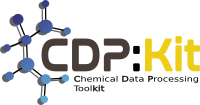 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_MATRIX_HPP
28 #define CDPL_MATH_MATRIX_HPP
35 #include <unordered_map>
36 #include <type_traits>
38 #include <initializer_list>
67 typedef typename std::conditional<std::is_const<M>::value,
68 typename M::ConstReference,
91 return data.getSize1();
96 return data.getSize2();
101 return data.getMaxSize();
106 return data.getMaxSize1();
111 return data.getMaxSize2();
116 return data.isEmpty();
131 data.operator=(
r.data);
135 template <
typename E>
142 template <
typename E>
149 template <
typename E>
156 template <
typename T>
163 template <
typename T>
170 template <
typename E>
177 template <
typename E>
184 template <
typename E>
205 template <
typename T,
typename A>
207 template <
typename T,
typename A>
210 template <
typename T>
217 typedef typename InitializerListType::value_type::value_type
ValueType;
218 typedef typename InitializerListType::value_type::const_reference
ConstReference;
219 typedef typename InitializerListType::value_type::reference
Reference;
220 typedef typename InitializerListType::size_type
SizeType;
230 for (
const auto&
r : l)
231 size2 = std::max(size2,
r.size());
238 if (j >= (list.begin() + i)->size())
241 return *((list.begin() + i)->begin() + j);
248 if (j >= (list.begin() + i)->size())
251 return *((list.begin() + i)->begin() + j);
266 return (size2 == 0 || list.size() == 0);
275 template <
typename T>
278 template <
typename T,
typename A = std::vector<T> >
301 size1(0), size2(0), data() {}
304 size1(
m), size2(n), data(storageSize(
m, n)) {}
307 size1(
m), size2(n), data(storageSize(
m, n), v) {}
310 size1(
m.size1), size2(
m.size2), data(
m.data) {}
313 size1(0), size2(0), data()
319 size1(0), size2(0), data()
324 template <
typename E>
328 matrixAssignMatrix<ScalarAssignment>(*
this, e);
360 return data.max_size();
387 template <
typename C>
398 template <
typename E>
406 template <
typename C>
417 template <
typename E>
425 template <
typename C>
436 template <
typename E>
444 template <
typename T1>
447 matrixAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
451 template <
typename T1>
454 matrixAssignScalar<ScalarDivisionAssignment>(*
this, t);
458 template <
typename E>
462 matrixAssignMatrix<ScalarAssignment>(*
this, e);
470 matrixAssignMatrix<ScalarAssignment>(*
this, ilm);
474 template <
typename E>
477 matrixAssignMatrix<ScalarAdditionAssignment>(*
this, e);
487 template <
typename E>
490 matrixAssignMatrix<ScalarSubtractionAssignment>(*
this, e);
503 std::swap(data,
m.data);
504 std::swap(size1,
m.size1);
505 std::swap(size2,
m.size2);
516 std::fill(data.begin(), data.end(), v);
521 if (size1 ==
m && size2 == n)
527 for (
SizeType i = 0, min_size1 = std::min(size1,
m); i < min_size1; i++)
528 for (
SizeType j = 0, min_size2 = std::min(size2, n); j < min_size2; j++)
529 tmp(i, j) = (*this)(i, j);
534 data.resize(storageSize(
m, n), v);
552 template <
typename T,
typename A = std::unordered_map<std::u
int64_t, T> >
576 size1(0), size2(0), data() {}
579 size1(
m), size2(n), data()
585 size1(
m.size1), size2(
m.size2), data(
m.data) {}
588 size1(0), size2(0), data()
594 size1(0), size2(0), data()
599 template <
typename E>
604 matrixAssignMatrix<ScalarAssignment>(*
this, e);
618 typename ArrayType::const_iterator it = data.find(makeKey(i, j));
620 if (it == data.end())
633 return (size1 == 0 || size2 == 0);
648 return data.max_size();
675 template <
typename C>
686 template <
typename E>
694 template <
typename C>
705 template <
typename E>
713 template <
typename C>
724 template <
typename E>
732 template <
typename T1>
735 matrixAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
739 template <
typename T1>
742 matrixAssignScalar<ScalarDivisionAssignment>(*
this, t);
746 template <
typename E>
750 matrixAssignMatrix<ScalarAssignment>(*
this, e);
758 matrixAssignMatrix<ScalarAssignment>(*
this, ilm);
762 template <
typename E>
765 matrixAssignMatrix<ScalarAdditionAssignment>(*
this, e);
775 template <
typename E>
778 matrixAssignMatrix<ScalarSubtractionAssignment>(*
this, e);
791 std::swap(data,
m.data);
792 std::swap(size1,
m.size1);
793 std::swap(size2,
m.size2);
811 for (
typename ArrayType::iterator it = data.begin(); it != data.end();) {
812 const KeyType& key = it->first;
814 if (getRowIdx(key) >=
m || getColIdx(key) >= n)
832 return (key >> (
sizeof(
SizeType) * 8));
846 template <
typename T,
typename A>
849 template <
typename T, std::
size_t N>
852 template <
typename T, std::
size_t M, std::
size_t N>
880 size1(0), size2(0) {}
895 size1(
