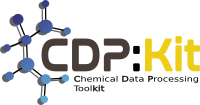 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_LINE2D_HPP
30 #define CDPL_VIS_LINE2D_HPP
71 Line2D(
double beg_x,
double beg_y,
double end_x,
double end_y);
123 void setPoints(
double beg_x,
double beg_y,
double end_x,
double end_y);
348 #endif // CDPL_VIS_LINE2D_HPP
Line2D()
Constructs a line whose starting and end point is set to (0, 0).
bool operator!=(const Line2D &line) const
Inequality comparison operator.
void setEnd(const Math::Vector2D &pt)
Sets the end point of the line to pt.
void translateBegin(const Math::Vector2D &vec)
Translates the starting point of the line by vec.
void translateEnd(const Math::Vector2D &vec)
Translates the end of the line point by vec.
void setPoints(const Math::Vector2D &beg, const Math::Vector2D &end)
Sets the starting point of the line to beg and the end point to end.
void setBegin(double x, double y)
Sets the starting point of the line to (x, y).
double getDistance(const Math::Vector2D &pt) const
Returns the perpendicular distance of the point pt to this line.
Line2D(double beg_x, double beg_y, double end_x, double end_y)
Constructs a line with the starting point set to (beg_x, beg_y) and the end point set to (end_x,...
void setEnd(double x, double y)
Sets the end point of the line to (x, y).
double getLength() const
Returns the length of the line segment.
bool setBeginToLineSegIntersection(const Line2D &line)
Sets the starting point of the line to the point of intersection with the specified line segment.
bool clipEndAgainstLineSeg(const Line2D &line)
Sets the end point of the line to the point of intersection with the specified line segment.
Math::Vector2D getCWPerpDirection() const
Calculates the normalized direction vector rotated by 90 degrees in clockwise direction.
void setPoints(double beg_x, double beg_y, double end_x, double end_y)
Sets the starting point of the line to (beg_x, beg_y) and the end point to (end_x,...
bool setEndToLineSegIntersection(const Line2D &line)
Sets the end point of the line to the point of intersection with the specified line segment.
bool clipBeginAgainstLineSeg(const Line2D &line)
Sets the starting point of the line to the point of intersection with the specified line segment.
bool clipEndAgainstRectangle(const Rectangle2D &rect)
Sets the end point of the line to the point of intersection between this line segment and the edges o...
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void setBegin(const Math::Vector2D &pt)
Sets the starting point of the line to pt.
void translate(const Math::Vector2D &vec)
Translates the starting and end point of the line by vec.
QuaternionVectorAdapter< E > vec(QuaternionExpression< E > &e)
Definition: QuaternionAdapter.hpp:237
bool operator==(const Line2D &line) const
Equality comparison operator.
void swapPoints()
Swaps the starting and end point of the line.
bool containsPoint(const Math::Vector2D &pt) const
Tests if the point pt is contained within the boundary of the line segment.
Math::Vector2D getCCWPerpDirection() const
Calculates the normalized direction vector rotated by 90 degrees in counter-clockwise direction.
bool clipBeginAgainstRectangle(const Rectangle2D &rect)
Sets the starting point of the line to the point of intersection between this line segment and the ed...
const Math::Vector2D & getBegin() const
Returns the starting point of the line.
The namespace of the Chemical Data Processing Library.
Math::Vector2D getDirection() const
Calculates the normalized direction vector of the line.
Line2D(const Math::Vector2D &beg, const Math::Vector2D &end)
Constructs a line with the specified starting and end point.
Specifies an axis aligned rectangular area in 2D space.
Definition: Rectangle2D.hpp:51
virtual ~Line2D()
Virtual destructor.
Definition: Line2D.hpp:76
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
bool getIntersectionPoint(const Line2D &line, Math::Vector2D &pt) const
Calculates the point of intersection with the specified line and stores the result in pt.
void getCWPerpDirection(Math::Vector2D &dir) const
Calculates the normalized direction vector rotated by 90 degrees in clockwise direction and stores th...
void getCenter(Math::Vector2D &ctr) const
Calculates the center point of the line and stores the result in ctr.
Definition of the preprocessor macro CDPL_VIS_API.
const Math::Vector2D & getEnd() const
Returns the end point of the line.
void getCCWPerpDirection(Math::Vector2D &dir) const
Calculates the normalized direction vector rotated by 90 degrees in counter-clockwise direction and s...
void getDirection(Math::Vector2D &dir) const
Calculates the normalized direction vector of the line and stores the result in dir.
Definition of vector data types.
Specifies a line segment in 2D space.
Definition: Line2D.hpp:48
Math::Vector2D getCenter() const
Calculates the center point of the line.