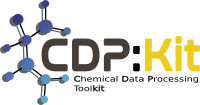 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_VECTORASSIGNMENT_HPP
28 #define CDPL_MATH_VECTORASSIGNMENT_HPP
44 class VectorExpression;
46 template <
template <
typename T1,
typename T2>
class F,
typename V,
typename E>
53 typedef F<typename V::Reference, typename E::ValueType> FunctorType;
55 for (SizeType i = 0; i < size; i++)
56 FunctorType::apply(v(i), e()(i));
59 template <
template <
typename T1,
typename T2>
class F,
typename V,
typename T>
62 typedef F<typename V::Reference, T> FunctorType;
63 typedef typename V::SizeType SizeType;
65 SizeType size = v.getSize();
67 for (SizeType i = 0; i < size; i++)
68 FunctorType::apply(v(i), t);
71 template <
typename V,
typename E>
78 for (SizeType i = 0; i < size; i++)
79 std::swap(v(i), e()(i));
84 #endif // CDPL_MATH_VECTORASSIGNMENT_HPP
#define CDPL_MATH_CHECK_SIZE_EQUALITY(size1, size2, e)
Definition: Check.hpp:62
void vectorSwap(V &v, VectorExpression< E > &e)
Definition: VectorAssignment.hpp:72
const unsigned int E
Specifies that the stereocenter has E configuration.
Definition: CIPDescriptor.hpp:96
std::common_type< T1, T2 >::type Type
Definition: CommonType.hpp:43
Definition: Expression.hpp:54
void vectorAssignVector(V &v, const VectorExpression< E > &e)
Definition: VectorAssignment.hpp:47
Thrown to indicate that the size of a (multidimensional) array is not correct.
Definition: Base/Exceptions.hpp:133
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Definition of exception classes.
const unsigned int V
Specifies Vanadium.
Definition: AtomType.hpp:177
void vectorAssignScalar(V &v, const T &t)
Definition: VectorAssignment.hpp:60
The namespace of the Chemical Data Processing Library.
Definition of various preprocessor macros for error checking.
const unsigned int F
Specifies Fluorine.
Definition: AtomType.hpp:107