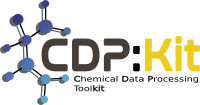 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_PSDMOLECULEREADER_HPP
30 #define CDPL_PHARM_PSDMOLECULEREADER_HPP
88 operator const void*()
const;
93 std::size_t recordIndex;
94 std::size_t numRecords;
100 #endif // CDPL_PHARM_PSDMOLECULEREADER_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setRecordIndex(std::size_t idx)
Sets the index of the current data record to idx.
Definition of the class CDPL::Base::DataReader.
bool hasMoreData()
Tells if there are any data records left to read.
A reader for molecule data in the PSD-format of the CDPL.
Definition: PSDMoleculeReader.hpp:56
Molecule.
Definition: Molecule.hpp:49
PSDMoleculeReader(const std::string &file_name)
Constructs a PSDMoleculeReader instance that will read the molecule data from the input file file_nam...
A class for accessing pharmacophore screening databases in the built-in optimized format.
Definition: PSDScreeningDBAccessor.hpp:50
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
Definition of the class CDPL::Pharm::PSDScreeningDBAccessor.
PSDMoleculeReader & skip()
Skips the data record at the current record index.
std::size_t getRecordIndex() const
The namespace of the Chemical Data Processing Library.
PSDMoleculeReader & read(Chem::Molecule &mol, bool overwrite=true)
Reads the data record at the current record index and stores the read data in obj.
PSDMoleculeReader(std::istream &is)
Constructs a PSDMoleculeReader instance that will read the molecule data from the input stream is.
std::size_t getNumRecords()
Returns the total number of available data records.
PSDMoleculeReader & read(std::size_t idx, Chem::Molecule &mol, bool overwrite=true)
Reads the data record at index idx and stores the read data in obj.