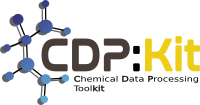 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_BONDCONTAINER_HPP
30 #define CDPL_CHEM_BONDCONTAINER_HPP
56 class ConstBondAccessor;
197 ConstBondAccessor(
const BondAccessor& accessor):
198 container(accessor.container) {}
203 const Bond& operator()(std::size_t idx)
const
205 return container->getBond(idx);
208 bool operator==(
const ConstBondAccessor& accessor)
const
210 return (container == accessor.container);
213 ConstBondAccessor& operator=(
const BondAccessor& accessor)
215 container = accessor.container;
220 const BondContainer* container;
226 friend class ConstBondAccessor;
229 BondAccessor(BondContainer* cntnr):
232 Bond& operator()(std::size_t idx)
const
234 return container->getBond(idx);
237 bool operator==(
const BondAccessor& accessor)
const
239 return (container == accessor.container);
243 BondContainer* container;
249 #endif // CDPL_CHEM_BONDCONTAINER_HPP
BondContainer & getBonds()
Returns a reference to itself.
Definition: BondContainer.hpp:174
ConstBondIterator begin() const
Returns a constant iterator pointing to the beginning of the stored const Chem::Bond objects.
virtual bool containsBond(const Bond &bond) const =0
Tells whether the specified Chem::Bond instance is stored in this container.
Definition of the preprocessor macro CDPL_CHEM_API.
Definition of the class CDPL::Util::IndexedElementIterator.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Bond.
Definition: Bond.hpp:50
const BondContainer & getBonds() const
Returns a const reference to itself.
Definition: BondContainer.hpp:165
GridEquality< E1, E2 >::ResultType operator==(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:339
virtual std::size_t getNumBonds() const =0
Returns the number of stored Chem::Bond objects.
virtual ~BondContainer()
Virtual destructor.
Definition: BondContainer.hpp:183
virtual std::size_t getBondIndex(const Bond &bond) const =0
Returns the index of the specified Chem::Bond instance in this container.
Util::IndexedElementIterator< Bond, BondAccessor > BondIterator
A mutable random access iterator used to iterate over the stored Chem::Bond objects.
Definition: BondContainer.hpp:68
virtual Bond & getBond(std::size_t idx)=0
Returns a non-const reference to the bond at index idx.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
Type definition of a generic wrapper class for storing user-defined Chem::Bond compare functions.
BondIterator begin()
Returns a mutable iterator pointing to the beginning of the stored Chem::Bond objects.
Util::IndexedElementIterator< const Bond, ConstBondAccessor > ConstBondIterator
A constant random access iterator used to iterate over the stored const Chem::Bond objects.
Definition: BondContainer.hpp:57
virtual const Bond & getBond(std::size_t idx) const =0
Returns a const reference to the Chem::Bond instance at index idx.
virtual void orderBonds(const BondCompareFunction &func)=0
Orders the stored bonds according to criteria implemented by the provided bond comparison function.
std::function< bool(const Chem::Bond &, const Chem::Bond &)> BondCompareFunction
A generic wrapper class used to store a user-defined bond compare function.
Definition: BondCompareFunction.hpp:41
BondContainer & operator=(const BondContainer &cntnr)
Assignment operator.
The namespace of the Chemical Data Processing Library.
A common interface for data-structures that support a random access to stored Chem::Bond instances.
Definition: BondContainer.hpp:54
BondIterator end()
Returns a mutable iterator pointing to the end of the stored Chem::Bond objects.
ConstBondIterator end() const
Returns a constant iterator pointing to the end of the stored const Chem::Bond objects.