m.size1), size2(
m.size2)
897 for (
SizeType i = 0; i < size1; i++)
898 std::copy(
m.data[i],
m.data[i] + size2, data[i]);
907 template <
typename E>
912 matrixAssignMatrix<ScalarAssignment>(*
this, e);
929 return (size1 == 0 || size2 == 0);
966 std::copy(
m.data[i],
m.data[i] +
m.size2, data[i]);
975 template <
typename C>
986 template <
typename E>
993 template <
typename C>
1004 template <
typename E>
1011 template <
typename C>
1022 template <
typename E>
1029 template <
typename T1>
1032 matrixAssignScalar<ScalarMultiplicationAssignment>(*
this, v);
1036 template <
typename T1>
1039 matrixAssignScalar<ScalarDivisionAssignment>(*
this, v);
1043 template <
typename E>
1047 matrixAssignMatrix<ScalarAssignment>(*
this, e);
1055 matrixAssignMatrix<ScalarAssignment>(*
this, ilm);
1059 template <
typename E>
1062 matrixAssignMatrix<ScalarAdditionAssignment>(*
this, e);
1072 template <
typename E>
1075 matrixAssignMatrix<ScalarSubtractionAssignment>(*
this, e);
1088 SizeType max_size1 = std::max(size1,
m.size1);
1089 SizeType max_size2 = std::max(size2,
m.size2);
1091 for (
SizeType i = 0; i < max_size1; i++)
1092 std::swap_ranges(data[i], data[i] + max_size2,
m.data[i]);
1094 std::swap(size1,
m.size1);
1095 std::swap(size2,
m.size2);
1106 for (
SizeType i = 0; i < size1; i++)
1107 std::fill(data[i], data[i] + size2, v);
1125 SizeType min_size1 = std::min(size1,
m);
1127 for (
SizeType i = 0; i < min_size1; i++)
1128 std::fill(data[i] + size2, data[i] + n, v);
1133 std::fill(data[i], data[i] + n, v);
1145 template <
typename T, std::
size_t M, std::
size_t N>
1147 template <
typename T, std::
size_t M, std::
size_t N>
1150 template <
typename T, std::
size_t M, std::
size_t N>
1180 std::fill(data[i], data[i] +
N,
ValueType());
1186 std::fill(data[i], data[i] +
N, v);
1192 std::copy(
m.data[i],
m.data[i] +
N, data[i]);
1200 template <
typename E>
1203 matrixAssignMatrix<ScalarAssignment>(*
this, e);
1220 return (
M == 0 ||
N == 0);
1257 std::copy(
m.data[i],
m.data[i] +
N, data[i]);
1263 template <
typename C>
1269 template <
typename T1>
1275 template <
typename E>
1282 template <
typename C>
1293 template <
typename E>
1300 template <
typename C>
1311 template <
typename E>
1318 template <
typename T1>
1321 matrixAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
1325 template <
typename T1>
1328 matrixAssignScalar<ScalarDivisionAssignment>(*
this, t);
1332 template <
typename E>
1335 matrixAssignMatrix<ScalarAssignment>(*
this, e);
1343 for (
SizeType i = 0; i < n_rows; i++) {
1344 const auto&
row = *(l.begin() + i);
1346 if (
row.size() <
N) {
1347 std::copy(
row.begin(),
row.end(), data[i]);
1352 std::copy(
row.begin(),
row.begin() +
N, data[i]);
1357 std::fill(data[i], data[i] +
N,
ValueType());
1362 template <
typename E>
1365 matrixAssignMatrix<ScalarAdditionAssignment>(*
this, e);
1375 template <
typename E>
1378 matrixAssignMatrix<ScalarSubtractionAssignment>(*
this, e);
1392 std::swap_ranges(data[i], data[i] +
N,
m.data[i]);
1404 std::fill(data[i], data[i] +
N, v);
1411 template <
typename T, std::
size_t M, std::
size_t N>
1413 template <
typename T, std::
size_t M, std::
size_t N>
1416 template <
typename T>
1434 size1(0), size2(0) {}
1437 size1(
m), size2(n) {}
1440 size1(
m.size1), size2(
m.size2) {}
1450 return (size1 == 0 || size2 == 0);
1465 return std::numeric_limits<SizeType>::max();
1470 return std::numeric_limits<SizeType>::max();
1486 std::swap(size1,
m.size1);
1487 std::swap(size2,
m.size2);
1508 template <
typename T>
1511 template <
typename T>
1529 size1(0), size2(0), value() {}
1532 size1(
m), size2(n), value(v) {}
1535 size1(
m.size1), size2(
m.size2), value(
m.value) {}
1545 return (size1 == 0 || size2 == 0);
1560 return std::numeric_limits<SizeType>::max();
1565 return std::numeric_limits<SizeType>::max();
1582 std::swap(size1,
m.size1);
1583 std::swap(size2,
m.size2);
1584 std::swap(value,
m.value);
1605 template <
typename T>
1623 size1(0), size2(0) {}
1626 size1(
m), size2(n) {}
1629 size1(
m.size1), size2(
m.size2) {}
1634 return (i == j ? one : zero);
1639 return (size1 == 0 || size2 == 0);
1654 return std::numeric_limits<SizeType>::max();
1659 return std::numeric_limits<SizeType>::max();
1675 std::swap(size1,
m.size1);
1676 std::swap(size2,
m.size2);
1698 template <
typename T>
1700 template <
typename T>
1703 template <
typename M>
1707 template <
typename M>
1711 template <
typename M>
1715 template <
typename M>
1719 template <
typename E>
1720 typename E::ValueType
1723 typedef typename E::ValueType ValueType;
1727 std::vector<SizeType> pv(lu.
