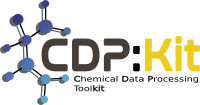 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_INTERACTIONANALYZER_HPP
30 #define CDPL_PHARM_INTERACTIONANALYZER_HPP
46 class FeatureContainer;
118 typedef std::pair<unsigned int, unsigned int> FeatureTypePair;
119 typedef std::map<FeatureTypePair, ConstraintFunction> ConstraintFunctionMap;
121 ConstraintFunctionMap constraintFuncMap;
126 #endif // CDPL_PHARM_INTERACTIONANALYZER_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
Definition of the type CDPL::Pharm::FeatureMapping.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
A data type for the storage and lookup of arbitrary feature to feature mappings.
Definition: FeatureMapping.hpp:54
void removeConstraintFunction(unsigned int type1, unsigned type2)
Removes the constraint check function that was registered for the specified pair of feature types.
const ConstraintFunction & getConstraintFunction(unsigned int type1, unsigned type2) const
Returns the constraint check function that was registered for the specified pair of feature types.
void analyze(const FeatureContainer &cntnr1, const FeatureContainer &cntnr2, FeatureMapping &iactions, bool append=false) const
Analyzes possible interactions of the features in feature container cntnr1 and with features of cntnr...
InteractionAnalyzer.
Definition: InteractionAnalyzer.hpp:53
virtual ~InteractionAnalyzer()
Virtual destructor.
Definition: InteractionAnalyzer.hpp:69
void setConstraintFunction(unsigned int type1, unsigned type2, const ConstraintFunction &func)
Specifies a function that gets used for checking whether all constraints are actually fulfilled for a...
FeatureContainer.
Definition: FeatureContainer.hpp:53
InteractionAnalyzer()
Constructs the InteractionAnalyzer instance.
Definition: InteractionAnalyzer.hpp:64
The namespace of the Chemical Data Processing Library.
Feature.
Definition: Feature.hpp:48
std::function< bool(const Feature &, const Feature &)> ConstraintFunction
A generic wrapper class used to store feature interaction constraint test functions.
Definition: InteractionAnalyzer.hpp:59