable iterator pointing to the beginning of the stored const Pharm::Feature objects.
bool removeFeature(const Feature &ftr)
Removes the specified feature.
FeatureIterator getFeaturesEnd()
Returns a mutable iterator pointing to the end of the stored const Pharm::Feature objects.
const Feature & getFeature(std::size_t idx) const
Returns a const reference to the feature at index idx.
std::shared_ptr< FeatureSet > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated FeatureSet instances.
Definition: FeatureSet.hpp:60
FeatureSet.
Definition: FeatureSet.hpp:52
The namespace of the Chemical Data Processing Library.
FeatureSet & operator-=(const FeatureContainer &cntnr)
Removes the pharmacophore features referenced by the feature container cntnr from this FeatureSet ins...
FeatureSet & operator=(const FeatureSet &ftr_set)
Replaces the current set of features and properties by the features and properties of the feature set...
std::size_t getFeatureIndex(const Feature &ftr) const
Returns the index of the specified feature.
ConstFeatureIterator getFeaturesBegin() const
Returns a constant iterator pointing to the beginning of the stored const Pharm::Feature objects.
FeatureSet & operator+=(const FeatureContainer &cntnr)
Extends the current set of features by the features in the feature container cntnr.
ConstFeatureIterator getFeaturesEnd() const
Returns a constant iterator pointing to the end of the stored const Pharm::Feature objects.
Feature.
Definition: Feature.hpp:48
bool addFeature(const Feature &ftr)
Extends the feature set by the specified feature.