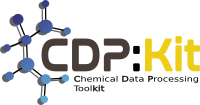 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_BASICREACTION_HPP
30 #define CDPL_CHEM_BASICREACTION_HPP
35 #include <boost/iterator/indirect_iterator.hpp>
56 typedef ComponentCache::SharedObjectPointer ComponentPtr;
57 typedef std::vector<ComponentPtr> ComponentList;
165 using Reaction::operator=;
204 void removeReactant(std::size_t idx);
205 void removeAgent(std::size_t idx);
206 void removeProduct(std::size_t idx);
208 void removeReactants();
210 void removeProducts();
221 void copyComponents(
const Reaction& rxn);
223 ComponentPtr allocComponent(
const Molecule* mol);
226 ComponentList components;
227 std::size_t agentsStartIdx;
228 std::size_t productsStartIdx;
233 #endif // CDPL_CHEM_BASICREACTION_HPP
Definition of the class CDPL::Util::ObjectPool.
const BasicMolecule & getComponent(std::size_t idx) const
Returns a const reference to the reaction component at index idx.
void clear()
Removes all components and clears all properties of the reaction.
Definition of the preprocessor macro CDPL_CHEM_API.
void removeComponent(std::size_t idx, unsigned int role)
Removes the reaction component at index idx in the list of components with the specified role.
void copy(const BasicReaction &rxn)
Replaces the current set of reaction components and properties by a copy of the components and proper...
ConstComponentIterator getComponentsEnd() const
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void copy(const Reaction &rxn)
Replaces the current set of reaction components and properties by a copy of the components and proper...
BasicMolecule.
Definition: BasicMolecule.hpp:54
void removeComponent(std::size_t idx)
Removes the reaction component at the specified index.
std::size_t getNumComponents() const
Returns the number of reaction components.
BasicReaction(const BasicReaction &rxn)
Constructs a copy of the BasicReaction instance rxn.
ConstComponentIterator getComponentsBegin() const
std::size_t getNumComponents(unsigned int role) const
Returns the number of reaction components with the specified role.
bool containsComponent(const Molecule &mol) const
Tells whether the specified molecule is a component of this reaction.
Molecule.
Definition: Molecule.hpp:49
BasicReaction()
Constructs an empty BasicReaction instance.
ComponentIterator getComponentsBegin(unsigned int role)
ComponentIterator getComponentsEnd(unsigned int role)
std::shared_ptr< Reaction > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Reaction instances.
Definition: Reaction.hpp:55
CDPL_CHEM_API void clearComponents(MolecularGraph &molgraph)
std::size_t getComponentIndex(const Molecule &mol) const
Returns the index of the specified reaction component.
Definition of the class CDPL::Chem::Reaction.
const BasicMolecule & getComponent(std::size_t idx, unsigned int role) const
Returns a const reference to the reaction component at index idx in the list of components with the s...
BasicMolecule & addComponent(unsigned int role, const Molecule &mol)
Creates a new reaction component with the specified role that is a copy of the molecule mol.
void swapComponentRoles(unsigned int role1, unsigned int role2)
Swaps the reaction roles of the component sets specified by role1 and role2.
std::shared_ptr< BasicReaction > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated BasicReaction instances.
Definition: BasicReaction.hpp:63
Reaction::SharedPointer clone() const
Creates a copy of the current reaction state.
ComponentIterator getComponentsEnd()
The namespace of the Chemical Data Processing Library.
Reaction.
Definition: Reaction.hpp:52
BasicMolecule & addComponent(unsigned int role)
Creates a new reaction component with the specified role.
~BasicReaction()
Destructor.
BasicMolecule & getComponent(std::size_t idx, unsigned int role)
Returns a non-const reference to the reaction component at index idx in the list of components with t...
ConstComponentIterator getComponentsEnd(unsigned int role) const
unsigned int getComponentRole(const Molecule &mol) const
Returns the reaction role of the specified component.
void removeComponents(unsigned int role)
Removes all components with the specified role.
boost::indirect_iterator< ComponentList::const_iterator, const BasicMolecule > ConstComponentIterator
Definition: BasicReaction.hpp:67
ConstComponentIterator getComponentsBegin(unsigned int role) const
BasicReaction.
Definition: BasicReaction.hpp:53
boost::indirect_iterator< ComponentList::iterator, BasicMolecule > ComponentIterator
Definition: BasicReaction.hpp:66
ComponentIterator getComponentsBegin()
BasicReaction(const Reaction &rxn)
Constructs a copy of the Chem::Reaction instance rxn.
ComponentIterator removeComponent(const ComponentIterator &it)
BasicReaction & operator=(const BasicReaction &rxn)
Replaces the current set of reaction components and properties by a copy of the components and proper...
BasicMolecule & getComponent(std::size_t idx)
Returns a non-const reference to the reaction component at index idx.
Definition of the class CDPL::Chem::BasicMolecule.