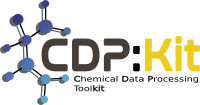 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_VECTORADAPTER_HPP
28 #define CDPL_MATH_VECTORADAPTER_HPP
30 #include <type_traits>
57 typedef typename std::conditional<std::is_const<V>::value,
58 typename V::ConstReference,
60 typedef typename std::conditional<std::is_const<V>::value,
61 typename V::ConstClosureType,
67 data(v), extElem(1) {}
71 if (i == data.getSize())
79 if (i == data.getSize())
87 if (i == data.getSize())
95 if (i == data.getSize())
103 return (data.getSize() +
SizeType(1));
127 template <
typename E>
134 template <
typename E>
141 template <
typename E>
148 template <
typename T>
151 vectorAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
155 template <
typename T>
158 vectorAssignScalar<ScalarDivisionAssignment>(*
this, t);
162 template <
typename E>
165 vectorAssignVector<ScalarAssignment>(*
this, e);
169 template <
typename E>
172 vectorAssignVector<ScalarAdditionAssignment>(*
this, e);
176 template <
typename E>
179 vectorAssignVector<ScalarSubtractionAssignment>(*
this, e);
199 template <
typename V>
209 typedef typename std::conditional<std::is_const<V>::value,
210 typename V::ConstReference,
212 typedef typename std::conditional<std::is_const<V>::value,
213 typename V::ConstClosureType,
277 template <
typename E>
284 template <
typename T>
295 template <
typename E>
302 template <
typename T>
310 template <
typename E>
317 template <
typename T>
325 template <
typename E>
332 template <
typename T>
336 quaternionAssignScalar<ScalarMultiplicationAssignment>(*
this, t);
340 template <
typename E>
347 template <
typename T>
351 quaternionAssignScalar<ScalarDivisionAssignment>(*
this, t);
355 template <
typename E>
358 quaternionAssignQuaternion<ScalarAssignment>(*
this, e);
362 template <
typename E>
365 quaternionAssignQuaternion<ScalarAdditionAssignment>(*
this, e);
369 template <
typename E>
372 quaternionAssignQuaternion<ScalarSubtractionAssignment>(*
this, e);
400 template <
typename T>
402 template <
typename V>
409 template <
typename V>
416 template <
typename V>
420 template <
typename V>
424 template <
typename E>
431 template <
typename E>
432 VectorQuaternionAdapter<const E>
438 template <
typename E>
439 HomogenousCoordsAdapter<E>
445 template <
typename E>
446 HomogenousCoordsAdapter<const E>
454 #endif // CDPL_MATH_VECTORADAPTER_HPP
void swap(VectorQuaternionAdapter &a)
Definition: VectorAdapter.hpp:376
Quaternion< typename V::ValueType > Type
Definition: VectorAdapter.hpp:406
HomogenousCoordsAdapter & operator+=(const VectorExpression< E > &e)
Definition: VectorAdapter.hpp:135
HomogenousCoordsAdapter & minusAssign(const VectorExpression< E > &e)
Definition: VectorAdapter.hpp:177
Definition: TypeTraits.hpp:179
const SelfType ConstClosureType
Definition: VectorAdapter.hpp:215
void vectorSwap(V &v, VectorExpression< E > &e)
Definition: VectorAssignment.hpp:72
Implementation of vector assignment routines.
HomogenousCoordsAdapter & operator=(const HomogenousCoordsAdapter &va)
Definition: VectorAdapter.hpp:121
std::enable_if< IsScalar< T >::value, VectorQuaternionAdapter >::type & operator*=(const T &t)
Definition: VectorAdapter.hpp:334
VectorClosureType & getData()
Definition: VectorAdapter.hpp:111
Reference operator()(SizeType i)
Definition: VectorAdapter.hpp:69
Q::QuaternionTemporaryType Type
Definition: TypeTraits.hpp:195
std::enable_if< IsScalar< T >::value, VectorQuaternionAdapter >::type & operator=(const T &t)
Definition: VectorAdapter.hpp:286
Reference getC3()
Definition: VectorAdapter.hpp:231
Definition: Expression.hpp:54
std::conditional< std::is_const< V >::value, typename V::ConstReference, typename V::Reference >::type Reference
Definition: VectorAdapter.hpp:211
SelfType ClosureType
Definition: VectorAdapter.hpp:216
Definition: VectorAdapter.hpp:47
void swap(HomogenousCoordsAdapter &va)
Definition: VectorAdapter.hpp:183
std::enable_if< IsScalar< T >::value, RealQuaternion< T > >::type quat(const T &t)
Definition: Quaternion.hpp:662
Definition: VectorAdapter.hpp:201
V VectorType
Definition: VectorAdapter.hpp:206
friend void swap(HomogenousCoordsAdapter &va1, HomogenousCoordsAdapter &va2)
Definition: VectorAdapter.hpp:189
Definition: Quaternion.hpp:239
const VectorClosureType & getData() const
Definition: VectorAdapter.hpp:116
VectorQuaternionAdapter & operator+=(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:296
V::ConstReference ConstReference
Definition: VectorAdapter.hpp:56
HomogenousCoordsAdapter & operator=(const VectorExpression< E > &e)
Definition: VectorAdapter.hpp:128
