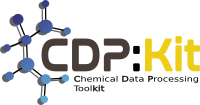 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_BASICPHARMACOPHORE_HPP
30 #define CDPL_PHARM_BASICPHARMACOPHORE_HPP
34 #include <boost/iterator/indirect_iterator.hpp>
55 typedef FeatureCache::SharedObjectPointer FeaturePointer;
56 typedef std::vector<FeaturePointer> FeatureList;
64 typedef boost::indirect_iterator<FeatureList::iterator, BasicFeature>
FeatureIterator;
158 using Pharmacophore::operator=;
171 using Pharmacophore::operator+=;
201 template <
typename T>
202 void doCopy(
const T& pharm);
204 template <
typename T>
205 void doAppend(
const T& pharm);
207 void clearFeatures();
209 void renumberFeatures(std::size_t idx);
217 FeatureList features;
222 #endif // CDPL_PHARM_BASICPHARMACOPHORE_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
FeatureIterator removeFeature(const FeatureIterator &it)
Removes the feature specified by the iterator it.
BasicFeature & getFeature(std::size_t idx)
Returns a non-const reference to the pharmacophore feature at index idx.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the class CDPL::Util::ObjectPool.
BasicFeature.
Definition: BasicFeature.hpp:48
void remove(const FeatureContainer &cntnr)
Removes the pharmacophore features referenced by the feature container cntnr from this Pharmacophore ...
ConstFeatureIterator getFeaturesBegin() const
Returns a constant iterator pointing to the beginning of the features.
BasicPharmacophore(const BasicPharmacophore &pharm)
Constructs a copy of the BasicPharmacophore instance pharm.
FeatureIterator getFeaturesEnd()
Returns a mutable iterator pointing to the end of the features.
const BasicFeature & getFeature(std::size_t idx) const
Returns a const reference to the pharmacophore feature at index idx.
BasicPharmacophore(const Pharmacophore &pharm)
Constructs a copy of the Pharm::Pharmacophore instance pharm.
BasicPharmacophore & operator=(const BasicPharmacophore &pharm)
Replaces the current set of features by a copy of the features and properties of the pharmacophore ph...
FeatureContainer.
Definition: FeatureContainer.hpp:53
void removeFeature(std::size_t idx)
Removes the pharmacophore feature at the specified index.
Pharmacophore::SharedPointer clone() const
Creates a copy of the current pharmacophore state.
void clear()
Removes all features and clears all properties of the pharmacophore.
std::size_t getNumFeatures() const
Returns the number of pharmacophore features.
void append(const FeatureContainer &cntnr)
Extends the current set of pharmacophore features by a copy of the features in the feature container ...
bool containsFeature(const Feature &feature) const
Tells whether the specified feature instance is stored in this pharmacophore.
Pharmacophore.
Definition: Pharmacophore.hpp:48
~BasicPharmacophore()
Destructor.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
std::shared_ptr< BasicPharmacophore > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated BasicPharmacophore instances.
Definition: BasicPharmacophore.hpp:62
BasicPharmacophore.
Definition: BasicPharmacophore.hpp:52
BasicPharmacophore()
Constructs an empty BasicPharmacophore instance.
The namespace of the Chemical Data Processing Library.
BasicFeature & addFeature()
Creates a new pharmacophore feature and adds it to the pharmacophore.
void copy(const Pharmacophore &pharm)
Replaces the current set of pharmacophore features and properties by a copy of the features and prope...
Definition of the class CDPL::Pharm::Pharmacophore.
void copy(const BasicPharmacophore &pharm)
Replaces the current set of features and properties by a copy of the features and properties of the p...
BasicPharmacophore & operator+=(const BasicPharmacophore &pharm)
Extends the current set of features by a copy of the features in the pharmacophore pharm.
std::size_t getFeatureIndex(const Feature &feature) const
Returns the index of the specified feature in this pharmacophore.
Definition of the class CDPL::Pharm::BasicFeature.
ConstFeatureIterator getFeaturesEnd() const
Returns a constant iterator pointing to the end of the features.
std::shared_ptr< Pharmacophore > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Pharmacophore instances.
Definition: Pharmacophore.hpp:54
void copy(const FeatureContainer &cntnr)
Replaces the current set of pharmacophore features and properties by a copy of the features and prope...
void append(const BasicPharmacophore &pharm)
Extends the current set of features by a copy of the features in the pharmacophore pharm.
Feature.
Definition: Feature.hpp:48
void append(const Pharmacophore &pharm)
Extends the current set of pharmacophore features by a copy of the features in the pharmacophore phar...
boost::indirect_iterator< FeatureList::iterator, BasicFeature > FeatureIterator
Definition: BasicPharmacophore.hpp:64
boost::indirect_iterator< FeatureList::const_iterator, const BasicFeature > ConstFeatureIterator
Definition: BasicPharmacophore.hpp:65
FeatureIterator getFeaturesBegin()
Returns a mutable iterator pointing to the beginning of the features.
BasicPharmacophore(const FeatureContainer &cntnr)
Constructs a BasicPharmacophore instance with copies of the features in the Pharm::FeatureContainer i...