getSize1());
1728 std::size_t num_row_swaps;
1735 for (SizeType i = 0; i < size; i++)
1738 return (num_row_swaps % 2 == 0 ? res : -res);
1741 template <
typename C>
1742 typename C::ValueType
1745 typedef typename C::ValueType ValueType;
1747 typedef typename CTemporaryType::SizeType SizeType;
1749 CTemporaryType lu(c);
1750 std::vector<SizeType> pv(lu.getSize1());
1751 std::size_t num_row_swaps;
1756 SizeType size = std::min(lu.getSize1(), lu.getSize2());
1758 for (SizeType i = 0; i < size; i++)
1761 return (num_row_swaps % 2 == 0 ? res : -res);
1764 template <
typename E,
typename C>
1768 typedef typename C::ValueType ValueType;
1770 typedef typename CTemporaryType::SizeType SizeType;
1772 CTemporaryType lu(e);
1773 std::vector<SizeType> pv(lu.getSize1());
1774 std::size_t num_row_swaps;
1784 template <
typename C>
1908 #endif // CDPL_MATH_MATRIX_HPP
BoundedMatrix & plusAssign(InitializerListType l)
Definition: Matrix.hpp:1066
ZeroMatrix< unsigned long > ULZeroMatrix
Definition: Matrix.hpp:1794
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:293
A ArrayType
Definition: Matrix.hpp:290
friend void swap(ScalarMatrix &m1, ScalarMatrix &m2)
Definition: Matrix.hpp:1588
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:221
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:564
void resize(SizeType m, SizeType n)
Definition: Matrix.hpp:807
Definition: TypeTraits.hpp:179
static const SizeType MaxSize1
Definition: Matrix.hpp:876
SparseMatrix & plusAssign(InitializerListType l)
Definition: Matrix.hpp:769
Definition: Vector.hpp:258
InitListMatrix(InitializerListType l)
Definition: Matrix.hpp:227
Matrix & operator-=(InitializerListType l)
Definition: Matrix.hpp:431
Matrix< float > FMatrix
An unbounded dense matrix holding floating point values of type float..
Definition: Matrix.hpp:1809
std::enable_if< IsScalar< T1 >::value, BoundedMatrix >::type & operator/=(const T1 &v)
Definition: Matrix.hpp:1037
InitializerListType::value_type::const_reference ConstReference
Definition: Matrix.hpp:218
void resize(SizeType m, SizeType n)
Definition: Matrix.hpp:1593
Matrix & plusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:475
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:1524
T * Pointer
Definition: Matrix.hpp:867
BoundedMatrix & operator=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:987
SizeType getSize1() const
Definition: Matrix.hpp:254
IdentityMatrix()
Definition: Matrix.hpp:1622
BoundedMatrix & operator=(InitializerListType l)
Definition: Matrix.hpp:981
friend void swap(MatrixReference &r1, MatrixReference &r2)
Definition: Matrix.hpp:196
SelfType MatrixTemporaryType
Definition: Matrix.hpp:570
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:569
IdentityMatrix< unsigned long > ULIdentityMatrix
Definition: Matrix.hpp:1804
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:1618
MatrixReference(MatrixType &m)
Definition: Matrix.hpp:76
ZeroMatrix(const ZeroMatrix &m)
Definition: Matrix.hpp:1439
ZeroMatrix< float > FZeroMatrix
Definition: Matrix.hpp:1791
std::size_t SizeType
Definition: Matrix.hpp:1615
Matrix< double > DMatrix
An unbounded dense matrix holding floating point values of type double..
Definition: Matrix.hpp:1814
friend void swap(BoundedMatrix &m1, BoundedMatrix &m2)
Definition: Matrix.hpp:1099
Matrix< T > MatrixTemporaryType
Definition: Matrix.hpp:1619
BoundedMatrix< T, M, N > MatrixTemporaryType
Definition: Matrix.hpp:1169
Definition: Matrix.hpp:1513
BoundedMatrix(SizeType m, SizeType n)
Definition: Matrix.hpp:882
CMatrix & operator=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1276
SizeType getMaxSize2() const
Definition: Matrix.hpp:947
Matrix & operator-=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:437
T & Reference
Definition: Matrix.hpp:1158
ScalarMatrix()
Definition: Matrix.hpp:1528
Definition: Matrix.hpp:212
const T & ConstReference
Definition: Matrix.hpp:1425
CMatrix< float, 2, 2 > Matrix2F
A bounded 2x2 matrix holding floating point values of type float.
