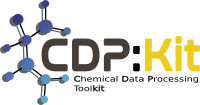 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_ORTHOGONALPIPIINTERACTIONSCORE_HPP
30 #define CDPL_PHARM_ORTHOGONALPIPIINTERACTIONSCORE_HPP
51 static constexpr
double DEF_MAX_V_DISTANCE = 1.4;
52 static constexpr
double DEF_MIN_H_DISTANCE = 4.0;
53 static constexpr
double DEF_MAX_H_DISTANCE = 6.0;
54 static constexpr
double DEF_MAX_ANGLE = 20.0;
72 double max_v_dist = DEF_MAX_V_DISTANCE,
double max_ang = DEF_MAX_ANGLE);
103 #endif // CDPL_PHARM_ORTHOGONALPIPIINTERACTIONSCORE_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
FeatureInteractionScore.
Definition: FeatureInteractionScore.hpp:50
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::function< double(double)> AngleScoringFunction
Definition: OrthogonalPiPiInteractionScore.hpp:62
Definition of the class CDPL::Pharm::FeatureInteractionScore.
std::shared_ptr< OrthogonalPiPiInteractionScore > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated OrthogonalPiPiInteractionScore in...
Definition: OrthogonalPiPiInteractionScore.hpp:59
double getMinHDistance() const
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
std::function< double(double)> DistanceScoringFunction
Definition: OrthogonalPiPiInteractionScore.hpp:61
void setAngleScoringFunction(const AngleScoringFunction &func)
void setDistanceScoringFunction(const DistanceScoringFunction &func)
OrthogonalPiPiInteractionScore(double min_h_dist=DEF_MIN_H_DISTANCE, double max_h_dist=DEF_MAX_H_DISTANCE, double max_v_dist=DEF_MAX_V_DISTANCE, double max_ang=DEF_MAX_ANGLE)
Constructs a OrthogonalPiPiInteractionScore functor with the specified constraints.
double operator()(const Feature &ftr1, const Feature &ftr2) const
double operator()(const Math::Vector3D &ftr1_pos, const Feature &ftr2) const
double getMaxVDistance() const
The namespace of the Chemical Data Processing Library.
double getMaxHDistance() const
OrthogonalPiPiInteractionScore.
Definition: OrthogonalPiPiInteractionScore.hpp:48
double getMaxAngle() const
Feature.
Definition: Feature.hpp:48