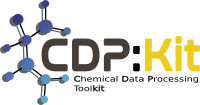 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_VECTORPROXY_HPP
28 #define CDPL_MATH_VECTORPROXY_HPP
30 #include <type_traits>
58 typedef typename std::conditional<std::is_const<V>::value,
59 typename V::ConstReference,
61 typedef typename std::conditional<std::is_const<V>::value,
62 typename V::ConstClosureType,
73 return data(range(i));
78 return data(range(i));
83 return data[range(i)];
88 return data[range(i)];
122 template <
typename E>
129 template <
typename E>
136 template <
typename E>
143 template <
typename T>
146 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
150 template <
typename T>
153 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
157 template <
typename E>
160 vectorAssignVector<ScalarAssignment>(*
this, e);
164 template <
typename E>
167 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
171 template <
typename E>
174 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
194 template <
typename V>
206 typedef typename std::conditional<std::is_const<V>::value,
207 typename V::ConstReference,
209 typedef typename std::conditional<std::is_const<V>::value,
210 typename V::ConstClosureType,
221 return data(slice(i));
226 return data(slice(i));
231 return data[slice(i)];
236 return data[slice(i)];
275 template <
typename E>
282 template <
typename E>
289 template <
typename E>
296 template <
typename T>
299 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
303 template <
typename T>
306 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
310 template <
typename E>
313 vectorAssignVector<ScalarAssignment>(*
this, e);
317 template <
typename E>
320 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
324 template <
typename E>
327 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
347 template <
typename V>
351 template <
typename V>
355 template <
typename V>
359 template <
typename V>
363 template <
typename E>
370 template <
typename E>
377 template <
typename E>
388 template <
typename E>
399 template <
typename E>
406 template <
typename E>
413 template <
typename E>
425 template <
typename E>
439 #endif // CDPL_MATH_VECTORPROXY_HPP
Definition: TypeTraits.hpp:179
Reference operator[](SizeType i)
Definition: VectorProxy.hpp:229
std::enable_if< IsScalar< T >::value, VectorSlice >::type & operator*=(const T &t)
Definition: VectorProxy.hpp:297
void vectorSwap(V &v, VectorExpression< E > &e)
Definition: VectorAssignment.hpp:72
VectorSlice & operator=(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:276
Implementation of vector assignment routines.
bool isEmpty() const
Definition: VectorProxy.hpp:254
V::ConstReference ConstReference
Definition: VectorProxy.hpp:57
friend void swap(VectorRange &r1, VectorRange &r2)
Definition: VectorProxy.hpp:184
VectorSlice & minusAssign(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:325
SelfType ClosureType
Definition: VectorProxy.hpp:213
std::enable_if< IsScalar< T >::value, VectorSlice >::type & operator/=(const T &t)
Definition: VectorProxy.hpp:304
ConstReference operator()(SizeType i) const
Definition: VectorProxy.hpp:224
Definition: Expression.hpp:54
std::conditional< std::is_const< V >::value, typename V::ConstClosureType, typename V::ClosureType >::type VectorClosureType
Definition: VectorProxy.hpp:211
Reference operator()(SizeType i)
Definition: VectorProxy.hpp:71
bool isEmpty() const
Definition: VectorProxy.hpp:101
VectorRange & operator-=(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:137
Range< SizeType > RangeType
Definition: VectorProxy.hpp:66
VectorSlice & operator-=(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:290
V VectorType
Definition: VectorProxy.hpp:53
VectorSlice & operator+=(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:283
V::SizeType SizeType
Definition: VectorProxy.hpp:54
Reference operator[](SizeType i)
Definition: VectorProxy.hpp:81
MatrixSlice< E > slice(MatrixExpression< E > &e, const typename MatrixSlice< E >::SliceType &s1, const typename MatrixSlice< E >::SliceType &s2)
Definition: MatrixProxy.hpp:788
VectorSlice & plusAssign(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:318
bool isEmpty() const
Definition: Slice.hpp:83
VectorRange & assign(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:158
V::ConstReference ConstReference
Definition: VectorProxy.hpp:205
V::ValueType ValueType
Definition: VectorProxy.hpp:56
V VectorType
Definition: VectorProxy.hpp:201
const SelfType ConstClosureType
Definition: VectorProxy.hpp:64
SizeType SizeType
Definition: Range.hpp:50
VectorRange & operator=(const VectorRange &r)
Definition: VectorProxy.hpp:116
std::conditional< std::is_const< V >::value, typename V::ConstClosureType, typename V::ClosureType >::type VectorClosureType
Definition: VectorProxy.hpp:63
Definition of type traits.