Definition: Matrix.hpp:1829
Definition: SparseContainerElement.hpp:42
std::enable_if< IsScalar< T1 >::value, Matrix >::type & operator*=(const T1 &t)
Definition: Matrix.hpp:445
Matrix(const MatrixExpression< E > &e)
Definition: Matrix.hpp:325
bool isEmpty() const
Definition: Matrix.hpp:1448
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:870
SparseMatrix & operator+=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:706
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:337
void resize(SizeType m, SizeType n)
Definition: Matrix.hpp:1496
M::ConstReference ConstReference
Definition: Matrix.hpp:70
const T & Reference
Definition: Matrix.hpp:1613
SparseMatrix & operator=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:676
bool invert(const MatrixExpression< E > &e, MatrixContainer< C > &c)
Definition: Matrix.hpp:1766
SizeType getSize1() const
Definition: Matrix.hpp:89
bool isEmpty() const
Definition: Matrix.hpp:631
std::size_t SizeType
Definition: Matrix.hpp:1426
Vector< T, std::vector< T > > VectorTemporaryType
Definition: Matrix.hpp:1526
CMatrix & operator+=(InitializerListType l)
Definition: Matrix.hpp:1288
SizeType getSize2() const
Definition: Matrix.hpp:641
Matrix & operator=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:388
SparseMatrix & operator=(SparseMatrix &&m)
Definition: Matrix.hpp:669
SparseMatrix & minusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:776
void swap(CMatrix &m)
Definition: Matrix.hpp:1388
std::enable_if< IsScalar< T1 >::value, BoundedMatrix >::type & operator*=(const T1 &v)
Definition: Matrix.hpp:1030
CMatrix & operator-=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1312
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:1631
Definition: Matrix.hpp:554
A::difference_type DifferenceType
Definition: Matrix.hpp:289
void clear(const ValueType &v=ValueType())
Definition: Matrix.hpp:514
Matrix & assign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:459
CMatrix & operator=(const CMatrix &m)
Definition: Matrix.hpp:1253
SizeType getSize2() const
Definition: Matrix.hpp:1647
InitializerListType::value_type::reference Reference
Definition: Matrix.hpp:219
const ArrayType & getData() const
Definition: Matrix.hpp:368
bool isEmpty() const
Definition: Matrix.hpp:1543
Reference operator()(SizeType i, SizeType j)
Definition: Matrix.hpp:915
std::enable_if< IsScalar< T >::value, MatrixReference >::type & operator*=(const T &t)
Definition: Matrix.hpp:157
Matrix()
Definition: Matrix.hpp:300
BoundedMatrix & operator+=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:994
SizeType getSize1() const
Definition: Matrix.hpp:636
bool isEmpty() const
Definition: Matrix.hpp:264
Definition: Matrix.hpp:1152
Vector< T, std::vector< T > > VectorTemporaryType
Definition: Matrix.hpp:571
std::size_t SizeType
Definition: Matrix.hpp:1521
Definition: Matrix.hpp:60
Reference operator()(SizeType i, SizeType j)
Definition: Matrix.hpp:1206
const T(* ConstArrayPointer)[N]
Definition: Matrix.hpp:866
ValueType ArrayType[M][N]
Definition: Matrix.hpp:1162
std::initializer_list< std::initializer_list< T > > InitializerListType
Definition: Matrix.hpp:1172
ScalarMatrix< long > LScalarMatrix
Definition: Matrix.hpp:1798
const T * ConstPointer
Definition: Matrix.hpp:567
std::uint32_t SizeType
Definition: Matrix.hpp:563
CMatrix & operator+=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1294
SizeType getSize2() const
Definition: Matrix.hpp:259
Matrix< T > MatrixTemporaryType
Definition: Matrix.hpp:1525
Matrix & plusAssign(InitializerListType l)
Definition: Matrix.hpp:481
Vector< T, std::vector< T > > VectorTemporaryType
Definition: Matrix.hpp:1431
Reference operator()(SizeType i, SizeType j)
Definition: Matrix.hpp:234
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:921
SizeType getSize2() const
Definition: Matrix.hpp:1228
const SelfType ConstClosureType
Definition: Matrix.hpp:74
const T & ConstReference
Definition: Matrix.hpp:1159
BoundedMatrix & operator+=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1005
BoundedMatrix & operator=(const BoundedMatrix &m)
Definition: Matrix.hpp:962
MatrixReference & operator-=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:150
SizeType getSize1() const
Definition: Matrix.hpp:1548
std::shared_ptr< SelfType > SharedPointer
Definition: Matrix.hpp:572
SparseMatrix & operator+=(InitializerListType l)
Definition: Matrix.hpp:700
IdentityMatrix< long > LIdentityMatrix
Definition: Matrix.hpp:1803
Matrix(Matrix &&m)
Definition: Matrix.hpp:312
SizeType getSize2() const
Definition: Matrix.hpp:1553
const unsigned int N
Specifies Nitrogen.
Definition: AtomType.hpp:97
SizeType getSize1() const
Definition: Matrix.hpp:1642
Definition: Expression.hpp:76
Matrix & minusAssign(InitializerListType l)
Definition: Matrix.hpp:494
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
CMatrix< unsigned long, 4, 4 > Matrix4UL
A bounded 4x4 matrix holding unsigned integers of type unsigned long.
