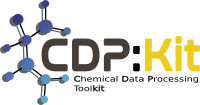 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_VECTOR_HPP
28 #define CDPL_MATH_VECTOR_HPP
35 #include <unordered_map>
36 #include <type_traits>
37 #include <initializer_list>
65 typedef typename std::conditional<std::is_const<V>::value,
66 typename V::ConstReference,
99 return data.getSize();
104 return data.getMaxSize();
109 return data.isEmpty();
124 data.operator=(
r.data);
128 template <
typename E>
135 template <
typename E>
142 template <
typename E>
149 template <
typename T>
156 template <
typename T>
163 template <
typename E>
170 template <
typename E>
177 template <
typename E>
198 template <
typename T,
typename A>
201 template <
typename T>
208 typedef typename InitializerListType::value_type
ValueType;
210 typedef typename InitializerListType::reference
Reference;
211 typedef typename InitializerListType::size_type
SizeType;
233 return *(list.begin() + i);
239 return *(list.begin() + i);
249 return (list.size() == 0);
256 template <
typename T,
typename A = std::vector<T> >
281 data(storageSize(n)) {}
284 data(storageSize(n), v) {}
293 data(std::move(v.data)) {}
298 template <
typename E>
300 data(storageSize(e().
getSize()))
302 vectorAssignVector<ScalarAssignment>(*
this, e);
339 return data.max_size();
360 data = std::move(v.data);
369 template <
typename C>
375 template <
typename E>
383 template <
typename C>
394 template <
typename E>
402 template <
typename C>
413 template <
typename E>
421 template <
typename T1>
424 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
428 template <
typename T1>
431 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
435 template <
typename E>
439 vectorAssignVector<ScalarAssignment>(*
this, e);
449 template <
typename E>
452 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
462 template <
typename E>
465 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
478 std::swap(data, v.data);
488 std::fill(data.begin(), data.end(), v);
493 data.resize(storageSize(n), v);
505 template <
typename T,
typename A = std::unordered_map<std::
size_t, T> >
531 data(), size(storageSize(n)) {}
534 data(v.data), size(v.size) {}
547 template <
typename E>
574 typename ArrayType::const_iterator it = data.find(i);
576 if (it == data.end())
599 return std::min(
SizeType(data.max_size()), std::numeric_limits<SizeType>::max());
630 template <
typename C>
636 template <
typename E>
644 template <
typename C>
655 template <
typename E>
663 template <
typename C>
674 template <
typename E>
682 template <
typename T1>
685 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
689 template <
typename T1>
692 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
696 template <
typename E>
700 vectorAssignVector<ScalarAssignment>(*
this, e);
711 template <
typename E>
714 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
724 template <
typename E>
727 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
740 std::swap(data, v.data);
741 std::swap(size, v.size);
759 for (
typename ArrayType::iterator it = data.begin(); it != data.end();) {
780 template <
typename T,
typename A>
783 template <
typename T, std::
size_t N>
824 std::copy(v.data, v.data + v.size, data);
832 template <
typename E>
889 std::copy(v.data, v.data + v.size, data);
901 template <
typename C>
907 template <
typename E>
914 template <
typename C>
925 template <
typename E>
932 template <
typename C>
943 template <
typename E>
950 template <
typename T1>
953 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
957 template <
typename T1>
960 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
964 template <
typename E>
968 vectorAssignVector<ScalarAssignment>(*
this, e);
975 std::copy(l.begin(), l.begin() + size, data);
979 template <
typename E>
982 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
992 template <
typename E>
995 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
1008 std::swap_ranges(data, data + std::max(size, v.size), v.data);
1009 std::swap(size, v.size);
1020 std::fill(data, data + size, v);
1025 size = storageSize(n);
1033 std::fill(data + size, data + n, v);
1048 template <
typename T, std::
