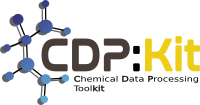 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_STEREOISOMERGENERATOR_HPP
30 #define CDPL_CHEM_STEREOISOMERGENERATOR_HPP
115 typedef std::pair<bool, std::size_t> StereoCenterID;
118 bool isExcluded(
const Bond& bond,
const MolecularGraph& molgraph,
bool has_config)
const;
124 void flipConfiguration(
const StereoCenterID& ctr_id);
126 typedef std::vector<StereoCenterID> StereoCenterIDList;
127 typedef std::vector<std::size_t> IndexList;
131 bool enumAtomConfig{
true};
132 bool enumBondConfig{
true};
133 bool incSpecifiedCtrs{
false};
134 bool incSymmetricCtrs{
false};
135 bool incBridgeheads{
false};
136 bool incInvNitrogens{
false};
137 bool incRingBonds{
false};
138 std::size_t minRingSize{8};
139 StereoDescriptorArray atomDescrs;
140 StereoDescriptorArray bondDescrs;
141 StereoCenterIDList procCtrs;
144 IndexList atomRingSet;
149 #endif // CDPL_CHEM_STEREOISOMERGENERATOR_HPP
void includeBridgeheadAtoms(bool include)
Definition of the preprocessor macro CDPL_CHEM_API.
void setAtomPredicate(const AtomPredicate &pred)
const AtomPredicate & getAtomPredicate() const
void setBondPredicate(const BondPredicate &pred)
void enumerateBondConfig(bool enumerate)
Util::Array< StereoDescriptor > StereoDescriptorArray
Definition: StereoisomerGenerator.hpp:64
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
std::function< bool(const Chem::Bond &)> BondPredicate
A generic wrapper class used to store a user-defined bond predicate.
Definition: BondPredicate.hpp:41
void includeSymmetricCenters(bool include)
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
bool bridgeheadAtomsIncluded() const
std::shared_ptr< StereoisomerGenerator > SharedPointer
Definition: StereoisomerGenerator.hpp:62
Atom.
Definition: Atom.hpp:52
void enumerateAtomConfig(bool enumerate)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
Definition of the class CDPL::Util::Array.
bool atomConfigEnumerated() const
std::function< bool(const Chem::Atom &)> AtomPredicate
A generic wrapper class used to store a user-defined atom predicate.
Definition: AtomPredicate.hpp:41
Type definition of a generic wrapper class for storing user-defined Chem::Bond predicates.
void setup(const MolecularGraph &molgraph)
bool bondConfigEnumerated() const
bool specifiedCentersIncluded() const
void includeRingBonds(bool include)
Type definition of a generic wrapper class for storing user-defined Chem::Atom predicates.
bool invertibleNitrogensIncluded() const
bool ringBondsIncluded() const
const StereoDescriptorArray & getBondDescriptors()
bool symmetricCentersIncluded() const
void setMinRingSize(std::size_t min_size)
const StereoDescriptorArray & getAtomDescriptors()
The namespace of the Chemical Data Processing Library.
void includeSpecifiedCenters(bool include)
void includeInvertibleNitrogens(bool include)
std::size_t getMinRingSize() const
A common interface for data-structures that support a random access to stored Chem::Bond instances.
Definition: BondContainer.hpp:54
Definition of the type CDPL::Chem::StereoDescriptor.
StereoisomerGenerator.
Definition: StereoisomerGenerator.hpp:59
const BondPredicate & getBondPredicate() const