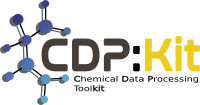 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_PATTERNBASEDFEATUREGENERATOR_HPP
30 #define CDPL_PHARM_PATTERNBASEDFEATUREGENERATOR_HPP
66 FEATURE_ATOM_FLAG = 0x01,
67 POS_REF_ATOM_FLAG = 0x02,
68 GEOM_REF_ATOM1_FLAG = 0x04,
69 GEOM_REF_ATOM2_FLAG = 0x08
97 double tol,
unsigned int geom,
double length = 1.0);
146 struct IncludePattern
150 double tol,
unsigned int geom,
double length):
152 subSearch(new Chem::SubstructureSearch(*substruct)), featureType(type),
153 featureTol(tol), featureGeom(geom), vectorLength(
length)
156 subSearch->uniqueMappingsOnly(
false);
161 unsigned int featureType;
163 unsigned int featureGeom;
167 struct ExcludePattern
171 subQuery(substruct), subSearch(new Chem::SubstructureSearch(*substruct))
174 subSearch->uniqueMappingsOnly(
true);
181 typedef std::vector<IncludePattern> IncludePatternList;
182 typedef std::vector<ExcludePattern> ExcludePatternList;
183 typedef Util::ObjectStack<Util::BitSet> BitSetCache;
184 typedef std::vector<Util::BitSet*> BitSetList;
186 void init(
const Chem::MolecularGraph& molgraph);
188 void getExcludeMatches();
190 void addFeature(
const Chem::AtomBondMapping&,
const IncludePattern&, Pharmacophore&);
192 void createMatchedAtomMask(
const Chem::AtomMapping&,
Util::BitSet&,
bool)
const;
193 bool isContainedInList(
const Util::BitSet&,
const BitSetList&)
const;
195 const Chem::MolecularGraph* molGraph;
196 IncludePatternList includePatterns;
197 ExcludePatternList excludePatterns;
198 BitSetList includeMatches;
199 BitSetList excludeMatches;
200 AtomList posRefAtomList;
201 AtomList geomRefAtom1List;
202 AtomList geomRefAtom2List;
203 Math::Matrix<double> svdU;
206 BitSetCache bitSetCache;
211 #endif // CDPL_PHARM_PATTERNBASEDFEATUREGENERATOR_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
Definition of the preprocessor macro CDPL_PHARM_API.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the class CDPL::Util::ObjectStack.
virtual ~PatternBasedFeatureGenerator()
Virtual destructor.
FeatureGenerator::SharedPointer clone() const
std::shared_ptr< FeatureGenerator > SharedPointer
Definition: FeatureGenerator.hpp:60
std::vector< const Chem::Atom * > AtomList
Definition: PatternBasedFeatureGenerator.hpp:134
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
double calcVecFeatureOrientation(const AtomList &, const AtomList &, Math::Vector3D &) const
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
PatternBasedFeatureGenerator(const PatternBasedFeatureGenerator &gen)
Constructs a copy of the PatternBasedFeatureGenerator instance gen.
PatternBasedFeatureGenerator & operator=(const PatternBasedFeatureGenerator &gen)
Replaces the current set include/exclude patterns by the patterns in the PatternBasedFeatureGenerator...
void generate(const Chem::MolecularGraph &molgraph, Pharmacophore &pharm)
Perceives pharmacophore features according to the specified include/exclude patterns and adds them to...
PatternAtomLabelFlag
Definition: PatternBasedFeatureGenerator.hpp:64
virtual void addNonPatternFeatures(const Chem::MolecularGraph &molgraph, Pharmacophore &pharm)
Definition: PatternBasedFeatureGenerator.hpp:140
FeatureGenerator.
Definition: FeatureGenerator.hpp:57
PatternBasedFeatureGenerator.
Definition: PatternBasedFeatureGenerator.hpp:58
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
void addIncludePattern(const Chem::MolecularGraph::SharedPointer &pattern, unsigned int type, double tol, unsigned int geom, double length=1.0)
Appends a new feature include pattern to the current set of patterns.
void clearExcludePatterns()
Clears the current set of exclude patterns.
std::shared_ptr< PatternBasedFeatureGenerator > SharedPointer
Definition: PatternBasedFeatureGenerator.hpp:61
Definition of the class CDPL::Chem::MolecularGraph.
Pharmacophore.
Definition: Pharmacophore.hpp:48
Definition of the class CDPL::Chem::AtomBondMapping.
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
PatternBasedFeatureGenerator()
Constructs the PatternBasedFeatureGenerator instance.
The namespace of the Chemical Data Processing Library.
bool isContainedInIncMatchList(const Util::BitSet &) const
bool calcPlaneFeatureOrientation(const AtomList &, Math::Vector3D &, Math::Vector3D &)
Definition of the class CDPL::Chem::SubstructureSearch.
Definition of matrix data types.
bool calcCentroid(const AtomList &, Math::Vector3D &) const
void clearIncludePatterns()
Clears the current set of include patterns.
bool isContainedInExMatchList(const Util::BitSet &) const
std::shared_ptr< SubstructureSearch > SharedPointer
Definition: SubstructureSearch.hpp:73
Definition of the class CDPL::Pharm::FeatureGenerator.
void addExcludePattern(const Chem::MolecularGraph::SharedPointer &pattern)
Appends a new feature include pattern to the current set of patterns.
Definition of vector data types.
VectorNorm2< E >::ResultType length(const VectorExpression< E > &e)
Definition: VectorExpression.hpp:553