size_t N>
1051 template <
typename T, std::
size_t N>
1086 std::copy(v.data, v.data +
N, data);
1094 template <
typename E>
1097 vectorAssignVector<ScalarAssignment>(*
this, e);
1150 std::copy(v.data, v.data +
N, data);
1160 template <
typename C>
1166 template <
typename E>
1173 template <
typename C>
1184 template <
typename E>
1191 template <
typename C>
1202 template <
typename E>
1209 template <
typename T1>
1212 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
1216 template <
typename T1>
1219 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
1223 template <
typename E>
1226 vectorAssignVector<ScalarAssignment>(*
this, e);
1233 std::copy(l.begin(), l.begin() + n, data);
1241 template <
typename E>
1244 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
1254 template <
typename E>
1257 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
1270 std::swap_ranges(data, data +
N, v.data);
1280 std::fill(data, data +
N, v);
1287 template <
typename T, std::
size_t N>
1290 template <
typename T>
1338 return std::numeric_limits<SizeType>::max();
1355 std::swap(size, v.size);
1368 template <
typename T>
1371 template <
typename T>
1388 size(0), index(0) {}
1391 size(n), index(i) {}
1394 size(v.size), index(v.index) {}
1405 return (i == index ? one : zero);
1425 return std::numeric_limits<SizeType>::max();
1446 std::swap(size, v.size);
1447 std::swap(index, v.index);
1463 template <
typename T>
1465 template <
typename T>
1468 template <
typename T>
1488 size(n), value(v) {}
1491 size(v.size), value(v.value) {}
1516 return std::numeric_limits<SizeType>::max();
1537 std::swap(size, v.size);
1538 std::swap(value, v.value);
1552 template <
typename V>
1556 template <
typename V>
1560 template <
typename T1,
typename T2>
1562 vec(
const T1& t1,
const T2& t2)
1572 template <
typename T1,
typename T2,
typename T3>
1573 CVector<typename CommonType<typename CommonType<T1, T2>::Type, T3>::Type, 3>
1574 vec(
const T1& t1,
const T2& t2,
const T3& t3)
1585 template <
typename T1,
typename T2,
typename T3,
typename T4>
1586 CVector<typename CommonType<typename CommonType<typename CommonType<T1, T2>::Type, T3>::Type, T4>::Type, 4>
1587 vec(
const T1& t1,
const T2& t2,
const T3& t3,
const T4& t4)
1721 #endif // CDPL_MATH_VECTOR_HPP
CVector< double, 4 > Vector4D
A bounded 4 element vector holding floating point values of type double.
Definition: Vector.hpp:1642
Definition: Vector.hpp:1373
BoundedVector & operator+=(const VectorContainer< C > &c)
Definition: Vector.hpp:915
static const SizeType Size
Definition: Vector.hpp:1072
ZeroVector< double > DZeroVector
Definition: Vector.hpp:1605
std::shared_ptr< SelfType > SharedPointer
Definition: Vector.hpp:801
Definition: TypeTraits.hpp:179
std::initializer_list< T > InitializerListType
Definition: Vector.hpp:525
std::enable_if< IsScalar< T1 >::value, Vector >::type & operator/=(const T1 &t)
Definition: Vector.hpp:429
Definition: Vector.hpp:258
Vector & operator+=(const VectorContainer< C > &c)
Definition: Vector.hpp:384
void clear()
Definition: Vector.hpp:750
Implementation of vector assignment routines.
BoundedVector & operator+=(InitializerListType l)
Definition: Vector.hpp:920
friend void swap(Vector &v1, Vector &v2)
Definition: Vector.hpp:481
A ArrayType
Definition: Vector.hpp:268
const T & Reference
Definition: Vector.hpp:1476
T ValueType
Definition: Vector.hpp:1297
bool isEmpty() const
Definition: Vector.hpp:327
InitializerListType::size_type SizeType
Definition: Vector.hpp:211
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:1067
bool isEmpty() const
Definition: Vector.hpp:1408
SparseVector & operator+=(const VectorExpression< E > &e)
Definition: Vector.hpp:656
BoundedVector & operator+=(const VectorExpression< E > &e)
Definition: Vector.hpp:926
friend void swap(ScalarVector &v1, ScalarVector &v2)
Definition: Vector.hpp:1542
Reference operator[](SizeType i)
Definition: Vector.hpp:77
T * Pointer
Definition: Vector.hpp:269
void swap(ZeroVector &v)
Definition: Vector.hpp:1352
UnitVector(const UnitVector &v)
Definition: Vector.hpp:1393
CVector< float, 4 > Vector4F
A bounded 4 element vector holding floating point values of type float.
