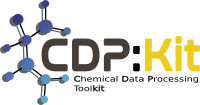 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_PHARM_FEATURECONTAINER_HPP
30 #define CDPL_PHARM_FEATURECONTAINER_HPP
55 class ConstFeatureAccessor;
56 class FeatureAccessor;
184 ConstFeatureAccessor(
const FeatureAccessor& accessor):
185 container(accessor.container) {}
190 const Feature& operator()(std::size_t idx)
const;
192 bool operator==(
const ConstFeatureAccessor& accessor)
const;
194 ConstFeatureAccessor& operator=(
const FeatureAccessor& accessor);
197 const FeatureContainer* container;
203 friend class ConstFeatureAccessor;
206 FeatureAccessor(FeatureContainer* cntnr):
209 Feature& operator()(std::size_t idx)
const;
211 bool operator==(
const FeatureAccessor& accessor)
const;
214 FeatureContainer* container;
220 #endif // CDPL_PHARM_FEATURECONTAINER_HPP
Definition of the preprocessor macro CDPL_PHARM_API.
virtual Feature & getFeature(std::size_t idx)=0
Returns a non-const reference to the pharmacophore feature at index idx.
#define CDPL_PHARM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
ConstFeatureIterator begin() const
Returns a constant iterator pointing to the beginning of the stored const Pharm::Feature objects.
Definition of the class CDPL::Util::IndexedElementIterator.
virtual ~FeatureContainer()
Virtual destructor.
Definition: FeatureContainer.hpp:77
virtual Chem::Entity3D & getEntity(std::size_t idx)
Returns a non-const reference to the entity at index idx.
GridEquality< E1, E2 >::ResultType operator==(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:339
Util::IndexedElementIterator< const Feature, ConstFeatureAccessor > ConstFeatureIterator
A constant random access iterator used to iterate over the stored const Pharm::Feature objects.
Definition: FeatureContainer.hpp:67
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
A common interface for data-structures that support a random access to stored Chem::Entity3D instance...
Definition: Entity3DContainer.hpp:53
FeatureContainer.
Definition: FeatureContainer.hpp:53
ConstFeatureIterator end() const
Returns a constant iterator pointing to the end of the stored const Pharm::Feature objects.
virtual std::size_t getFeatureIndex(const Feature &feature) const =0
Returns the index of the specified feature.
Definition of the class CDPL::Chem::Entity3DContainer.
std::shared_ptr< FeatureContainer > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated FeatureContainer instances.
Definition: FeatureContainer.hpp:56
A class providing methods for the storage and lookup of object properties.
Definition: PropertyContainer.hpp:75
virtual const Feature & getFeature(std::size_t idx) const =0
Returns a const reference to the feature at index idx.
The namespace of the Chemical Data Processing Library.
virtual bool containsFeature(const Feature &feature) const =0
Tells whether the specified feature instance is stored in this pharmacophore.
virtual std::size_t getNumFeatures() const =0
Returns the number of contained features.
Util::IndexedElementIterator< Feature, FeatureAccessor > FeatureIterator
A mutable random access iterator used to iterate over the stored Pharm::Feature objects.
Definition: FeatureContainer.hpp:72
FeatureIterator begin()
Returns a mutable iterator pointing to the beginning of the stored Pharm::Feature objects.
virtual std::size_t getNumEntities() const
Returns the number of stored Chem::Entity3D objects.
FeatureIterator end()
Returns a mutable iterator pointing to the end of the stored Pharm::Feature objects.
virtual const Chem::Entity3D & getEntity(std::size_t idx) const
Returns a const reference to the Chem::Entity3D instance at index idx.
Feature.
Definition: Feature.hpp:48
Entity3D.
Definition: Entity3D.hpp:46
Definition of the class CDPL::Base::PropertyContainer.
FeatureContainer & operator=(const FeatureContainer &cntnr)
Replaces the current set of pharmacophore features and properties by a copy of the features and prope...