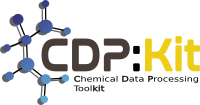 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_COMPOUNDDATAREADER_HPP
30 #define CDPL_UTIL_COMPOUNDDATAREADER_HPP
49 template <
typename DataType>
91 operator const void*()
const;
95 ReaderType* getReaderForRecordIndex(std::size_t& idx)
const;
97 typedef std::vector<ReaderPointer> ReaderArray;
98 typedef std::vector<std::size_t> RecordIndexArray;
102 RecordIndexArray recordIdxBounds;
103 std::size_t recordIdx;
104 std::size_t numRecords;
112 template <
typename DataType>
114 state(false), recordIdx(0), numRecords(0)
117 template <
typename DataType>
123 template <
typename DataType>
126 std::for_each(readers.begin(), readers.end(),
127 std::bind(&ReaderType::setParent, std::placeholders::_1,
130 recordIdxBounds.clear();
137 template <
typename DataType>
140 readers.reserve(readers.size() + 1);
141 recordIdxBounds.reserve(readers.size() + 1);
143 reader->setParent(
this);
145 std::size_t num_recs = reader->getNumRecords();
147 readers.push_back(reader);
148 numRecords += num_recs;
149 recordIdxBounds.push_back(numRecords);
151 state |=
static_cast<bool>(reader->operator
const void*());
154 template <
typename DataType>
157 if (idx >= readers.size())
160 std::size_t num_lost_records = readers[idx]->getNumRecords();
162 readers[idx]->setParent(0);
164 readers.erase(readers.begin() + idx);
165 recordIdxBounds.erase(recordIdxBounds.begin() + idx);
167 for (; idx < readers.size(); idx++)
168 recordIdxBounds[idx] -= num_lost_records;
170 numRecords -= num_lost_records;
173 template <
typename DataType>
176 return readers.size();
179 template <
typename DataType>
183 if (idx >= readers.size())
189 template <
typename DataType>
195 if (recordIdx >= numRecords)
198 std::size_t idx = recordIdx;
199 ReaderType* reader = getReaderForRecordIndex(idx);
201 if (reader && (state = reader->
read(idx, obj, overwrite))) {
203 this->invokeIOCallbacks(1.0);
209 template <
typename DataType>
215 return read(obj, overwrite);
218 template <
typename DataType>
224 if (recordIdx >= numRecords)
230 this->invokeIOCallbacks(1.0);
235 template <
typename DataType>
238 return (recordIdx < numRecords);
241 template <
typename DataType>
247 template <
typename DataType>
250 if (idx > numRecords)
256 template <
typename DataType>
262 template <
typename DataType>
265 return (state ?
this : 0);
268 template <
typename DataType>
274 template <
typename DataType>
277 for (std::size_t i = 0; i < readers.size(); i++) {
278 if (idx < recordIdxBounds[i])
285 template <
typename DataType>
289 for (std::size_t i = 0; i < readers.size(); i++) {
290 if (idx < recordIdxBounds[i]) {
291 idx -= (i == 0 ? std::size_t(0) : recordIdxBounds[i - 1]);
292 return readers[i].get();
299 #endif // CDPL_UTIL_COMPOUNDDATAREADER_HPP
std::shared_ptr< CompoundDataReader > SharedPointer
Definition: CompoundDataReader.hpp:54
std::size_t getNumReaders()
Definition: CompoundDataReader.hpp:174
virtual DataReader & read(DataType &obj, bool overwrite=true)=0
Reads the data record at the current record index and stores the read data in obj.
~CompoundDataReader()
Definition: CompoundDataReader.hpp:118
DataType DataType
The type of the read data objects.
Definition: DataReader.hpp:79
CompoundDataReader.
Definition: CompoundDataReader.hpp:51
Definition of the class CDPL::Base::DataReader.
CompoundDataReader & read(DataType &obj, bool overwrite=true)
Reads the data record at the current record index and stores the read data in obj.
Definition: CompoundDataReader.hpp:191
CompoundDataReader & operator=(const CompoundDataReader &)=delete
CompoundDataReader & skip()
Skips the data record at the current record index.
Definition: CompoundDataReader.hpp:220
CompoundDataReader()
Definition: CompoundDataReader.hpp:113
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
std::size_t getReaderIDForRecordIndex(std::size_t idx) const
Definition: CompoundDataReader.hpp:275
Base::DataReader< DataType > ReaderType
Definition: CompoundDataReader.hpp:56
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
void clear()
Definition: CompoundDataReader.hpp:124
ReaderType::SharedPointer ReaderPointer
Definition: CompoundDataReader.hpp:57
void addReader(const ReaderPointer &reader)
Definition: CompoundDataReader.hpp:138
Definition of exception classes.
bool hasMoreData()
Tells if there are any data records left to read.
Definition: CompoundDataReader.hpp:236
std::shared_ptr< DataReader > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated DataReader instances.
Definition: DataReader.hpp:84
std::size_t getNumRecords()
Returns the total number of available data records.
Definition: CompoundDataReader.hpp:257
CompoundDataReader(const CompoundDataReader &)=delete
The namespace of the Chemical Data Processing Library.
const ReaderPointer & getReader(std::size_t idx) const
Definition: CompoundDataReader.hpp:181
bool operator!() const
Definition: CompoundDataReader.hpp:269
std::size_t getRecordIndex() const
Definition: CompoundDataReader.hpp:242
void setRecordIndex(std::size_t idx)
Sets the index of the current data record to idx.
Definition: CompoundDataReader.hpp:248
void removeReader(std::size_t idx)
Definition: CompoundDataReader.hpp:155