Definition: Vector.hpp:1627
SizeType getSize() const
Definition: Vector.hpp:1331
InitListVector SelfType
Definition: Vector.hpp:206
ZeroVector(SizeType n)
Definition: Vector.hpp:1309
Definition: SparseContainerElement.hpp:42
const T * ConstPointer
Definition: Vector.hpp:797
Vector(SizeType n, const ValueType &v)
Definition: Vector.hpp:283
SparseVector & operator=(const SparseVector &v)
Definition: Vector.hpp:612
std::enable_if< IsScalar< T1 >::value, CVector >::type & operator*=(const T1 &t)
Definition: Vector.hpp:1210
V::DifferenceType DifferenceType
Definition: Vector.hpp:70
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:1480
Reference operator[](SizeType i)
Definition: Vector.hpp:554
Vector & operator=(Vector &&v)
Definition: Vector.hpp:358
UnitVector(SizeType n, SizeType i)
Definition: Vector.hpp:1390
SizeType getSize() const
Definition: Vector.hpp:592
ScalarVector< double > DScalarVector
Definition: Vector.hpp:1600
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:1301
const SelfType ConstClosureType
Definition: Vector.hpp:72
Vector()
Definition: Vector.hpp:277
Definition: Vector.hpp:1470
Vector< T > VectorTemporaryType
Definition: Vector.hpp:1385
void swap(UnitVector &v)
Definition: Vector.hpp:1443
SelfType ClosureType
Definition: Vector.hpp:213
Vector & operator=(const Vector &v)
Definition: Vector.hpp:352
SparseVector & plusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:712
bool isEmpty() const
Definition: Vector.hpp:587
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
Definition: Expression.hpp:54
Vector< float > FVector
An unbounded dense vector holding floating point values of type float.
Definition: Vector.hpp:1682
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:82
std::enable_if< IsScalar< T1 >::value, SparseVector >::type & operator/=(const T1 &t)
Definition: Vector.hpp:690
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:798
const ArrayType & getData() const
Definition: Vector.hpp:607
std::initializer_list< T > InitializerListType
Definition: Vector.hpp:207
void swap(SparseVector &v)
Definition: Vector.hpp:737
Definition: Vector.hpp:507
SizeType getSize() const
Definition: Vector.hpp:1509
Reference operator[](SizeType i)
Definition: Vector.hpp:1100
Vector & operator+=(const VectorExpression< E > &e)
Definition: Vector.hpp:395
CVector & operator=(const CVector &v)
Definition: Vector.hpp:1147
void swap(BoundedVector &v)
Definition: Vector.hpp:1005
InitializerListType::reference Reference
Definition: Vector.hpp:210
CVector & operator-=(const VectorExpression< E > &e)
Definition: Vector.hpp:1203
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:514
Definition of various vector expression types and operations.
SparseVector & operator+=(const VectorContainer< C > &c)
Definition: Vector.hpp:645
SparseContainerElement< SelfType, KeyType > Reference
Definition: Vector.hpp:517
ValueType ArrayType[N]
Definition: Vector.hpp:795
CVector(const CVector &v)
Definition: Vector.hpp:1084
std::enable_if< IsScalar< T1 >::value, Vector >::type & operator*=(const T1 &t)
Definition: Vector.hpp:422
const ContainerType & operator()() const
Definition: Expression.hpp:147
SizeType getNumElements() const
Definition: Vector.hpp:582
Pointer getData()
Definition: Vector.hpp:1137
ScalarVector()
Definition: Vector.hpp:1484
CVector & minusAssign(InitializerListType l)
Definition: Vector.hpp:1261
CVector & operator=(const VectorContainer< C > &c)
Definition: Vector.hpp:1161
const T & ConstReference
Definition: Vector.hpp:516
Reference operator()(SizeType i)
Definition: Vector.hpp:87
CVector & operator+=(const VectorExpression< E > &e)
Definition: Vector.hpp:1185
Vector(Vector &&v)
Definition: Vector.hpp:292
const T & Reference
Definition: Vector.hpp:1298
T * Pointer
Definition: Vector.hpp:796
InitializerListType::value_type ValueType
Definition: Vector.hpp:208
Reference operator()(SizeType i)
Definition: Vector.hpp:230
T ValueType
Definition: Vector.hpp:1058
bool isEmpty() const
Definition: Vector.hpp:1504
SparseVector & assign(InitializerListType l)
Definition: Vector.hpp:704
Reference operator()(SizeType i)
Definition: Vector.hpp:849
std::size_t SizeType
Definition: Vector.hpp:1300
bool isEmpty() const
Definition: Vector.hpp:107
Vector & operator-=(InitializerListType l)
Definition: Vector.hpp:408
ArrayType & getData()
Definition: Vector.hpp:602
SparseVector< double > SparseDVector
An unbounded sparse vector holding floating point values of type double.