Reference operator()(SizeType i)
Definition: VectorProxy.hpp:219
std::conditional< std::is_const< V >::value, typename V::ConstReference, typename V::Reference >::type Reference
Definition: VectorProxy.hpp:208
VectorRange & operator+=(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:130
VectorRange & minusAssign(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:172
Definition of various functors.
bool isEmpty() const
Definition: Range.hpp:82
VectorRange(VectorType &v, const RangeType &r)
Definition: VectorProxy.hpp:68
V::DifferenceType DifferenceType
Definition: VectorProxy.hpp:55
VectorClosureType & getData()
Definition: VectorProxy.hpp:259
SizeType SizeType
Definition: Slice.hpp:50
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
SizeType getStart() const
Definition: VectorProxy.hpp:91
const unsigned int s
Specifies that the stereocenter has s configuration.
Definition: CIPDescriptor.hpp:81
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
ConstReference operator()(SizeType i) const
Definition: VectorProxy.hpp:76
Slice< SizeType, DifferenceType > SliceType
Definition: VectorProxy.hpp:214
DifferenceType getStride() const
Definition: Slice.hpp:73
VectorSlice(VectorType &v, const SliceType &s)
Definition: VectorProxy.hpp:216
SizeType getStart() const
Definition: Slice.hpp:68
const VectorClosureType & getData() const
Definition: VectorProxy.hpp:111
The namespace of the Chemical Data Processing Library.
SizeType getStart() const
Definition: VectorProxy.hpp:239
DifferenceType DifferenceType
Definition: Slice.hpp:51
VectorRange & operator=(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:123
VectorClosureType & getData()
Definition: VectorProxy.hpp:106
V::SizeType SizeType
Definition: VectorProxy.hpp:202
V::ValueType ValueType
Definition: VectorProxy.hpp:204
VectorSlice & assign(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:311
const SelfType ConstClosureType
Definition: VectorProxy.hpp:212
SelfType ClosureType
Definition: VectorProxy.hpp:65
std::conditional< std::is_const< V >::value, typename V::ConstReference, typename V::Reference >::type Reference
Definition: VectorProxy.hpp:60
ConstReference operator[](SizeType i) const
Definition: VectorProxy.hpp:86
void swap(VectorSlice &s)
Definition: VectorProxy.hpp:331
friend void swap(VectorSlice &s1, VectorSlice &s2)
Definition: VectorProxy.hpp:337
V::DifferenceType DifferenceType
Definition: VectorProxy.hpp:203
VectorRange & plusAssign(const VectorExpression< E > &e)
Definition: VectorProxy.hpp:165
ConstReference operator[](SizeType i) const
Definition: VectorProxy.hpp:234
std::enable_if< IsScalar< T >::value, VectorRange >::type & operator/=(const T &t)
Definition: VectorProxy.hpp:151
SizeType getSize() const
Definition: VectorProxy.hpp:96
void swap(VectorRange &r)
Definition: VectorProxy.hpp:178
Definition: VectorProxy.hpp:48
SizeType getSize() const
Definition: Range.hpp:77
Definition of a data type for describing index slices.
Definition of basic expression types.
MatrixRange< E > range(MatrixExpression< E > &e, const typename MatrixRange< E >::RangeType &r1, const typename MatrixRange< E >::RangeType &r2)
Definition: MatrixProxy.hpp:744
DifferenceType getStride() const
Definition: VectorProxy.hpp:244
VectorSlice & operator=(const VectorSlice &s)
Definition: VectorProxy.hpp:269
Definition of a data type for describing index ranges.
const VectorClosureType & getData() const
Definition: VectorProxy.hpp:264
SizeType getSize() const
Definition: Slice.hpp:78
Definition: VectorProxy.hpp:196
V::VectorTemporaryType Type
Definition: TypeTraits.hpp:181
SizeType getStart() const
Definition: Range.hpp:67
std::enable_if< IsScalar< T >::value, VectorRange >::type & operator*=(const T &t)
Definition: VectorProxy.hpp:144
SizeType getSize() const
Definition: VectorProxy.hpp:249