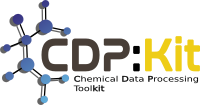 |
Chemical Data Processing Library C++ API - Version 1.0.0
|
AutomorphismGroupSearch.
More...
#include <AutomorphismGroupSearch.hpp>
◆ SharedPointer
◆ MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
◆ ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
◆ MappingCallbackFunction
◆ AutomorphismGroupSearch()
Constructs and initializes a AutomorphismGroupSearch
instance.
◆ setAtomPropertyFlags()
void CDPL::Chem::AutomorphismGroupSearch::setAtomPropertyFlags |
( |
unsigned int |
flags | ) |
|
◆ getAtomPropertyFlags()
unsigned int CDPL::Chem::AutomorphismGroupSearch::getAtomPropertyFlags |
( |
| ) |
const |
◆ setBondPropertyFlags()
void CDPL::Chem::AutomorphismGroupSearch::setBondPropertyFlags |
( |
unsigned int |
flags | ) |
|
◆ getBondPropertyFlags()
unsigned int CDPL::Chem::AutomorphismGroupSearch::getBondPropertyFlags |
( |
| ) |
const |
◆ includeIdentityMapping()
void CDPL::Chem::AutomorphismGroupSearch::includeIdentityMapping |
( |
bool |
include | ) |
|
◆ identityMappingIncluded()
bool CDPL::Chem::AutomorphismGroupSearch::identityMappingIncluded |
( |
| ) |
const |
◆ findMappings()
Searches for the possible atom/bond mappings in the automorphism group of the given molecular graph.
The method will store all found mappings up to the maximum number of recorded mappings specified by setMaxNumMappings().
- Parameters
-
molgraph | The molecular graph that has to be searched for automorphisms. |
- Returns
true
if any mappings of the specified molecular graph have been found, and false
otherwise.
- Note
- Any atom/bond mappings that were recorded in a previous call to findMappings() will be discarded.
◆ stopSearch()
void CDPL::Chem::AutomorphismGroupSearch::stopSearch |
( |
| ) |
|
◆ getNumMappings()
std::size_t CDPL::Chem::AutomorphismGroupSearch::getNumMappings |
( |
| ) |
const |
Returns the number of atom/bond mappings that were recorded in the last call to findMappings().
- Returns
- The number of atom/bond mappings that were recorded in the last call to findMappings().
◆ getMapping() [1/2]
AtomBondMapping& CDPL::Chem::AutomorphismGroupSearch::getMapping |
( |
std::size_t |
idx | ) |
|
Returns a non-const
reference to the stored atom/bond mapping object at index idx.
- Parameters
-
idx | The zero-based index of the atom/bond mapping object to return. |
- Returns
- A non-
const
reference to the atom/bond mapping object at index idx.
- Exceptions
-
◆ getMapping() [2/2]
const AtomBondMapping& CDPL::Chem::AutomorphismGroupSearch::getMapping |
( |
std::size_t |
idx | ) |
const |
Returns a const
reference to the stored atom/bond mapping object at index idx.
- Parameters
-
idx | The zero-based index of the atom/bond mapping object to return. |
- Returns
- A
const
reference to the atom/bond mapping object at index idx.
- Exceptions
-
◆ getMappingsBegin() [1/2]
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
- Returns
- A mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
◆ getMappingsBegin() [2/2]
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
- Returns
- A constant iterator pointing to the beginning of the stored atom/bond mapping objects.
◆ getMappingsEnd() [1/2]
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
- Returns
- A mutable iterator pointing to the end of the stored atom/bond mapping objects.
◆ getMappingsEnd() [2/2]
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
- Returns
- A constant iterator pointing to the end of the stored atom/bond mapping objects.
◆ begin() [1/2]
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
- Returns
- A mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
◆ begin() [2/2]
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
- Returns
- A constant iterator pointing to the beginning of the stored atom/bond mapping objects.
◆ end() [1/2]
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
- Returns
- A mutable iterator pointing to the end of the stored atom/bond mapping objects.
◆ end() [2/2]
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
- Returns
- A constant iterator pointing to the end of the stored atom/bond mapping objects.
◆ setMaxNumMappings()
void CDPL::Chem::AutomorphismGroupSearch::setMaxNumMappings |
( |
std::size_t |
max_num_mappings | ) |
|
Allows to specify a limit on the number of stored atom/bond mappings.
In a call to findMappings() the automorphism search will terminate as soon as the specified maximum number of stored atom/bond mappings has been reached. A previously set limit on the number of mappings can be disabled by providing zero for the value of max_num_mappings.
- Parameters
-
max_num_mappings | The maximum number of atom/bond mappings to store. |
- Note
- By default, no limit is imposed on the number of stored mappings.
◆ getMaxNumMappings()
std::size_t CDPL::Chem::AutomorphismGroupSearch::getMaxNumMappings |
( |
| ) |
const |
Returns the specified limit on the number of stored atom/bond mappings.
- Returns
- The specified maximum number of stored atom/bond mappings.
- See also
- setMaxNumMappings(), findMappings()
◆ setFoundMappingCallback()
◆ getFoundMappingCallback()
◆ DEF_ATOM_PROPERTY_FLAGS
constexpr unsigned int CDPL::Chem::AutomorphismGroupSearch::DEF_ATOM_PROPERTY_FLAGS |
|
staticconstexpr |
Initial value:
Specifies the default set of atomic properties considered for atom matching.
◆ DEF_BOND_PROPERTY_FLAGS
constexpr unsigned int CDPL::Chem::AutomorphismGroupSearch::DEF_BOND_PROPERTY_FLAGS |
|
staticconstexpr |
Initial value:
Specifies the default set of bond properties considered for bond matching.
The documentation for this class was generated from the following file:
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
const unsigned int CONFIGURATION
Specifies the steric configuration of a double bond.
Definition: BondPropertyFlag.hpp:78
const unsigned int EXPLICIT_BOND_COUNT
Specifies the explicit bond count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:118
const unsigned int CONFIGURATION
Specifies the configuration of a stereogenic atom.
Definition: Chem/AtomPropertyFlag.hpp:98
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
const unsigned int TOPOLOGY
Specifies the ring/chain topology of a bond.
Definition: BondPropertyFlag.hpp:68
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
const unsigned int AROMATICITY
Specifies the membership of an atom in aromatic rings.
Definition: Chem/AtomPropertyFlag.hpp:93
const unsigned int H_COUNT
Specifies the hydrogen count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:78
const unsigned int HYBRIDIZATION_STATE
Specifies the hybridization state an atom.
Definition: Chem/AtomPropertyFlag.hpp:123