Definition: Matrix.hpp:1884
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:1617
SparseMatrix & minusAssign(InitializerListType l)
Definition: Matrix.hpp:782
SelfType MatrixTemporaryType
Definition: Matrix.hpp:871
Reference operator()(SizeType i, SizeType j)
Definition: Matrix.hpp:79
CMatrix< double, 4, 4 > Matrix4D
A bounded 4x4 matrix holding floating point values of type double.
Definition: Matrix.hpp:1854
CMatrix()
Definition: Matrix.hpp:1177
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:1522
ValueType ArrayType[M][N]
Definition: Matrix.hpp:864
InitializerListType::value_type::value_type ValueType
Definition: Matrix.hpp:217
MatrixReference & minusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:185
const T & Reference
Definition: Matrix.hpp:1519
static const SizeType Size1
Definition: Matrix.hpp:1174
void swap(SparseMatrix &m)
Definition: Matrix.hpp:788
Matrix & operator=(const Matrix &m)
Definition: Matrix.hpp:373
BoundedVector< T, M *N > VectorTemporaryType
Definition: Matrix.hpp:1170
CMatrix & operator=(InitializerListType l)
Definition: Matrix.hpp:1270
CMatrix< unsigned long, 3, 3 > Matrix3UL
A bounded 3x3 matrix holding unsigned integers of type unsigned long.
Definition: Matrix.hpp:1879
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:244
SizeType getMaxSize2() const
Definition: Matrix.hpp:1657
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:1167
E::SizeType luDecompose(MatrixExpression< E > &e)
Definition: LUDecomposition.hpp:45
const T & ConstReference
Definition: Matrix.hpp:1520
ZeroMatrix< double > DZeroMatrix
Definition: Matrix.hpp:1792
const MatrixType & getData() const
Definition: Matrix.hpp:119
ScalarMatrix & operator=(const ScalarMatrix &m)
Definition: Matrix.hpp:1568
void swap(ScalarMatrix &m)
Definition: Matrix.hpp:1579
Definition of a proxy type for direct assignment of vector and matrix expressions.
Matrix(const Matrix &m)
Definition: Matrix.hpp:309
SparseMatrix< long > SparseLMatrix
An unbounded sparse matrix holding signed integers of type long.
Definition: Matrix.hpp:1899
ArrayPointer getData()
Definition: Matrix.hpp:1243
void swap(BoundedMatrix &m)
Definition: Matrix.hpp:1085
CMatrix(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1201
CMatrix & plusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1363
bool isEmpty() const
Definition: Matrix.hpp:1218
MatrixReference & plusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:178
SizeType getMaxSize2() const
Definition: Matrix.hpp:1468
T ValueType
Definition: Matrix.hpp:1518
BoundedMatrix()
Definition: Matrix.hpp:879
CMatrix< double, 2, 2 > Matrix2D
A bounded 2x2 matrix holding floating point values of type double.
Definition: Matrix.hpp:1844
const T & ConstReference
Definition: Matrix.hpp:861
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:568
BoundedMatrix(SizeType m, SizeType n, const ValueType &v)
Definition: Matrix.hpp:888
BoundedMatrix & assign(InitializerListType l)
Definition: Matrix.hpp:1051
std::initializer_list< std::initializer_list< T > > InitializerListType
Definition: Matrix.hpp:573
MatrixReference & operator+=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:143
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
Definition: Matrix.hpp:1418
T(* ArrayPointer)[N]
Definition: Matrix.hpp:865
SizeType getSize2() const
Definition: Matrix.hpp:937
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:84
BoundedMatrix & minusAssign(InitializerListType l)
Definition: Matrix.hpp:1079
IdentityMatrix & operator=(const IdentityMatrix &m)
Definition: Matrix.hpp:1662
Matrix & minusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:488
BoundedMatrix(const BoundedMatrix &m)
Definition: Matrix.hpp:894
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:1523
Matrix< unsigned long > ULMatrix
An unbounded dense matrix holding unsigned integers of type unsigned long.
Definition: Matrix.hpp:1824
T(* ArrayPointer)[N]
Definition: Matrix.hpp:1163
BoundedMatrix & assign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1044
Definition: Matrix.hpp:854
SparseMatrix(SizeType m, SizeType n)
Definition: Matrix.hpp:578
SelfType ClosureType
Definition: Matrix.hpp:222
void swap(IdentityMatrix &m)
Definition: Matrix.hpp:1672
void clear()
Definition: Matrix.hpp:802
E::ValueType det(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1721
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:1212
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
Matrix & operator=(Matrix &&m)
Definition: Matrix.hpp:381
M::ValueType ValueType
Definition: Matrix.hpp:66
const ArrayType & getData() const
Definition: Matrix.hpp:656
bool isEmpty() const
Definition: Matrix.hpp:114
BoundedMatrix(const MatrixExpression< E > &e)
Definition: Matrix.hpp:908
BoundedMatrix & operator-=(InitializerListType l)
Definition: Matrix.hpp:1017
std::enable_if< IsScalar< T1 >::value, SparseMatrix >::type & operator*=(const T1 &t)
Definition: Matrix.hpp:733
bool isEmpty() const
Definition: Matrix.hpp:343
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
std::enable_if< IsScalar< T1 >::value, CMatrix >::type & operator/=(const T1 &t)
Definition: Matrix.hpp:1326
const T(* ConstArrayPointer)[N]
Definition: Matrix.hpp:1164
T ValueType
Definition: Matrix.hpp:1157
SparseMatrix & operator+=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:695
Definition of type traits.