VectorQuaternionAdapter & assign(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:356
V::ConstReference ConstReference
Definition: VectorAdapter.hpp:208
HomogenousCoordsAdapter & plusAssign(const VectorExpression< E > &e)
Definition: VectorAdapter.hpp:170
std::enable_if< IsScalar< T >::value, VectorQuaternionAdapter >::type & operator+=(const T &t)
Definition: VectorAdapter.hpp:304
V::DifferenceType DifferenceType
Definition: VectorAdapter.hpp:54
Definition: Expression.hpp:98
ConstReference getC2() const
Definition: VectorAdapter.hpp:246
VectorQuaternionAdapter & plusAssign(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:363
VectorQuaternionAdapter & operator-=(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:311
std::enable_if< IsScalar< T >::value, HomogenousCoordsAdapter >::type & operator/=(const T &t)
Definition: VectorAdapter.hpp:156
SizeType getSize() const
Definition: VectorAdapter.hpp:101
std::enable_if< IsScalar< T >::value, HomogenousCoordsAdapter >::type & operator*=(const T &t)
Definition: VectorAdapter.hpp:149
SelfType ClosureType
Definition: VectorAdapter.hpp:64
V::SizeType SizeType
Definition: VectorAdapter.hpp:53
std::conditional< std::is_const< V >::value, typename V::ConstReference, typename V::Reference >::type Reference
Definition: VectorAdapter.hpp:59
const VectorClosureType & getData() const
Definition: VectorAdapter.hpp:266
Definition of type traits.
ConstReference getC3() const
Definition: VectorAdapter.hpp:251
HomogenousCoordsAdapter< E > homog(VectorExpression< E > &e)
Definition: VectorAdapter.hpp:440
ConstReference operator()(SizeType i) const
Definition: VectorAdapter.hpp:77
VectorClosureType & getData()
Definition: VectorAdapter.hpp:261
void quaternionSwap(Q &q, QuaternionExpression< E > &e)
Definition: QuaternionAssignment.hpp:65
std::enable_if< IsScalar< T >::value, VectorQuaternionAdapter >::type & operator/=(const T &t)
Definition: VectorAdapter.hpp:349
Definition of various functors.
Definition: TypeTraits.hpp:193
Reference getC4()
Definition: VectorAdapter.hpp:236
VectorQuaternionAdapter & operator=(const VectorQuaternionAdapter &a)
Definition: VectorAdapter.hpp:271
Implementation of quaternion assignment routines.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
VectorQuaternionAdapter(VectorType &v)
Definition: VectorAdapter.hpp:218
Reference getC1()
Definition: VectorAdapter.hpp:221
const SelfType ConstClosureType
Definition: VectorAdapter.hpp:63
V::ValueType ValueType
Definition: VectorAdapter.hpp:55
The namespace of the Chemical Data Processing Library.
VectorQuaternionAdapter & operator=(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:278
void set(const ValueType &c1=ValueType(), const ValueType &c2=ValueType(), const ValueType &c3=ValueType(), const ValueType &c4=ValueType())
Definition: VectorAdapter.hpp:387
bool isEmpty() const
Definition: VectorAdapter.hpp:106
V::ValueType ValueType
Definition: VectorAdapter.hpp:207
VectorQuaternionAdapter & operator*=(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:326
friend void swap(VectorQuaternionAdapter &a1, VectorQuaternionAdapter &a2)
Definition: VectorAdapter.hpp:382
Reference operator[](SizeType i)
Definition: VectorAdapter.hpp:85
std::conditional< std::is_const< V >::value, typename V::ConstClosureType, typename V::ClosureType >::type VectorClosureType
Definition: VectorAdapter.hpp:62
ConstReference operator[](SizeType i) const
Definition: VectorAdapter.hpp:93
Reference getC2()
Definition: VectorAdapter.hpp:226
VectorQuaternionAdapter & minusAssign(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:370
HomogenousCoordsAdapter & assign(const VectorExpression< E > &e)
Definition: VectorAdapter.hpp:163
Quaternion< typename V::ValueType > Type
Definition: VectorAdapter.hpp:413
Definition of basic expression types.
std::conditional< std::is_const< V >::value, typename V::ConstClosureType, typename V::ClosureType >::type VectorClosureType
Definition: VectorAdapter.hpp:214
ConstReference getC4() const
Definition: VectorAdapter.hpp:256
ConstReference getC1() const
Definition: VectorAdapter.hpp:241
V VectorType
Definition: VectorAdapter.hpp:52
VectorQuaternionAdapter & operator/=(const QuaternionExpression< E > &e)
Definition: VectorAdapter.hpp:341
V::VectorTemporaryType Type
Definition: TypeTraits.hpp:181
HomogenousCoordsAdapter & operator-=(const VectorExpression< E > &e)
Definition: VectorAdapter.hpp:142
HomogenousCoordsAdapter(VectorType &v)
Definition: VectorAdapter.hpp:66
std::enable_if< IsScalar< T >::value, VectorQuaternionAdapter >::type & operator-=(const T &t)
Definition: VectorAdapter.hpp:319