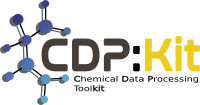 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_FEATUREFUNCTIONS_HPP
30 #define CDPL_PHARM_FEATUREFUNCTIONS_HPP
136 #endif // CDPL_PHARM_FEATUREFUNCTIONS_HPP
CDPL_PHARM_API bool hasOptionalFlag(const Feature &feature)
Definition of the preprocessor macro CDPL_PHARM_API.
CDPL_PHARM_API double getHydrophobicity(const Feature &feature)
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
CDPL_PHARM_API void clearSubstructure(Feature &feature)
CDPL_PHARM_API bool hasDisabledFlag(const Feature &feature)
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
CDPL_PHARM_API void setSubstructure(Feature &feature, const Chem::Fragment::SharedPointer &substruct)
CDPL_PHARM_API void clearOptionalFlag(Feature &feature)
CDPL_PHARM_API bool hasType(const Feature &feature)
CDPL_PHARM_API void clearGeometry(Feature &feature)
CDPL_PHARM_API unsigned int getType(const Feature &feature)
CDPL_PHARM_API const Chem::Fragment::SharedPointer & getSubstructure(const Feature &feature)
CDPL_PHARM_API void clearLength(Feature &feature)
CDPL_PHARM_API void setTolerance(Feature &feature, double tol)
CDPL_PHARM_API double getWeight(const Feature &feature)
CDPL_PHARM_API void setHydrophobicity(Feature &feature, double hyd)
CDPL_PHARM_API bool hasLength(const Feature &feature)
CDPL_PHARM_API void clearHydrophobicity(Feature &feature)
CDPL_PHARM_API bool getDisabledFlag(const Feature &feature)
CDPL_PHARM_API void clearTolerance(Feature &feature)
CDPL_PHARM_API void setOrientation(Feature &feature, const Math::Vector3D &orient)
std::shared_ptr< Fragment > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Fragment instances.
Definition: Fragment.hpp:61
Definition of the class CDPL::Chem::Fragment.
CDPL_PHARM_API bool hasGeometry(const Feature &feature)
The namespace of the Chemical Data Processing Library.
CDPL_PHARM_API void setOptionalFlag(Feature &feature, bool flag)
CDPL_PHARM_API double getTolerance(const Feature &feature)
CDPL_PHARM_API bool hasOrientation(const Feature &feature)
CDPL_PHARM_API bool getOptionalFlag(const Feature &feature)
CDPL_PHARM_API void clearOrientation(Feature &feature)
CDPL_PHARM_API void setLength(Feature &feature, double length)
CDPL_PHARM_API void setDisabledFlag(Feature &feature, bool flag)
CDPL_PHARM_API void clearWeight(Feature &feature)
CDPL_PHARM_API void setWeight(Feature &feature, double weight)
CDPL_PHARM_API void setGeometry(Feature &feature, unsigned int geom)
CDPL_PHARM_API bool hasSubstructure(const Feature &feature)
CDPL_PHARM_API bool hasTolerance(const Feature &feature)
CDPL_PHARM_API unsigned int getGeometry(const Feature &feature)
Feature.
Definition: Feature.hpp:48
CDPL_PHARM_API bool hasWeight(const Feature &feature)
CDPL_PHARM_API double getLength(const Feature &feature)
CDPL_PHARM_API void clearDisabledFlag(Feature &feature)
CDPL_PHARM_API bool hasHydrophobicity(const Feature &feature)
CDPL_PHARM_API void setType(Feature &feature, unsigned int type)
Definition of vector data types.
CDPL_PHARM_API void clearType(Feature &feature)
CDPL_PHARM_API const Math::Vector3D & getOrientation(const Feature &feature)
VectorNorm2< E >::ResultType length(const VectorExpression< E > &e)
Definition: VectorExpression.hpp:553