Definition: Vector.hpp:1707
SparseVector< long > SparseLVector
An unbounded sparse vector holding signed integers of type long.
Definition: Vector.hpp:1712
const unsigned int N
Specifies Nitrogen.
Definition: AtomType.hpp:97
void resize(SizeType n, const ValueType &v)
Definition: Vector.hpp:1028
SizeType getMaxSize() const
Definition: Vector.hpp:1514
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
SizeType getMaxSize() const
Definition: Vector.hpp:871
Vector & plusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:450
void clear(const ValueType &v=ValueType())
Definition: Vector.hpp:486
const T * ConstPointer
Definition: Vector.hpp:1065
A::size_type SizeType
Definition: Vector.hpp:266
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:1382
Definition: Vector.hpp:203
BoundedVector(const VectorExpression< E > &e)
Definition: Vector.hpp:833
std::shared_ptr< SelfType > SharedPointer
Definition: Vector.hpp:524
CVector & operator-=(InitializerListType l)
Definition: Vector.hpp:1197
VectorReference(VectorType &v)
Definition: Vector.hpp:74
SparseVector & operator-=(InitializerListType l)
Definition: Vector.hpp:669
T ValueType
Definition: Vector.hpp:1378
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:1493
Definition of a proxy type for direct assignment of vector and matrix expressions.
CVector< long, 2 > Vector2L
A bounded 2 element vector holding signed integers of type long.
Definition: Vector.hpp:1652
Definition: Vector.hpp:58
SparseVector< unsigned long > SparseULVector
An unbounded sparse vector holding unsigned integers of type unsigned long.
Definition: Vector.hpp:1717
CVector(const VectorExpression< E > &e)
Definition: Vector.hpp:1095
CVector(const ValueType &v)
Definition: Vector.hpp:1079
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:1066
CVector & plusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:1242
CVector & minusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:1255
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
std::shared_ptr< SelfType > SharedPointer
Definition: Vector.hpp:274
const SelfType ConstClosureType
Definition: Vector.hpp:214
Vector(const Vector &v)
Definition: Vector.hpp:289
CVector & assign(const VectorExpression< E > &e)
Definition: Vector.hpp:1224
ZeroVector< long > LZeroVector
Definition: Vector.hpp:1606
VectorReference & minusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:178
Pointer getData()
Definition: Vector.hpp:876
bool isEmpty() const
Definition: Vector.hpp:1122
T & Reference
Definition: Vector.hpp:1059
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:271
friend void swap(SparseVector &v1, SparseVector &v2)
Definition: Vector.hpp:745
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
SelfType ClosureType
Definition: Vector.hpp:71
void clear(const ValueType &v=ValueType())
Definition: Vector.hpp:1278
void swap(Vector &v)
Definition: Vector.hpp:475
VectorReference & plusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:171
BoundedVector & operator-=(const VectorExpression< E > &e)
Definition: Vector.hpp:944
std::enable_if< IsScalar< T1 >::value, BoundedVector >::type & operator/=(const T1 &t)
Definition: Vector.hpp:958
CVector< double, 7 > Vector7D
A bounded 7 element vector holding floating point values of type double.
