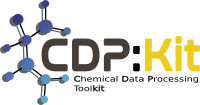 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_HYDROPHOBICFEATUREGENERATOR_HPP
30 #define CDPL_PHARM_HYDROPHOBICFEATUREGENERATOR_HPP
58 static constexpr
double DEF_HYD_THRESHOLD_RING = 2.0;
59 static constexpr
double DEF_HYD_THRESHOLD_CHAIN = 0.8;
60 static constexpr
double DEF_HYD_THRESHOLD_GROUP = 0.8;
62 static constexpr
double DEF_FEATURE_TOL = 1.5;
209 double calcHydWeightedCentroid(
const AtomList&,
Math::Vector3D&)
const;
211 void getAtomHydrophobicities();
213 void createAtomMask(
const AtomList&,
Util::BitSet&)
const;
214 double calcSummedHydrophobicity(
const AtomList& alist)
const;
224 unsigned int featureType;
226 unsigned int featureGeom;
227 double hydThreshRing;
228 double hydThreshChain;
229 double hydThreshGroup;
231 AtomList featureAtoms;
237 #endif // CDPL_PHARM_HYDROPHOBICFEATUREGENERATOR_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
~HydrophobicFeatureGenerator()
const unsigned int SPHERE
Definition: FeatureGeometry.hpp:47
std::shared_ptr< FeatureGenerator > SharedPointer
Definition: FeatureGenerator.hpp:60
void setChainHydrophobicityThreshold(double thresh)
Specifies the minimum summed hydrophobicity of the atoms in chain fragments that is required for the ...
std::shared_ptr< HydrophobicFeatureGenerator > SharedPointer
Definition: HydrophobicFeatureGenerator.hpp:66
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
void setFeatureTolerance(double tol)
Specifies the value of the feature tolerance property that has to be set on newly generated features.
HydrophobicFeatureGenerator & operator=(const HydrophobicFeatureGenerator &gen)
Copies the HydrophobicFeatureGenerator instance gen.
void setFeatureType(unsigned int type)
Specifies the value of the feature type property that has to be set on newly generated features.
HydrophobicFeatureGenerator()
Constructs the HydrophobicFeatureGenerator instance.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Atom.
Definition: Atom.hpp:52
void setGroupHydrophobicityThreshold(double thresh)
Specifies the minimum summed hydrophobicity of the atoms in group fragments that is required for the ...
Fragment.
Definition: Fragment.hpp:52
double getRingHydrophobicityThreshold() const
Returns the minimum summed hydrophobicity of the atoms in small rings that is required for the genera...
void setRingHydrophobicityThreshold(double thresh)
Specifies the minimum summed hydrophobicity of the atoms in small rings that is required for the gene...
PatternBasedFeatureGenerator.
Definition: PatternBasedFeatureGenerator.hpp:58
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition of the class CDPL::Util::Array.
unsigned int getFeatureGeometry() const
Returns the value of the feature geometry property that gets set on newly generated features.
Definition of constants in namespace CDPL::Pharm::FeatureGeometry.
HydrophobicFeatureGenerator(const HydrophobicFeatureGenerator &gen)
Constructs a copy of the HydrophobicFeatureGenerator instance gen.
HydrophobicFeatureGenerator(const Chem::MolecularGraph &molgraph, Pharmacophore &pharm)
Perceives hydrophobic group features of the molecular graph a\ molgraph and adds them to the pharmaco...
std::shared_ptr< Fragment > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Fragment instances.
Definition: Fragment.hpp:61
Pharmacophore.
Definition: Pharmacophore.hpp:48
FeatureGenerator::SharedPointer clone() const
Definition of the class CDPL::Chem::Fragment.
double getGroupHydrophobicityThreshold() const
Returns the minimum summed hydrophobicity of the atoms in group fragments that is required for the ge...
void setFeatureGeometry(unsigned int geom)
Specifies the value of the feature geometry property that has to be set on newly generated features.
The namespace of the Chemical Data Processing Library.
double getFeatureTolerance() const
Returns the value of the feature tolerance property that gets set on newly generated features.
Definition of the class CDPL::Pharm::PatternBasedFeatureGenerator.
unsigned int getFeatureType() const
Returns the value of the feature type property that gets set on newly generated features.
Array< double > DArray
An array of double precision floating-point numbers.
Definition: Array.hpp:587
const unsigned int HYDROPHOBIC
Definition: Pharm/FeatureType.hpp:47
HydrophobicFeatureGenerator.
Definition: HydrophobicFeatureGenerator.hpp:55
Feature.
Definition: Feature.hpp:48
double getChainHydrophobicityThreshold() const
Returns the minimum summed hydrophobicity of the atoms in chain fragments that is required for the ge...
Definition of constants in namespace CDPL::Pharm::FeatureType.
Definition of vector data types.