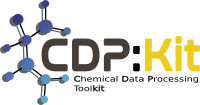 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_TYPETRAITS_HPP
28 #define CDPL_MATH_TYPETRAITS_HPP
34 #include <type_traits>
43 template <
bool Signed>
115 template <
typename T>
118 template <
typename T>
169 template <
typename T>
173 template <
typename T>
177 template <
typename V>
181 typedef typename V::VectorTemporaryType
Type;
184 template <
typename M>
188 typedef typename M::MatrixTemporaryType
Type;
191 template <
typename Q>
195 typedef typename Q::QuaternionTemporaryType
Type;
198 template <
typename G>
202 typedef typename G::GridTemporaryType
Type;
205 template <
typename T>
209 template <
typename T>
210 struct IsScalar<std::complex<T> > :
public std::is_arithmetic<T>
215 #endif // CDPL_MATH_TYPETRAITS_HPP
Definition: TypeTraits.hpp:171
Definition: TypeTraits.hpp:179
T ValueType
Definition: TypeTraits.hpp:69
static RealType norm2(ConstReference t)
Definition: TypeTraits.hpp:104
Definition: TypeTraits.hpp:67
static RealType abs(ConstReference t)
Definition: TypeTraits.hpp:89
Q::QuaternionTemporaryType Type
Definition: TypeTraits.hpp:195
static RealType imag(ConstReference)
Definition: TypeTraits.hpp:79
GridUnaryTraits< E, ScalarReal< typename E::ValueType > >::ResultType real(const GridExpression< E > &e)
Definition: GridExpression.hpp:378
Definition: TypeTraits.hpp:200
ScalarTraits< T > SelfType
Definition: TypeTraits.hpp:72
static RealType conj(ConstReference t)
Definition: TypeTraits.hpp:84
static const T & abs(const T &t)
Definition: TypeTraits.hpp:59
static RealType norm1(ConstReference t)
Definition: TypeTraits.hpp:99
M::MatrixTemporaryType Type
Definition: TypeTraits.hpp:188
GridUnaryTraits< E, ScalarConjugation< typename E::ValueType > >::ResultType conj(const GridExpression< E > &e)
Definition: GridExpression.hpp:360
Definition: TypeTraits.hpp:186
Definition: TypeTraits.hpp:193
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
static RealType normInf(ConstReference t)
Definition: TypeTraits.hpp:109
The namespace of the Chemical Data Processing Library.
static ValueType sqrt(ConstReference t)
Definition: TypeTraits.hpp:94
static RealType real(ConstReference t)
Definition: TypeTraits.hpp:74
GridUnaryTraits< E, ScalarImaginary< typename E::ValueType > >::ResultType imag(const GridExpression< E > &e)
Definition: GridExpression.hpp:387
Definition: TypeTraits.hpp:207
G::GridTemporaryType Type
Definition: TypeTraits.hpp:202
Definition: TypeTraits.hpp:45
const T & ConstReference
Definition: TypeTraits.hpp:71
T RealType
Definition: TypeTraits.hpp:70
V::VectorTemporaryType Type
Definition: TypeTraits.hpp:181
static T abs(const T &t)
Definition: TypeTraits.hpp:48