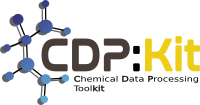 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_MAXCOMMONATOMSUBSTRUCTURESEARCH_HPP
30 #define CDPL_CHEM_MAXCOMMONATOMSUBSTRUCTURESEARCH_HPP
37 #include <boost/iterator/indirect_iterator.hpp>
63 typedef std::vector<AtomBondMapping*> ABMappingList;
71 typedef boost::indirect_iterator<ABMappingList::iterator, AtomBondMapping>
MappingIterator;
76 typedef boost::indirect_iterator<ABMappingList::const_iterator, const AtomBondMapping>
ConstMappingIterator;
288 void initMatchExpressions();
290 bool buildAssocGraph();
292 bool findAssocGraphCliques(std::size_t);
293 bool isLegal(
const AGNode*);
297 bool hasPostMappingMatchExprs()
const;
300 bool foundMappingUnique();
302 void clearMappings();
304 void freeAtomBondMapping();
305 void freeAtomBondMappings();
306 void freeAssocGraph();
312 AGNode* allocAGNode(
const Atom*,
const Atom*);
313 AGEdge* allocAGEdge(
const Bond*,
const Bond*);
315 typedef std::vector<const AGEdge*> AGraphEdgeList;
321 void setQueryAtom(
const Atom*);
322 const Atom* getQueryAtom()
const;
324 void setAssocAtom(
const Atom*);
325 const Atom* getAssocAtom()
const;
327 void addEdge(
const AGEdge*);
329 bool isConnected(
const AGNode*)
const;
330 const AGEdge* findEdge(
const AGNode*)
const;
334 void setIndex(std::size_t idx);
338 const Atom* queryAtom;
339 const Atom* assocAtom;
341 AGraphEdgeList bondEdges;
348 void setQueryBond(
const Bond*);
349 const Bond* getQueryBond()
const;
351 void setAssocBond(
const Bond*);
352 const Bond* getAssocBond()
const;
354 void setNode1(
const AGNode*);
355 void setNode2(
const AGNode*);
357 const AGNode* getNode1()
const;
358 const AGNode* getNode2()
const;
360 const AGNode* getOther(
const AGNode*)
const;
363 const Bond* queryBond;
364 const Bond* assocBond;
373 void initQueryAtomMask(std::size_t);
374 void initTargetAtomMask(std::size_t);
376 void initQueryBondMask(std::size_t);
377 void initTargetBondMask(std::size_t);
379 void setQueryAtomBit(std::size_t);
380 void setTargetAtomBit(std::size_t);
382 void setQueryBondBit(std::size_t);
383 void setTargetBondBit(std::size_t);
387 bool operator<(
const ABMappingMask&)
const;
388 bool operator>(
const ABMappingMask&)
const;
399 typedef std::vector<AGNode*> AGraphNodeList;
400 typedef std::vector<AGraphNodeList> AGraphNodeMatrix;
401 typedef std::set<ABMappingMask> UniqueMappingList;
402 typedef std::vector<const Atom*> AtomList;
403 typedef std::vector<const Bond*> BondList;
404 typedef std::vector<MatchExpression<Atom, MolecularGraph>::SharedPointer> AtomMatchExprTable;
405 typedef std::vector<MatchExpression<Bond, MolecularGraph>::SharedPointer> BondMatchExprTable;
406 typedef Util::ObjectStack<AGNode> NodeCache;
407 typedef Util::ObjectStack<AGEdge> EdgeCache;
408 typedef Util::ObjectStack<AtomBondMapping> MappingCache;
410 const MolecularGraph* query;
411 const MolecularGraph* target;
412 AGraphNodeMatrix nodeMatrix;
413 ABMappingList foundMappings;
414 UniqueMappingList uniqueMappings;
415 AGraphEdgeList cliqueEdges;
416 AGraphNodeList cliqueNodes;
417 ABMappingMask mappingMask;
418 AtomMatchExprTable atomMatchExprTable;
419 BondMatchExprTable bondMatchExprTable;
420 MolGraphMatchExprPtr molGraphMatchExpr;
421 AtomList postMappingMatchAtoms;
422 BondList postMappingMatchBonds;
425 MappingCache mappingCache;
430 bool maxBondMappingsOnly;
431 std::size_t numQueryAtoms;
432 std::size_t numQueryBonds;
433 std::size_t numTargetAtoms;
434 std::size_t numTargetBonds;
435 std::size_t maxAtomSubstructureSize;
436 std::size_t maxBondSubstructureSize;
437 std::size_t currNumNullNodes;
438 std::size_t minNumNullNodes;
439 std::size_t maxNumMappings;
440 std::size_t minSubstructureSize;
441 std::size_t currNodeIdx;
446 #endif // CDPL_CHEM_MAXCOMMONATOMSUBSTRUCTURESEARCH_HPP
MaxCommonAtomSubstructureSearch.
