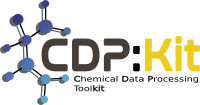 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_HBONDINGINTERACTIONSCORE_HPP
30 #define CDPL_PHARM_HBONDINGINTERACTIONSCORE_HPP
51 static constexpr
double DEF_MIN_HB_LENGTH = 1.2;
52 static constexpr
double DEF_MAX_HB_LENGTH = 2.8;
53 static constexpr
double DEF_MIN_AHD_ANGLE = 150.0;
54 static constexpr
double DEF_MAX_ACC_ANGLE = 75.0;
74 double min_ahd_ang = DEF_MIN_AHD_ANGLE,
double max_acc_ang = DEF_MAX_ACC_ANGLE);
107 #endif // CDPL_PHARM_HBONDINGINTERACTIONSCORE_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
FeatureInteractionScore.
Definition: FeatureInteractionScore.hpp:50
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the class CDPL::Pharm::FeatureInteractionScore.
std::shared_ptr< HBondingInteractionScore > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated HBondingInteractionScore instance...
Definition: HBondingInteractionScore.hpp:59
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
void setAcceptorAngleScoringFunction(const AngleScoringFunction &func)
double getMaxAcceptorAngle() const
std::function< double(double)> DistanceScoringFunction
Definition: HBondingInteractionScore.hpp:61
double operator()(const Feature &ftr1, const Feature &ftr2) const
double operator()(const Math::Vector3D &ftr1_pos, const Feature &ftr2) const
void setDistanceScoringFunction(const DistanceScoringFunction &func)
HBondingInteractionScore(bool don_acc, double min_len=DEF_MIN_HB_LENGTH, double max_len=DEF_MAX_HB_LENGTH, double min_ahd_ang=DEF_MIN_AHD_ANGLE, double max_acc_ang=DEF_MAX_ACC_ANGLE)
Constructs a HBondingInteractionScore functor with the specified constraints.
double getMinLength() const
The namespace of the Chemical Data Processing Library.
double getMaxLength() const
std::function< double(double)> AngleScoringFunction
Definition: HBondingInteractionScore.hpp:62
void setAHDAngleScoringFunction(const AngleScoringFunction &func)
Feature.
Definition: Feature.hpp:48
HBondingInteractionScore.
Definition: HBondingInteractionScore.hpp:48
double getMinAHDAngle() const