Definition: Vector.hpp:1647
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:570
CVector(InitializerListType l)
Definition: Vector.hpp:1089
Definition of type traits.
UnitVector< long > LUnitVector
Definition: Vector.hpp:1611
SizeType getIndex() const
Definition: Vector.hpp:1418
friend void swap(ZeroVector &v1, ZeroVector &v2)
Definition: Vector.hpp:1358
ScalarVector(SizeType n, const ValueType &v=ValueType())
Definition: Vector.hpp:1487
ConstPointer getData() const
Definition: Vector.hpp:1142
std::initializer_list< T > InitializerListType
Definition: Vector.hpp:802
SelfType VectorTemporaryType
Definition: Vector.hpp:523
SizeType getMaxSize() const
Definition: Vector.hpp:1423
Vector< T, std::vector< T > > VectorTemporaryType
Definition: Vector.hpp:215
T * Pointer
Definition: Vector.hpp:1064
Vector & operator-=(const VectorContainer< C > &c)
Definition: Vector.hpp:403
BoundedVector & plusAssign(InitializerListType l)
Definition: Vector.hpp:986
friend void swap(VectorReference &r1, VectorReference &r2)
Definition: Vector.hpp:189
Definition: Vector.hpp:1053
Vector(const ArrayType &data)
Definition: Vector.hpp:286
A::difference_type DifferenceType
Definition: Vector.hpp:267
SparseVector(InitializerListType l)
Definition: Vector.hpp:542
SizeType getSize() const
Definition: Vector.hpp:332
Vector< double > DVector
An unbounded dense vector holding floating point values of type double.
Definition: Vector.hpp:1687
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:1116
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:1303
SparseVector & operator=(const VectorContainer< C > &c)
Definition: Vector.hpp:631
void swap(ScalarVector &v)
Definition: Vector.hpp:1534
Definition: Vector.hpp:1292
void resize(SizeType n)
Definition: Vector.hpp:1529
Reference operator[](SizeType i)
Definition: Vector.hpp:220
Definition: Expression.hpp:142
QuaternionVectorAdapter< E > vec(QuaternionExpression< E > &e)
Definition: QuaternionAdapter.hpp:237
void resize(SizeType n)
Definition: Vector.hpp:1023
const T & ConstReference
Definition: Vector.hpp:1060
std::initializer_list< T > InitializerListType
Definition: Vector.hpp:275
void resize(SizeType n)
Definition: Vector.hpp:755
std::enable_if< IsScalar< T1 >::value, SparseVector >::type & operator*=(const T1 &t)
Definition: Vector.hpp:683
Definition of an element proxy for sparse data types.
SparseVector(const SparseVector &v)
Definition: Vector.hpp:533
Definition of various functors.
CVector & operator=(const VectorExpression< E > &e)
Definition: Vector.hpp:1167
VectorReference & operator-=(const VectorExpression< E > &e)
Definition: Vector.hpp:143
ScalarVector< float > FScalarVector
Definition: Vector.hpp:1599
ZeroVector & operator=(const ZeroVector &v)
Definition: Vector.hpp:1341
BoundedVector< T, N+1 > VectorTemporaryType
Definition: Vector.hpp:1068
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:1401
SizeType getMaxSize() const
Definition: Vector.hpp:337
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:1062
Vector(SizeType n)
Definition: Vector.hpp:280
SizeType getMaxSize() const
Definition: Vector.hpp:597
std::size_t SizeType
Definition: Vector.hpp:513
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:844
ValueType ArrayType[N]
Definition: Vector.hpp:1063
void clear(const ValueType &v=ValueType())
Definition: Vector.hpp:1018
Definition: Vector.hpp:785
static const SizeType MaxSize
Definition: Vector.hpp:804
CVector & plusAssign(InitializerListType l)
Definition: Vector.hpp:1248
SparseVector(SparseVector &&v)
Definition: Vector.hpp:536
BoundedVector< T, N+1 > VectorTemporaryType
Definition: Vector.hpp:800
Vector & plusAssign(InitializerListType l)
Definition: Vector.hpp:456
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
std::size_t SizeType
Definition: Vector.hpp:1478
ScalarVector & operator=(const ScalarVector &v)
Definition: Vector.hpp:1519
VectorType & getData()
Definition: Vector.hpp:117
Definition of exception classes.