Definition: MaxCommonAtomSubstructureSearch.hpp:61
boost::indirect_iterator< ABMappingList::iterator, AtomBondMapping > MappingIterator
A mutable random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: MaxCommonAtomSubstructureSearch.hpp:71
Definition of the class CDPL::Util::ObjectStack.
MappingIterator getMappingsBegin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
Definition of the preprocessor macro CDPL_CHEM_API.
ConstMappingIterator end() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
std::size_t getMaxNumMappings() const
Returns the specified limit on the number of stored atom/bond mappings.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
MappingIterator getMappingsEnd()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
Bond.
Definition: Bond.hpp:50
MappingIterator begin()
Returns a mutable iterator pointing to the beginning of the stored atom/bond mapping objects.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
std::shared_ptr< MaxCommonAtomSubstructureSearch > SharedPointer
Definition: MaxCommonAtomSubstructureSearch.hpp:66
Atom.
Definition: Atom.hpp:52
bool mappingExists(const MolecularGraph &target)
Searches for a common substructure between the query and the specified target molecular graph.
ConstMappingIterator getMappingsEnd() const
Returns a constant iterator pointing to the end of the stored atom/bond mapping objects.
void setMinSubstructureSize(std::size_t min_size)
Allows to specify the minimum accepted common substructure size.
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
ConstMappingIterator begin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
boost::indirect_iterator< ABMappingList::const_iterator, const AtomBondMapping > ConstMappingIterator
A constant random access iterator used to iterate over the stored atom/bond mapping objects.
Definition: MaxCommonAtomSubstructureSearch.hpp:76
std::size_t getNumMappings() const
Returns the number of atom/bond mappings that were recorded in the last search for common substructur...
bool operator<(const Array< ValueType > &array1, const Array< ValueType > &array2)
Less than comparison operator.
bool uniqueMappingsOnly() const
Tells whether duplicate atom/bond mappings are discarded.
std::shared_ptr< MatchExpression > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MatchExpression instances.
Definition: MatchExpression.hpp:81
void setMaxNumMappings(std::size_t max_num_mappings)
Allows to specify a limit on the number of stored atom/bond mappings.
MaxCommonAtomSubstructureSearch(const MolecularGraph &query)
Constructs and initializes a MaxCommonAtomSubstructureSearch instance for the specified query structu...
Definition of the class CDPL::Chem::AtomBondMapping.
bool findMaxBondMappings(const MolecularGraph &target)
Searches for all atom/bond mappings of query subgraphs to substructures of the specified target molec...
MappingIterator end()
Returns a mutable iterator pointing to the end of the stored atom/bond mapping objects.
MaxCommonAtomSubstructureSearch()
Constructs and initializes a MaxCommonAtomSubstructureSearch instance.
Definition of the class CDPL::Chem::MatchExpression.
The namespace of the Chemical Data Processing Library.
void setQuery(const MolecularGraph &query)
Allows to specify a new query structure.
bool findAllMappings(const MolecularGraph &target)
Searches for all atom/bond mappings of query subgraphs to substructures of the specified target molec...
void uniqueMappingsOnly(bool unique)
Allows to specify whether or not to store only unique atom/bond mappings.
AtomBondMapping & getMapping(std::size_t idx)
Returns a non-const reference to the stored atom/bond mapping object at index idx.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
ConstMappingIterator getMappingsBegin() const
Returns a constant iterator pointing to the beginning of the stored atom/bond mapping objects.
std::size_t getMinSubstructureSize() const
Returns the minimum accepted common substructure size.
bool operator>(const Array< ValueType > &array1, const Array< ValueType > &array2)
Greater than comparison operator.
const AtomBondMapping & getMapping(std::size_t idx) const
Returns a const reference to the stored atom/bond mapping object at index idx.
~MaxCommonAtomSubstructureSearch()
Destructor.