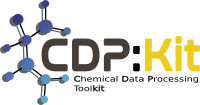 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_VECTORITERATOR_HPP
28 #define CDPL_MATH_VECTORITERATOR_HPP
47 typedef typename E::SizeType SizeType;
48 typedef typename E::Reference Reference;
61 return (&vec.get() == &accessor.vec.get());
65 std::reference_wrapper<VectorType> vec;
73 typedef typename E::SizeType SizeType;
74 typedef typename E::ConstReference Reference;
90 return (&vec.get() == &accessor.vec.get());
95 vec = std::reference_wrapper<const VectorType>(accessor.vec);
100 std::reference_wrapper<const VectorType> vec;
103 template <
typename E>
119 template <
typename E>
135 template <
typename E>
141 template <
typename E>
147 template <
typename E>
153 template <
typename E>
161 #endif // CDPL_MATH_VECTORITERATOR_HPP
const unsigned int E
Specifies that the stereocenter has E configuration.
Definition: CIPDescriptor.hpp:96
bool operator==(const VectorElementAccessor &accessor) const
Definition: VectorIterator.hpp:59
Definition of the class CDPL::Util::IndexedElementIterator.
Definition: Expression.hpp:54
Reference operator()(SizeType i) const
Definition: VectorIterator.hpp:54
E::SizeType SizeType
Definition: VectorIterator.hpp:108
VectorElementAccessor & operator=(const VectorElementAccessor< E > &accessor)
Definition: VectorIterator.hpp:93
VectorElementAccessor(const VectorElementAccessor< E > &accessor)
Definition: VectorIterator.hpp:77
Definition: VectorIterator.hpp:105
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
VectorElementAccessor(const VectorType &e)
Definition: VectorIterator.hpp:80
VectorElementAccessor(VectorType &e)
Definition: VectorIterator.hpp:51
E::SizeType SizeType
Definition: VectorIterator.hpp:124
Definition: VectorIterator.hpp:44
E::ValueType ValueType
Definition: VectorIterator.hpp:125
bool operator==(const VectorElementAccessor &accessor) const
Definition: VectorIterator.hpp:88
Util::IndexedElementIterator< ValueType, AccessorType, SizeType > IteratorType
Definition: VectorIterator.hpp:111
E::ValueType ValueType
Definition: VectorIterator.hpp:109
Reference operator()(SizeType i) const
Definition: VectorIterator.hpp:83
static IteratorType makeIterator(VectorType &e, SizeType idx)
Definition: VectorIterator.hpp:113
The namespace of the Chemical Data Processing Library.
Definition: VectorIterator.hpp:70
Util::IndexedElementIterator< const ValueType, AccessorType, SizeType > IteratorType
Definition: VectorIterator.hpp:127
static IteratorType makeIterator(const VectorType &e, SizeType idx)
Definition: VectorIterator.hpp:129
E VectorType
Definition: VectorIterator.hpp:107
VectorIteratorTraits< E >::IteratorType vectorBegin(VectorExpression< E > &e)
Definition: VectorIterator.hpp:136
E VectorType
Definition: VectorIterator.hpp:123
Definition of basic expression types.
VectorElementAccessor< E > AccessorType
Definition: VectorIterator.hpp:110
VectorIteratorTraits< E >::IteratorType vectorEnd(VectorExpression< E > &e)
Definition: VectorIterator.hpp:142
VectorElementAccessor< const E > AccessorType
Definition: VectorIterator.hpp:126