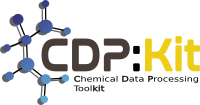 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_STRUCTUREVIEW2D_HPP
30 #define CDPL_VIS_STRUCTUREVIEW2D_HPP
62 class PropertyContainer;
78 class StructureView2DParameters;
547 class CustomGraphicsData;
550 typedef std::shared_ptr<CustomGraphicsData> CustomGraphicsDataPtr;
551 typedef std::vector<CustomGraphicsDataPtr> CustomGraphicsDataList;
560 void renderGraphicsPrimitives(
Renderer2D&)
const;
564 void initTextLabelBounds();
566 void createAtomPrimitives();
576 void createAtomConfigLabelPrimitive(
const Chem::Atom&,
char);
578 void createBondPrimitives();
581 double createBondRxnInfoLabelPrimitive(
const Chem::Bond&,
const Line2D&,
int);
582 void createBondQueryInfoLabelPrimitive(
const Chem::Bond&,
const Line2D&,
int,
double);
590 void createDownSingleBondPrimitives(
const Chem::Bond&,
const Line2D&,
bool);
591 void createEitherSingleBondPrimitives(
const Chem::Bond&,
const Line2D&,
bool);
595 void createAsymDoubleBondPrimitives(
const Chem::Bond&,
bool,
const Line2D&,
int);
602 int getBondAsymmetryShiftDirection(
const Chem::Bond&)
const;
604 bool trimLine(
Line2D&,
double,
bool)
const;
607 bool clipLineAgainstAtomBounds(
Line2D&, std::size_t,
bool)
const;
613 std::size_t getHydrogenCount(
const Chem::Atom&)
const;
618 double calcInputBondLength(
const Chem::Bond&)
const;
620 void prepareStructureData();
621 void initInputAtomPosTable();
623 void calcViewTransforms();
625 double calcAvgInputBondLength()
const;
627 void calcStdBondLengthScalingFactor();
628 void calcViewportAdjustmentScalingFactor();
630 void calcInputStructureBounds();
631 void calcOutputStructureBounds();
632 void calcOutputAtomCoords();
634 void initOutputBondLineTable();
637 std::size_t getBondOrder(
const Chem::Bond& bond)
const;
639 void setHasAtomCoordsFlag();
642 double getRxnCenterLineLength(
const Chem::Bond&)
const;
643 double getRxnCenterLineSpacing(
const Chem::Bond&)
const;
647 double getWedgeWidth(
const Chem::Bond&)
const;
648 double getHashSpacing(
const Chem::Bond&)
const;
650 double getConfigLabelSize(
const Chem::Bond&)
const;
666 double getConfigLabelSize(
const Chem::Atom&)
const;
667 double getElectronDotSize(
const Chem::Atom&)
const;
682 void setupSecondaryLabelFont(
const Chem::Atom&);
691 void freeGraphicsPrimitives();
700 typedef std::vector<const GraphicsPrimitive2D*> GraphicsPrimitiveList;
701 typedef std::vector<Rectangle2D> RectangleList;
702 typedef std::vector<RectangleList> RectangleListTable;
703 typedef std::vector<Line2D> BondLineTable;
711 typedef std::unique_ptr<StructureView2DParameters> StructureView2DParametersPtr;
712 typedef std::unique_ptr<Chem::Fragment> FragmentPtr;
714 StructureView2DParametersPtr parameters;
717 FragmentPtr hDepleteStructure;
725 BondLineTable outputBondLines;
726 RectangleListTable atomLabelBounds;
727 RectangleList bondLabelBounds;
728 GraphicsPrimitiveList drawList;
729 double avgInputBondLength;
730 double stdBondLengthScalingFactor;
731 double viewportAdjustmentScalingFactor;
732 double viewScalingFactor;
734 bool structureChanged;
735 bool fontMetricsChanged;
737 Font activeLabelFont;
738 Font activeSecondaryLabelFont;
739 Font activeConfigLabelFont;
740 double activeLabelMargin;
741 bool reactionContext;
753 #endif // CDPL_VIS_STRUCTUREVIEW2D_HPP
Specifies the value and type of a size attribute and defines how the value may change during processi...
Definition: SizeSpecification.hpp:45
An interface that provides methods for low level 2D drawing operations.
Definition: Renderer2D.hpp:86
Definition of the class CDPL::Util::ObjectStack.
CDPL_VIS_API const Font & getSecondaryLabelFont(const Chem::Atom &atom)
Definition of the class CDPL::Vis::SizeSpecification.
