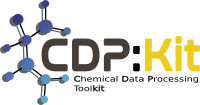 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_VECTORARRAYALIGNMENTCALCULATOR_HPP
28 #define CDPL_MATH_VECTORARRAYALIGNMENTCALCULATOR_HPP
43 template <
typename VA,
typename V =
typename VA::ElementType,
typename T =
typename V::ValueType>
53 template <
typename VE>
55 bool do_center =
true, std::size_t max_svd_iter = 0)
58 return kabschAlgo.
align(MatrixVectorArrayAdapter(points), MatrixVectorArrayAdapter(ref_points),
59 weights, do_center, max_svd_iter);
63 bool do_center =
true, std::size_t max_svd_iter = 0)
66 return kabschAlgo.
align(MatrixVectorArrayAdapter(points), MatrixVectorArrayAdapter(ref_points),
67 do_center, max_svd_iter);
76 class MatrixVectorArrayAdapter :
public MatrixExpression<MatrixVectorArrayAdapter>
79 typedef MatrixVectorArrayAdapter SelfType;
85 typedef typename VectorArrayType::SizeType SizeType;
86 typedef std::ptrdiff_t DifferenceType;
87 typedef SelfType ClosureType;
88 typedef const SelfType ConstClosureType;
93 Reference operator()(SizeType i, SizeType j)
95 return data.getData()[j].getData()[i];
98 ConstReference operator()(SizeType i, SizeType j)
const
103 SizeType getSize1()
const
105 return VectorType::Size;
108 SizeType getSize2()
const
110 return data.getSize();
113 SizeType getMaxSize()
const
115 return data.getMaxSize();
118 SizeType getMaxSize1()
const
120 return VectorType::Size;
123 SizeType getMaxSize2()
const
125 return data.getMaxSize();
130 return data.isEmpty();
143 MatrixVectorArrayAdapter& operator=(
const MatrixVectorArrayAdapter& a)
145 data.operator=(a.data);
149 void swap(MatrixVectorArrayAdapter& a)
154 friend void swap(MatrixVectorArrayAdapter& a1, MatrixVectorArrayAdapter& a2)
163 KabschAlgorithm<ValueType> kabschAlgo;
168 #endif // CDPL_MATH_VECTORARRAYALIGNMENTCALCULATOR_HPP
KabschAlgorithm< ValueType >::MatrixType MatrixType
Definition: VectorArrayAlignmentCalculator.hpp:51
Definition: Expression.hpp:54
bool calculate(const VectorArrayType &points, const VectorArrayType &ref_points, const VectorExpression< VE > &weights, bool do_center=true, std::size_t max_svd_iter=0)
Definition: VectorArrayAlignmentCalculator.hpp:54
Definition of various vector expression types and operations.
V VectorType
Definition: VectorArrayAlignmentCalculator.hpp:49
Definition: VectorArrayAlignmentCalculator.hpp:45
Definition: Expression.hpp:76
const MatrixType & getTransform() const
Definition: VectorArrayAlignmentCalculator.hpp:70
T ValueType
Definition: VectorArrayAlignmentCalculator.hpp:50
Definition of various matrix expression types and operations.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
bool align(const MatrixExpression< M1 > &points, const MatrixExpression< M2 > &ref_points, const VectorExpression< V > &weights, bool do_center=true, std::size_t max_svd_iter=0)
Computes the rigid body transformation that aligns a set of -dimensional points points with a corres...
Definition: KabschAlgorithm.hpp:86
Implementation of the Kabsch algorithm [KABA].
Definition: KabschAlgorithm.hpp:62
Implementation of the Kabsch algorithm.
The namespace of the Chemical Data Processing Library.
bool calculate(const VectorArrayType &points, const VectorArrayType &ref_points, bool do_center=true, std::size_t max_svd_iter=0)
Definition: VectorArrayAlignmentCalculator.hpp:62
VA VectorArrayType
Definition: VectorArrayAlignmentCalculator.hpp:48
const MatrixType & getTransform() const
Definition: KabschAlgorithm.hpp:199