SizeType getMaxSize() const
Definition: Vector.hpp:1132
T & Reference
Definition: Vector.hpp:264
std::enable_if< IsScalar< T >::value, VectorReference >::type & operator/=(const T &t)
Definition: Vector.hpp:157
const ArrayType & getData() const
Definition: Vector.hpp:347
UnitVector()
Definition: Vector.hpp:1387
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
CVector< long, 4 > Vector4L
A bounded 4 element vector holding signed integers of type long.
Definition: Vector.hpp:1662
BoundedVector & minusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:993
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:212
T ValueType
Definition: Vector.hpp:263
CVector()
Definition: Vector.hpp:1074
BoundedVector & operator-=(const VectorContainer< C > &c)
Definition: Vector.hpp:933
Vector & minusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:463
BoundedVector & assign(const VectorExpression< E > &e)
Definition: Vector.hpp:965
SizeType getSize() const
Definition: Vector.hpp:1127
VectorReference & operator+=(const VectorExpression< E > &e)
Definition: Vector.hpp:136
T ValueType
Definition: Vector.hpp:790
SparseVector & operator=(SparseVector &&v)
Definition: Vector.hpp:619
ArrayType & getData()
Definition: Vector.hpp:342
CVector & assign(InitializerListType l)
Definition: Vector.hpp:1230
V::SizeType SizeType
Definition: Vector.hpp:69
Reference operator[](SizeType i)
Definition: Vector.hpp:305
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:799
Reference operator()(SizeType i)
Definition: Vector.hpp:1110
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:1105
InitializerListType::const_reference ConstReference
Definition: Vector.hpp:209
BoundedVector & operator=(InitializerListType l)
Definition: Vector.hpp:896
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:794
BoundedVector & operator-=(InitializerListType l)
Definition: Vector.hpp:938
V VectorType
Definition: Vector.hpp:63
T ValueType
Definition: Vector.hpp:512
void swap(CVector &v)
Definition: Vector.hpp:1267
ConstPointer getData() const
Definition: Vector.hpp:881
SparseVector & operator=(InitializerListType l)
Definition: Vector.hpp:625
BoundedVector(InitializerListType l)
Definition: Vector.hpp:827
The namespace of the Chemical Data Processing Library.
bool isEmpty() const
Definition: Vector.hpp:861
ZeroVector(const ZeroVector &v)
Definition: Vector.hpp:1312
bool isEmpty() const
Definition: Vector.hpp:247
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:521
friend void swap(CVector &v1, CVector &v2)
Definition: Vector.hpp:1273
A ArrayType
Definition: Vector.hpp:518
SparseVector & minusAssign(InitializerListType l)
Definition: Vector.hpp:731
void resize(SizeType n, const ValueType &v=ValueType())
Definition: Vector.hpp:491
const T & ConstReference
Definition: Vector.hpp:792
ScalarVector(const ScalarVector &v)
Definition: Vector.hpp:1490
CVector & operator=(InitializerListType l)
Definition: Vector.hpp:1155
Reference operator()(SizeType i)
Definition: Vector.hpp:315
SparseVector & plusAssign(InitializerListType l)
Definition: Vector.hpp:718
ScalarVector< long > LScalarVector
Definition: Vector.hpp:1601
const T & ConstReference
Definition: Vector.hpp:1380
CVector & operator+=(const VectorContainer< C > &c)
Definition: Vector.hpp:1174
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:1320
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:522
SelfType VectorTemporaryType
Definition: Vector.hpp:273
SparseVector(const VectorExpression< E > &e)
Definition: Vector.hpp:548
BoundedVector & minusAssign(InitializerListType l)
Definition: Vector.hpp:999
std::enable_if< IsScalar< T1 >::value, CVector >::type & operator/=(const T1 &t)
Definition: Vector.hpp:1217
std::size_t SizeType
Definition: Vector.hpp:1061
Vector(const VectorExpression< E > &e)
Definition: Vector.hpp:299
UnitVector< double > DUnitVector
Definition: Vector.hpp:1610
SparseVector & operator+=(InitializerListType l)
Definition: Vector.hpp:650
const T & ConstReference
Definition: Vector.hpp:1299
ZeroVector()
Definition: Vector.hpp:1306
CVector< double, 2 > Vector2D
A bounded 2 element vector holding floating point values of type double.