Definition of the class CDPL::Math::VectorArray.
The abstract base of classes implementing the 2D visualization of data objects.
Definition: View2D.hpp:59
~StructureView2D()
Destructor.
Definition of the class CDPL::Vis::Font.
Implements the 2D visualization of chemical reactions.
Definition: ReactionView2D.hpp:590
Definition of the class CDPL::Vis::LineSegmentListPrimitive2D.
CDPL_VIS_API const Font & getLabelFont(const Chem::Atom &atom)
Bond.
Definition: Bond.hpp:50
FontMetrics * getFontMetrics() const
Returns a pointer to the used font metrics object.
CDPL_VIS_API const SizeSpecification & getSecondaryLabelSize(const Chem::Atom &atom)
CDPL_VIS_API const SizeSpecification & getDoubleBondTrimLength(const Chem::Bond &bond)
Specifies how to draw lines and outlines of shapes.
Definition: Pen.hpp:53
Definition of the class CDPL::Vis::PointListPrimitive2D.
A graphics primitive representing a single line.
Definition: LinePrimitive2D.hpp:51
CDPL_VIS_API const Color & getColor(const Chem::Atom &atom)
Atom.
Definition: Atom.hpp:52
A graphics primitive representing a list of disjoint line segments.
Definition: LineSegmentListPrimitive2D.hpp:51
Definition of the class CDPL::Vis::PolygonPrimitive2D.
StructureView2D(const Chem::MolecularGraph *molgraph=0)
Constructs and initializes a StructureView2D instance for the visualization of the chemical structure...
Specifies a font for drawing text.
Definition: Font.hpp:54
Implements the 2D visualization of chemical structures.
Definition: StructureView2D.hpp:493
Definition of the class CDPL::Vis::Pen.
void setFontMetrics(FontMetrics *font_metrics)
Specifies a font metrics object that will be used to measure the dimension of text labels.
MolecularGraph.
Definition: MolecularGraph.hpp:52
std::shared_ptr< StructureView2D > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated StructureView2D instances.
Definition: StructureView2D.hpp:499
CDPL_VIS_API const SizeSpecification & getLabelSize(const Chem::Atom &atom)
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition of the class CDPL::Vis::PolylinePrimitive2D.
CDPL_VIS_API const SizeSpecification & getTripleBondTrimLength(const Chem::Bond &bond)
Definition of the class CDPL::Vis::LinePrimitive2D.
Definition of the class CDPL::Vis::View2D.
A graphics primitive representing a text label.
Definition: TextLabelPrimitive2D.hpp:51
void setStructure(const Chem::MolecularGraph *molgraph)
Specifies the chemical structure to visualize.
const Chem::MolecularGraph * getStructure() const
Returns a pointer to the visualized chemical structure.
A class providing methods for the storage and lookup of object properties.
Definition: PropertyContainer.hpp:75
ValueType calcBondAngle(const CoordsVec &term_atom1_pos, const CoordsVec &ctr_atom_pos, const CoordsVec &term_atom2_pos)
Calculates the bond angle between the two bonds i-j and j-k.
A graphics primitive representing a polygon.
Definition: PolygonPrimitive2D.hpp:52
The namespace of the Chemical Data Processing Library.
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
void getModelBounds(Rectangle2D &bounds)
Calculates the bounds of the rendered structure in output space.
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
Definition of the class CDPL::Vis::Rectangle2D.
Definition of the class CDPL::Vis::Line2D.
void render(Renderer2D &renderer)
Renders the visual representation of the model using the specified Vis::Renderer2D instance.
CDPL_VIS_API const SizeSpecification & getLineSpacing(const Chem::Bond &bond)
An interface class with methods that provide information about the metrics of a font.
Definition: FontMetrics.hpp:71
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
Array< unsigned int > UIArray
An array of unsigned integers.
Definition: Array.hpp:562
Definition of the class CDPL::Vis::Color.
Definition of the preprocessor macro CDPL_VIS_API.
CDPL_VIS_API const SizeSpecification & getLineWidth(const Chem::Bond &bond)
A graphics primitive representing a list of points.
Definition: PointListPrimitive2D.hpp:51
Specifies a color in terms of its red, green and blue components and an alpha-channel for transparenc...
Definition: Color.hpp:52
Specifies a line segment in 2D space.
Definition: Line2D.hpp:48
Definition of the class CDPL::Vis::TextLabelPrimitive2D.
A graphics primitive representing a set of connected line segments.
Definition: PolylinePrimitive2D.hpp:51