void clear(const ValueType &v=ValueType())
Definition: Matrix.hpp:1401
MatrixReference & operator=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:136
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:863
Vector< T, std::vector< T > > VectorTemporaryType
Definition: Matrix.hpp:1620
SizeType getMaxSize2() const
Definition: Matrix.hpp:1238
Vector< T, std::vector< T > > VectorTemporaryType
Definition: Matrix.hpp:225
void resize(SizeType m, SizeType n, bool preserve=true, const ValueType &v=ValueType())
Definition: Matrix.hpp:519
SparseMatrix< float > SparseFMatrix
An unbounded sparse matrix holding floating point values of type float..
Definition: Matrix.hpp:1889
const SelfType ConstClosureType
Definition: Matrix.hpp:223
Matrix & operator-=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:426
CMatrix & assign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1333
std::initializer_list< std::initializer_list< T > > InitializerListType
Definition: Matrix.hpp:874
CMatrix & operator-=(InitializerListType l)
Definition: Matrix.hpp:1306
SparseMatrix(const SparseMatrix &m)
Definition: Matrix.hpp:584
CMatrix & assign(InitializerListType l)
Definition: Matrix.hpp:1339
IdentityMatrix< float > FIdentityMatrix
Definition: Matrix.hpp:1801
CMatrix & minusAssign(InitializerListType l)
Definition: Matrix.hpp:1382
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:1616
Definition of various matrix expression types and operations.
ScalarMatrix< float > FScalarMatrix
Definition: Matrix.hpp:1796
T * Pointer
Definition: Matrix.hpp:566
const T & ConstReference
Definition: Matrix.hpp:287
SizeType getSize2() const
Definition: Matrix.hpp:353
SizeType getMaxSize2() const
Definition: Matrix.hpp:109
CMatrix< float, 3, 3 > Matrix3F
A bounded 3x3 matrix holding floating point values of type float.
Definition: Matrix.hpp:1834
BoundedMatrix(InitializerListType l)
Definition: Matrix.hpp:901
T * Pointer
Definition: Matrix.hpp:1165
BoundedMatrix & operator+=(InitializerListType l)
Definition: Matrix.hpp:999
friend void swap(SparseMatrix &m1, SparseMatrix &m2)
Definition: Matrix.hpp:797
CMatrix< long, 2, 2 > Matrix2L
A bounded 2x2 matrix holding signed integers of type long.
Definition: Matrix.hpp:1859
Definition of an element proxy for sparse data types.
bool isEmpty() const
Definition: Matrix.hpp:1637
IdentityMatrix< double > DIdentityMatrix
Definition: Matrix.hpp:1802
void swap(ZeroMatrix &m)
Definition: Matrix.hpp:1483
Definition of various functors.
ScalarMatrix(SizeType m, SizeType n, const ValueType &v=ValueType())
Definition: Matrix.hpp:1531
M::MatrixTemporaryType Type
Definition: TypeTraits.hpp:188
CMatrix< long, 3, 3 > Matrix3L
A bounded 3x3 matrix holding signed integers of type long.
Definition: Matrix.hpp:1864
SparseMatrix & operator=(InitializerListType l)
Definition: Matrix.hpp:681
Matrix(SizeType m, SizeType n)
Definition: Matrix.hpp:303
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
CMatrix & plusAssign(InitializerListType l)
Definition: Matrix.hpp:1369
Definition: TypeTraits.hpp:186
Implementation of matrix assignment routines.
M::SizeType SizeType
Definition: Matrix.hpp:71
SparseMatrix & operator-=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:725
SparseMatrix & operator=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:687
std::enable_if< IsScalar< T1 >::value, SparseMatrix >::type & operator/=(const T1 &t)
Definition: Matrix.hpp:740
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:1161
Definition: Vector.hpp:785
Vector< T, A > VectorTemporaryType
Definition: Matrix.hpp:296
static const SizeType Size2
Definition: Matrix.hpp:1175
void resize(SizeType m, SizeType n, const ValueType &v)
Definition: Matrix.hpp:1119
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:1428
std::enable_if< IsScalar< T1 >::value, Matrix >::type & operator/=(const T1 &t)
Definition: Matrix.hpp:452
CMatrix & minusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1376
std::enable_if< IsScalar< T >::value, MatrixReference >::type & operator/=(const T &t)
Definition: Matrix.hpp:164
Matrix & operator=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:399
SparseMatrix & plusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:763
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
SparseContainerElement< SelfType > Reference
Definition: Matrix.hpp:561
Definition of exception classes.