Definition: Vector.hpp:1632
Vector & assign(InitializerListType l)
Definition: Vector.hpp:443
SparseVector & minusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:725
Vector< unsigned long > ULVector
An unbounded dense vector holding unsigned integers of type unsigned long.
Definition: Vector.hpp:1697
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:559
void swap(VectorReference &r)
Definition: Vector.hpp:184
std::size_t SizeType
Definition: Vector.hpp:1381
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:92
SizeType getSize() const
Definition: Vector.hpp:242
Reference operator[](SizeType i)
Definition: Vector.hpp:839
BoundedVector()
Definition: Vector.hpp:806
void resize(SizeType n)
Definition: Vector.hpp:1438
Vector< T > VectorTemporaryType
Definition: Vector.hpp:1304
V::ValueType ValueType
Definition: Vector.hpp:64
UnitVector< unsigned long > ULUnitVector
Definition: Vector.hpp:1612
std::enable_if< IsScalar< T >::value, VectorReference >::type & operator*=(const T &t)
Definition: Vector.hpp:150
SizeType getMaxSize() const
Definition: Vector.hpp:1336
const T * ConstPointer
Definition: Vector.hpp:520
BoundedVector(SizeType n, const ValueType &v)
Definition: Vector.hpp:815
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:1481
const T & ConstReference
Definition: Vector.hpp:1477
void resize(SizeType n)
Definition: Vector.hpp:1347
std::enable_if< IsScalar< T1 >::value, BoundedVector >::type & operator*=(const T1 &t)
Definition: Vector.hpp:951
Vector & operator+=(InitializerListType l)
Definition: Vector.hpp:389
CVector & operator+=(InitializerListType l)
Definition: Vector.hpp:1179
VectorReference & operator=(const VectorReference &r)
Definition: Vector.hpp:122
UnitVector & operator=(const UnitVector &v)
Definition: Vector.hpp:1428
SparseVector< float > SparseFVector
An unbounded sparse vector holding floating point values of type float.
Definition: Vector.hpp:1702
VectorReference & assign(const VectorExpression< E > &e)
Definition: Vector.hpp:164
VectorReference & operator=(const VectorExpression< E > &e)
Definition: Vector.hpp:129
friend void swap(BoundedVector &v1, BoundedVector &v2)
Definition: Vector.hpp:1013
std::initializer_list< T > InitializerListType
Definition: Vector.hpp:1070
BoundedVector(const BoundedVector &v)
Definition: Vector.hpp:821
Vector(InitializerListType l)
Definition: Vector.hpp:295
SparseVector & operator-=(const VectorExpression< E > &e)
Definition: Vector.hpp:675
A::key_type KeyType
Definition: Vector.hpp:515
std::conditional< std::is_const< V >::value, typename V::ConstReference, typename V::Reference >::type Reference
Definition: Vector.hpp:67
CVector< float, 3 > Vector3F
A bounded 3 element vector holding floating point values of type float.
Definition: Vector.hpp:1622
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:225
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:855
CVector< unsigned long, 3 > Vector3UL
A bounded 3 element vector holding unsigned integers of type unsigned long.
