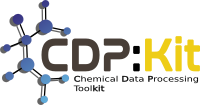 |
Chemical Data Processing Library C++ API - Version 1.0.0
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_TAUTOMERGENERATOR_HPP
30 #define CDPL_CHEM_TAUTOMERGENERATOR_HPP
35 #include <unordered_set>
118 typedef MoleculeCache::SharedObjectPointer MoleculePtr;
121 void initHashCalculator();
129 bool addNewTautomer(
const MoleculePtr& mol);
131 std::uint64_t calcTautomerHashCode(
const BasicMolecule& tautomer);
133 typedef std::array<std::size_t, 3> BondDescriptor;
134 typedef std::vector<MoleculePtr> MoleculeList;
135 typedef std::vector<TautomerizationRule::SharedPointer> TautRuleList;
136 typedef std::vector<BondDescriptor> BondDescrArray;
137 typedef std::vector<std::size_t> SizeTArray;
138 typedef std::unordered_set<std::uint64_t> HashCodeSet;
139 typedef std::array<std::size_t, 6> StereoCenter;
140 typedef std::vector<StereoCenter> StereoCenterList;
148 TautRuleList tautRules;
149 MoleculeList currGeneration;
150 MoleculeList nextGeneration;
151 StereoCenterList atomStereoCenters;
152 StereoCenterList bondStereoCenters;
153 HashCodeSet tautHashCodes;
155 BondDescrArray tautomerBonds;
161 #endif // CDPL_CHEM_TAUTOMERGENERATOR_HPP
std::shared_ptr< TautomerizationRule > SharedPointer
Definition: TautomerizationRule.hpp:53
Definition of the class CDPL::Util::ObjectPool.
void regardStereochemistry(bool regard)
Definition of the class CDPL::Chem::TautomerizationRule.
Definition of the preprocessor macro CDPL_CHEM_API.
const CallbackFunction & getCallbackFunction() const
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
TautomerGenerator.
Definition: TautomerGenerator.hpp:57
Mode
Definition: TautomerGenerator.hpp:61
bool isotopesRegarded() const
Definition of the class CDPL::Chem::HashCodeCalculator.
BasicMolecule.
Definition: BasicMolecule.hpp:54
void addTautomerizationRule(const TautomerizationRule::SharedPointer &rule)
TautomerGenerator & operator=(const TautomerGenerator &gen)
TautomerGenerator()
Constructs the TautomerGenerator instance.
TautomerGenerator(const TautomerGenerator &gen)
std::size_t getNumTautomerizationRules() const
MolecularGraph.
Definition: MolecularGraph.hpp:52
std::function< bool(MolecularGraph &)> CallbackFunction
Definition: TautomerGenerator.hpp:70
void removeTautomerizationRule(std::size_t idx)
bool stereochemistryRegarded() const
@ TOPOLOGICALLY_UNIQUE
Definition: TautomerGenerator.hpp:63
std::shared_ptr< TautomerGenerator > SharedPointer
Definition: TautomerGenerator.hpp:68
const TautomerizationRule::SharedPointer & getTautomerizationRule(std::size_t idx) const
@ GEOMETRICALLY_UNIQUE
Definition: TautomerGenerator.hpp:64
The namespace of the Chemical Data Processing Library.
std::function< void(MolecularGraph &)> CustomSetupFunction
Definition: TautomerGenerator.hpp:71
void setCallbackFunction(const CallbackFunction &func)
void generate(const MolecularGraph &molgraph)
Generates all unique tautomers of the molecular graph molgraph.
HashCodeCalculator.
Definition: HashCodeCalculator.hpp:57
void setCustomSetupFunction(const CustomSetupFunction &func)
virtual ~TautomerGenerator()
Definition: TautomerGenerator.hpp:80
Definition of the class CDPL::Chem::BasicMolecule.
void regardIsotopes(bool regard)