std::size_t SizeType
Definition: Matrix.hpp:1160
SparseMatrix(InitializerListType l)
Definition: Matrix.hpp:593
const T & ConstReference
Definition: Matrix.hpp:1614
A::size_type SizeType
Definition: Matrix.hpp:288
CMatrix & operator-=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:1301
SizeType getSize1() const
Definition: Matrix.hpp:348
M::DifferenceType DifferenceType
Definition: Matrix.hpp:72
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
ArrayType & getData()
Definition: Matrix.hpp:363
T ValueType
Definition: Matrix.hpp:1612
Matrix & operator+=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:407
CMatrix(const CMatrix &m)
Definition: Matrix.hpp:1189
Matrix & operator+=(InitializerListType l)
Definition: Matrix.hpp:412
CMatrix< long, 4, 4 > Matrix4L
A bounded 4x4 matrix holding signed integers of type long.
Definition: Matrix.hpp:1869
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:1442
friend void swap(ZeroMatrix &m1, ZeroMatrix &m2)
Definition: Matrix.hpp:1491
SizeType getMaxSize1() const
Definition: Matrix.hpp:1652
CMatrix & operator+=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:1283
Definition: Matrix.hpp:280
bool isEmpty() const
Definition: Matrix.hpp:927
CMatrix< unsigned long, 2, 2 > Matrix2UL
A bounded 2x2 matrix holding unsigned integers of type unsigned long.
Definition: Matrix.hpp:1874
SparseMatrix< double > SparseDMatrix
An unbounded sparse matrix holding floating point values of type double..
Definition: Matrix.hpp:1894
ScalarMatrix(const ScalarMatrix &m)
Definition: Matrix.hpp:1534
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:1168
BoundedMatrix & operator=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:976
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:614
The namespace of the Chemical Data Processing Library.
CMatrix(const ValueType &v)
Definition: Matrix.hpp:1183
Definition: Matrix.hpp:1607
T & Reference
Definition: Matrix.hpp:286
A::key_type KeyType
Definition: Matrix.hpp:560
ZeroMatrix()
Definition: Matrix.hpp:1433
std::shared_ptr< SelfType > SharedPointer
Definition: Matrix.hpp:873
static const SizeType MaxSize2
Definition: Matrix.hpp:877
MatrixReference & assign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:171
SizeType getMaxSize() const
Definition: Matrix.hpp:358
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:1429
CMatrix & operator=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:1264
friend void swap(CMatrix &m1, CMatrix &m2)
Definition: Matrix.hpp:1396
BoundedMatrix & minusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1073
friend void swap(Matrix &m1, Matrix &m2)
Definition: Matrix.hpp:509
SparseMatrix(SparseMatrix &&m)
Definition: Matrix.hpp:587
SparseMatrix & operator-=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:714
SizeType getMaxSize1() const
Definition: Matrix.hpp:1463
SizeType getSize1() const
Definition: Matrix.hpp:1223
SizeType getMaxSize1() const
Definition: Matrix.hpp:942
SizeType getMaxSize1() const
Definition: Matrix.hpp:1233
ZeroMatrix & operator=(const ZeroMatrix &m)
Definition: Matrix.hpp:1473
std::enable_if< IsScalar< T1 >::value, CMatrix >::type & operator*=(const T1 &t)
Definition: Matrix.hpp:1319
SparseMatrix & assign(InitializerListType l)
Definition: Matrix.hpp:754
A ArrayType
Definition: Matrix.hpp:565
SparseMatrix & assign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:747
T ValueType
Definition: Matrix.hpp:285
SparseMatrix & operator=(const SparseMatrix &m)
Definition: Matrix.hpp:661
CMatrix< float, 4, 4 > Matrix4F
A bounded 4x4 matrix holding floating point values of type float.
Definition: Matrix.hpp:1839
MatrixReference< SelfType > ClosureType
Definition: Matrix.hpp:869
ScalarMatrix< double > DScalarMatrix
Definition: Matrix.hpp:1797
std::ptrdiff_t DifferenceType
Definition: Matrix.hpp:1427
BoundedVector< T, M *N > VectorTemporaryType
Definition: Matrix.hpp:872
std::initializer_list< std::initializer_list< T > > InitializerListType
Definition: Matrix.hpp:216
T ValueType
Definition: Matrix.hpp:859
MatrixRow< M > row(MatrixExpression< M > &e, typename MatrixRow< M >::SizeType i)
Definition: MatrixProxy.hpp:716
SizeType getSize2() const
Definition: Matrix.hpp:1458
const MatrixReference< const SelfType > ConstClosureType
Definition: Matrix.hpp:294
BoundedMatrix & operator-=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1023
SizeType getSize1() const
Definition: Matrix.hpp:932
void resize(SizeType m, SizeType n)
Definition: Matrix.hpp:1110
T * Pointer
Definition: Matrix.hpp:291
T ValueType
Definition: Matrix.hpp:559
IdentityMatrix(SizeType m, SizeType n)
Definition: Matrix.hpp:1625
Implementation of matrix LU-decomposition and associated operations.
const T * ConstPointer
Definition: Matrix.hpp:1166
Definition of various preprocessor macros for error checking.
