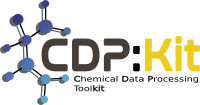 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_SCREENINGPROCESSOR_HPP
30 #define CDPL_PHARM_SCREENINGPROCESSOR_HPP
52 class FeatureContainer;
53 class ScreeningDBAccessor;
54 class ScreeningProcessorImpl;
78 std::size_t mol_idx, std::size_t conf_idx);
102 std::size_t pharmIndex;
103 std::size_t molIndex;
104 std::size_t confIndex;
160 typedef std::unique_ptr<ScreeningProcessorImpl> ImplementationPointer;
166 ImplementationPointer impl;
171 #endif // CDPL_PHARM_SCREENINGPROCESSOR_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
const Chem::Molecule & getHitMolecule() const
std::size_t getHitPharmacophoreIndex() const
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void seekBestAlignments(bool seek_best)
void setProgressCallback(const ProgressCallbackFunction &func)
@ FIRST_MATCHING_CONF
Definition: Pharm/ScreeningProcessor.hpp:66
std::size_t getMaxNumOmittedFeatures() const
const FeatureContainer & getHitPharmacophore() const
const ScoringFunction & getScoringFunction() const
std::size_t searchDB(const FeatureContainer &query, std::size_t mol_start_idx=0, std::size_t mol_end_idx=0)
const Math::Matrix4D & getHitAlignmentTransform() const
std::size_t getHitConformationIndex() const
std::function< bool(const SearchHit &, double)> HitCallbackFunction
Definition: Pharm/ScreeningProcessor.hpp:109
void checkXVolumeClashes(bool check)
std::function< bool(std::size_t, std::size_t)> ProgressCallbackFunction
Definition: Pharm/ScreeningProcessor.hpp:111
const ProgressCallbackFunction & getProgressCallback() const
void setHitCallback(const HitCallbackFunction &func)
std::function< double(const SearchHit &)> ScoringFunction
Definition: Pharm/ScreeningProcessor.hpp:110
FeatureContainer.
Definition: FeatureContainer.hpp:53
Molecule.
Definition: Molecule.hpp:49
HitReportMode
Definition: Pharm/ScreeningProcessor.hpp:64
std::shared_ptr< ScreeningProcessor > SharedPointer
Definition: Pharm/ScreeningProcessor.hpp:107
bool bestAlignmentsSeeked() const
@ BEST_MATCHING_CONF
Definition: Pharm/ScreeningProcessor.hpp:67
ScreeningProcessor.
Definition: Pharm/ScreeningProcessor.hpp:60
ScreeningProcessor(ScreeningDBAccessor &db_acc)
Constructs the ScreeningProcessor instance for the given screening database accessor db_acc.
Definition: Pharm/ScreeningProcessor.hpp:72
void setDBAccessor(ScreeningDBAccessor &db_acc)
void setScoringFunction(const ScoringFunction &func)
SearchHit(const ScreeningProcessor &hit_prov, const FeatureContainer &qry_pharm, const FeatureContainer &hit_pharm, const Chem::Molecule &mol, const Math::Matrix4D &xform, std::size_t pharm_idx, std::size_t mol_idx, std::size_t conf_idx)
The namespace of the Chemical Data Processing Library.
void setHitReportMode(HitReportMode mode)
bool xVolumeClashesChecked() const
const ScreeningProcessor & getHitProvider() const
const HitCallbackFunction & getHitCallback() const
ScreeningDBAccessor & getDBAccessor() const
Definition of matrix data types.
std::size_t getHitMoleculeIndex() const
HitReportMode getHitReportMode() const
A class for accessing the data stored in pharmacophore screening databases.
Definition: ScreeningDBAccessor.hpp:58
void setMaxNumOmittedFeatures(std::size_t max_num)
const FeatureContainer & getQueryPharmacophore() const