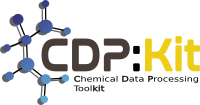 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
31 #ifndef CDPL_DESCR_CIRCULARFINGERPRINTGENERATOR_HPP
32 #define CDPL_DESCR_CIRCULARFINGERPRINTGENERATOR_HPP
40 #include <boost/random/linear_congruential.hpp>
76 static constexpr
unsigned int DEF_ATOM_PROPERTY_FLAGS =
85 static constexpr
unsigned int DEF_BOND_PROPERTY_FLAGS =
279 typedef std::pair<std::pair<std::uint64_t, std::uint64_t>,
const Chem::Atom*> NeighborData;
283 void performIteration(std::size_t iter_num);
285 unsigned int getStereoFlag(
const Chem::Atom& ctr_atom)
const;
289 static bool compareNeighborData(
const NeighborData& nbr1,
const NeighborData& nbr2);
291 typedef std::pair<std::uint64_t, Util::BitSet> Feature;
292 typedef std::vector<Feature> FeatureArray;
293 typedef std::vector<const Feature*> FeaturePtrList;
294 typedef std::vector<std::uint64_t> UInt64Array;
295 typedef std::vector<NeighborData> NeighborDataList;
298 std::size_t numIterations;
303 boost::rand48 randGenerator;
304 UInt64Array bondIdentifiers;
305 FeatureArray features;
306 FeaturePtrList outputFeatures;
307 UInt64Array idCalculationData;
308 NeighborDataList neighborData;
314 #endif // CDPL_DESCR_CIRCULARFINGERPRINTGENERATOR_HPP
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
CircularFingerprintGenerator(const Chem::MolecularGraph &molgraph)
Constructs the CircularFingerprintGenerator instance and generates the atom-centered circular substru...
void setAtomIdentifierFunction(const AtomIdentifierFunction &func)
Allows to specify a customized function for the generation of initial atom identifiers.
Bond.
Definition: Bond.hpp:50
void generate(const Chem::MolecularGraph &molgraph)
Generates the atom-centered circular substructure fingerprint of the molecular graph molgraph.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
std::function< std::uint64_t(const Chem::Bond &)> BondIdentifierFunction
Type of the generic functor class used to store user-defined functions or function objects for the ge...
Definition: CircularFingerprintGenerator.hpp:189
const Util::BitSet & getFeatureSubstructure(std::size_t ftr_idx) const
Atom.
Definition: Atom.hpp:52
Fragment.
Definition: Fragment.hpp:52
void setFeatureBits(Util::BitSet &bs, bool reset=true) const
Maps previously generated feature identifiers to bit indices and sets the correponding bits of bs.
void setBondIdentifierFunction(const BondIdentifierFunction &func)
Allows to specify a customized function for the generation of initial bond identifiers.
CircularFingerprintGenerator()
Constructs the CircularFingerprintGenerator instance.
void setNumIterations(std::size_t num_iter)
Allows to specify the desired number of feature substructure growing iterations.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
DefAtomIdentifierFunctor(unsigned int flags=DEF_ATOM_PROPERTY_FLAGS)
Constructs the atom identifier functor object for the specified set of atomic properties.
Definition: CircularFingerprintGenerator.hpp:113
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
DefBondIdentifierFunctor(unsigned int flags=DEF_BOND_PROPERTY_FLAGS)
Constructs the bond identifier functor object for the specified set of bond properties.
Definition: CircularFingerprintGenerator.hpp:153
const unsigned int VALENCE
Specifies the valence of an atom.
Definition: Chem/AtomPropertyFlag.hpp:113
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
void getFeatureSubstructure(std::size_t ftr_idx, Chem::Fragment &frag, bool clear=true) const
std::size_t getNumIterations() const
Returns the number of feature substructure growing iterations.
std::uint64_t operator()(const Chem::Bond &bond) const
Generates an identifier for the argument bond.
std::function< std::uint64_t(const Chem::Atom &, const Chem::MolecularGraph &)> AtomIdentifierFunction
Type of the generic functor class used to store user-defined functions or function objects for the ge...
Definition: CircularFingerprintGenerator.hpp:179
void includeHydrogens(bool include)
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
void getFeatureSubstructures(std::size_t bit_idx, std::size_t bs_size, Chem::FragmentList &frags, bool clear=true) const
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
void setFeatureBits(std::size_t atom_idx, Util::BitSet &bs, bool reset=true) const
Maps previously generated identifiers of structural features involving the atom specified by atom_idx...
std::uint64_t getFeatureIdentifier(std::size_t ftr_idx) const
const unsigned int TOPOLOGY
Specifies the ring/chain topology of an atom.
Definition: Chem/AtomPropertyFlag.hpp:88
The namespace of the Chemical Data Processing Library.
void includeChirality(bool include)
const unsigned int HEAVY_BOND_COUNT
Specifies the heavy bond count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:108
bool hydrogensIncluded() const
bool chiralityIncluded() const
const unsigned int H_COUNT
Specifies the hydrogen count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:78
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
Definition of the preprocessor macro CDPL_DESCR_API.
CircularFingerprintGenerator.
Definition: CircularFingerprintGenerator.hpp:69
#define CDPL_DESCR_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
The default functor for the generation of bond identifiers.
Definition: CircularFingerprintGenerator.hpp:137
std::uint64_t operator()(const Chem::Atom &atom, const Chem::MolecularGraph &molgraph) const
Generates an identifier for the argument atom.
std::size_t getNumFeatures() const
The functor for the generation of ECFP atom identifiers.
Definition: CircularFingerprintGenerator.hpp:92