SizeType getSize1() const
Definition: Matrix.hpp:1453
SparseMatrix & operator-=(InitializerListType l)
Definition: Matrix.hpp:719
SizeType getMaxSize2() const
Definition: Matrix.hpp:1563
Matrix< long > LMatrix
An unbounded dense matrix holding signed integers of type long.
Definition: Matrix.hpp:1819
#define CDPL_MATH_CHECK_MAX_SIZE(size, max_size, e)
Definition: Check.hpp:64
ConstReference operator()(SizeType i, SizeType j) const
Definition: Matrix.hpp:1537
std::shared_ptr< SelfType > SharedPointer
Definition: Matrix.hpp:297
Matrix & operator=(InitializerListType l)
Definition: Matrix.hpp:393
std::initializer_list< std::initializer_list< T > > InitializerListType
Definition: Matrix.hpp:298
Matrix & operator+=(const MatrixExpression< E > &e)
Definition: Matrix.hpp:418
SizeType getMaxSize1() const
Definition: Matrix.hpp:104
std::size_t SizeType
Definition: Matrix.hpp:862
SparseMatrix()
Definition: Matrix.hpp:575
Matrix(InitializerListType l)
Definition: Matrix.hpp:318
T ValueType
Definition: Matrix.hpp:1423
MatrixReference & operator=(const MatrixReference &r)
Definition: Matrix.hpp:129
CMatrix(InitializerListType l)
Definition: Matrix.hpp:1195
IdentityMatrix(const IdentityMatrix &m)
Definition: Matrix.hpp:1628
std::shared_ptr< SelfType > SharedPointer
Definition: Matrix.hpp:1171
ScalarMatrix< unsigned long > ULScalarMatrix
Definition: Matrix.hpp:1799
SizeType getMaxSize1() const
Definition: Matrix.hpp:1558
const T * ConstPointer
Definition: Matrix.hpp:292
void clear(const ValueType &v=ValueType())
Definition: Matrix.hpp:1104
friend void swap(IdentityMatrix &m1, IdentityMatrix &m2)
Definition: Matrix.hpp:1680
bool luSubstitute(const MatrixExpression< E1 > &lu, VectorExpression< E2 > &b)
Definition: LUDecomposition.hpp:157
Reference operator()(SizeType i, SizeType j)
Definition: Matrix.hpp:607
ZeroMatrix(SizeType m, SizeType n)
Definition: Matrix.hpp:1436
Matrix & assign(InitializerListType l)
Definition: Matrix.hpp:466
ConstArrayPointer getData() const
Definition: Matrix.hpp:957
void swap(Matrix &m)
Definition: Matrix.hpp:500
ArrayType & getData()
Definition: Matrix.hpp:651
SparseMatrix< unsigned long > SparseULMatrix
An unbounded sparse matrix holding unsigned integers of type unsigned long.
Definition: Matrix.hpp:1904
std::conditional< std::is_const< M >::value, typename M::ConstReference, typename M::Reference >::type Reference
Definition: Matrix.hpp:69
Matrix< T > MatrixTemporaryType
Definition: Matrix.hpp:1430
SizeType getNumElements() const
Definition: Matrix.hpp:626
ArrayPointer getData()
Definition: Matrix.hpp:952
BoundedMatrix & plusAssign(const MatrixExpression< E > &e)
Definition: Matrix.hpp:1060
const T * ConstPointer
Definition: Matrix.hpp:868
void swap(MatrixReference &r)
Definition: Matrix.hpp:191
Matrix< T, std::vector< T > > MatrixTemporaryType
Definition: Matrix.hpp:224
const unsigned int A
A generic type that covers any element except hydrogen.
Definition: AtomType.hpp:617
SizeType getMaxSize() const
Definition: Matrix.hpp:99
InitListMatrix SelfType
Definition: Matrix.hpp:215
T & Reference
Definition: Matrix.hpp:860
SelfType ClosureType
Definition: Matrix.hpp:73
BoundedMatrix & operator-=(const MatrixContainer< C > &c)
Definition: Matrix.hpp:1012
ArrayType::size_type getMaxSize() const
Definition: Matrix.hpp:646
ZeroMatrix< long > LZeroMatrix
Definition: Matrix.hpp:1793
MatrixType & getData()
Definition: Matrix.hpp:124
InitializerListType::size_type SizeType
Definition: Matrix.hpp:220
Definition: Expression.hpp:164
const T & ConstReference
Definition: Matrix.hpp:562
Matrix(SizeType m, SizeType n, const ValueType &v)
Definition: Matrix.hpp:306
M MatrixType
Definition: Matrix.hpp:65
Reference operator()(SizeType i, SizeType j)
Definition: Matrix.hpp:331
SizeType getSize2() const
Definition: Matrix.hpp:94
ConstArrayPointer getData() const
Definition: Matrix.hpp:1248
const T & Reference
Definition: Matrix.hpp:1424
SelfType MatrixTemporaryType
Definition: Matrix.hpp:295
void resize(SizeType m, SizeType n)
Definition: Matrix.hpp:1685
SparseMatrix(const MatrixExpression< E > &e)
Definition: Matrix.hpp:600