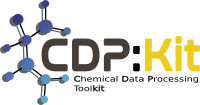 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_PHARMACOPHORE_HPP
30 #define CDPL_PHARM_PHARMACOPHORE_HPP
238 #endif // CDPL_PHARM_PHARMACOPHORE_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
virtual Feature & getFeature(std::size_t idx)=0
Returns a non-const reference to the pharmacophore feature at index idx.
virtual std::size_t getNumFeatures() const =0
Returns the number of pharmacophore features.
virtual bool containsFeature(const Feature &feature) const =0
Tells whether the specified feature instance is stored in this pharmacophore.
FeatureIterator removeFeature(const FeatureIterator &it)
Removes the pharmacophore feature specified by the iterator it.
virtual void copy(const Pharmacophore &pharm)=0
Replaces the current set of pharmacophore features and properties by a copy of the features and prope...
virtual void copy(const FeatureContainer &cntnr)=0
Replaces the current set of pharmacophore features and properties by a copy of the features and prope...
Definition of the class CDPL::Pharm::FeatureContainer.
virtual void remove(const FeatureContainer &cntnr)=0
Removes the pharmacophore features referenced by the feature container cntnr from this Pharmacophore ...
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
virtual Feature & addFeature()=0
Creates a new pharmacophore feature and adds it to the pharmacophore.
FeatureContainer.
Definition: FeatureContainer.hpp:53
FeatureContainer::ConstFeatureIterator ConstFeatureIterator
A constant random access iterator used to iterate over the stored const Pharm::Feature objects.
Definition: Pharmacophore.hpp:59
virtual void clear()=0
Removes all features and clears all properties of the pharmacophore.
std::shared_ptr< FeatureContainer > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated FeatureContainer instances.
Definition: FeatureContainer.hpp:56
virtual void removeFeature(std::size_t idx)=0
Removes the pharmacophore feature at the specified index.
Pharmacophore.
Definition: Pharmacophore.hpp:48
Pharmacophore & operator-=(const FeatureContainer &cntnr)
Removes the pharmacophore features referenced by the feature container cntnr from this Pharmacophore ...
virtual const Feature & getFeature(std::size_t idx) const =0
Returns a const reference to the pharmacophore feature at index idx.
The namespace of the Chemical Data Processing Library.
virtual ~Pharmacophore()
Virtual destructor.
Definition: Pharmacophore.hpp:69
virtual void append(const FeatureContainer &cntnr)=0
Extends the current set of pharmacophore features by a copy of the features in the feature container ...
virtual std::size_t getFeatureIndex(const Feature &feature) const =0
Returns the index of the specified feature in this pharmacophore.
ConstFeatureIterator getFeaturesBegin() const
Returns a constant iterator pointing to the beginning of the stored const Pharm::Feature objects.
ConstFeatureIterator getFeaturesEnd() const
Returns a constant iterator pointing to the end of the stored const Pharm::Feature objects.
virtual void append(const Pharmacophore &pharm)=0
Extends the current set of pharmacophore features by a copy of the features in the pharmacophore phar...
std::shared_ptr< Pharmacophore > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Pharmacophore instances.
Definition: Pharmacophore.hpp:54
FeatureContainer::FeatureIterator FeatureIterator
A mutable random access iterator used to iterate over the stored Pharm::Feature objects.
Definition: Pharmacophore.hpp:64
Feature.
Definition: Feature.hpp:48
virtual SharedPointer clone() const =0
Creates a copy of the current pharmacophore state.