Definition: Vector.hpp:1672
std::ptrdiff_t DifferenceType
Definition: Vector.hpp:1479
ScalarVector< unsigned long > ULScalarVector
Definition: Vector.hpp:1602
Vector & operator-=(const VectorExpression< E > &e)
Definition: Vector.hpp:414
std::size_t SizeType
Definition: Vector.hpp:793
T * Pointer
Definition: Vector.hpp:519
ZeroVector< unsigned long > ULZeroVector
Definition: Vector.hpp:1607
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:1396
BoundedVector & operator=(const BoundedVector &v)
Definition: Vector.hpp:886
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:1498
SparseVector & operator-=(const VectorContainer< C > &c)
Definition: Vector.hpp:664
const VectorType & getData() const
Definition: Vector.hpp:112
SparseVector(SizeType n)
Definition: Vector.hpp:530
Vector< T > VectorTemporaryType
Definition: Vector.hpp:1482
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:1383
Definition of various preprocessor macros for error checking.
const T & Reference
Definition: Vector.hpp:1379
UnitVector< float > FUnitVector
Definition: Vector.hpp:1609
#define CDPL_MATH_CHECK_MAX_SIZE(size, max_size, e)
Definition: Check.hpp:64
std::shared_ptr< SelfType > SharedPointer
Definition: Vector.hpp:1069
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:236
Vector & operator=(InitializerListType l)
Definition: Vector.hpp:364
SizeType getSize() const
Definition: Vector.hpp:1413
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:272
Vector & minusAssign(InitializerListType l)
Definition: Vector.hpp:469
Vector & assign(const VectorExpression< E > &e)
Definition: Vector.hpp:436
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:1315
const T & ConstReference
Definition: Vector.hpp:265
BoundedVector & plusAssign(const VectorExpression< E > &e)
Definition: Vector.hpp:980
Vector< long > LVector
An unbounded dense vector holding signed integers of type long.
Definition: Vector.hpp:1692
BoundedVector & assign(InitializerListType l)
Definition: Vector.hpp:972
friend void swap(UnitVector &v1, UnitVector &v2)
Definition: Vector.hpp:1451
CVector< long, 3 > Vector3L
A bounded 3 element vector holding signed integers of type long.
Definition: Vector.hpp:1657
SparseVector()
Definition: Vector.hpp:527
CVector< float, 2 > Vector2F
A bounded 2 element vector holding floating point values of type float.
Definition: Vector.hpp:1617
Reference operator()(SizeType i)
Definition: Vector.hpp:564
Vector & operator=(const VectorExpression< E > &e)
Definition: Vector.hpp:376
CVector & operator-=(const VectorContainer< C > &c)
Definition: Vector.hpp:1192
InitListVector(InitializerListType l)
Definition: Vector.hpp:217
CVector< unsigned long, 4 > Vector4UL
A bounded 4 element vector holding unsigned integers of type unsigned long.
Definition: Vector.hpp:1677
T ValueType
Definition: Vector.hpp:1475
SizeType getSize() const
Definition: Vector.hpp:866
SizeType getMaxSize() const
Definition: Vector.hpp:102
const unsigned int A
A generic type that covers any element except hydrogen.
Definition: AtomType.hpp:617
T & Reference
Definition: Vector.hpp:791
ConstReference operator[](SizeType i) const
Definition: Vector.hpp:310
BoundedVector(SizeType n)
Definition: Vector.hpp:809
SparseVector & operator=(const VectorExpression< E > &e)
Definition: Vector.hpp:637
ZeroVector< float > FZeroVector
Definition: Vector.hpp:1604
SizeType getSize() const
Definition: Vector.hpp:97
const T * ConstPointer
Definition: Vector.hpp:270
Vector & operator=(const VectorContainer< C > &c)
Definition: Vector.hpp:370
BoundedVector & operator=(const VectorContainer< C > &c)
Definition: Vector.hpp:902
SparseVector & assign(const VectorExpression< E > &e)
Definition: Vector.hpp:697
V::ConstReference ConstReference
Definition: Vector.hpp:68
BoundedVector & operator=(const VectorExpression< E > &e)
Definition: Vector.hpp:908
const VectorReference< const SelfType > ConstClosureType
Definition: Vector.hpp:1384
ConstReference operator()(SizeType i) const
Definition: Vector.hpp:321
CVector< unsigned long, 2 > Vector2UL
A bounded 2 element vector holding unsigned integers of type unsigned long.
Definition: Vector.hpp:1667
VectorReference< SelfType > ClosureType
Definition: Vector.hpp:1302
bool isEmpty() const
Definition: Vector.hpp:1326