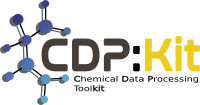 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_TORSIONRULE_HPP
30 #define CDPL_CONFGEN_TORSIONRULE_HPP
53 typedef std::vector<AngleEntry> AngleEntryList;
60 AngleEntry(
double ang,
double tol1 = 0.0,
double tol2 = 0.0,
double score = 0.0):
61 angle(ang), tolerance1(tol1), tolerance2(tol2), score(score) {}
103 void addAngle(
double angle,
double tol1 = 0.0,
double tol2 = 0.0,
double score = 0.0);
134 void checkAngleIndex(std::size_t idx,
bool it)
const;
136 std::string matchPatternStr;
138 AngleEntryList angles;
143 #endif // CDPL_CONFGEN_TORSIONRULE_HPP
ConstAngleEntryIterator getAnglesBegin() const
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
void addAngle(double angle, double tol1=0.0, double tol2=0.0, double score=0.0)
const std::string & getMatchPatternString() const
void setMatchPatternString(const std::string &ptn_str)
void setMatchPattern(const Chem::MolecularGraph::SharedPointer &ptn)
double getTolerance2() const
Definition: TorsionRule.hpp:73
Definition: TorsionRule.hpp:47
double getTolerance1() const
Definition: TorsionRule.hpp:68
AngleEntryIterator getAnglesEnd()
double getScore() const
Definition: TorsionRule.hpp:78
ConstAngleEntryIterator begin() const
const Chem::MolecularGraph::SharedPointer & getMatchPattern() const
std::size_t getNumAngles() const
Definition: TorsionRule.hpp:57
void removeAngle(const AngleEntryIterator &it)
ConstAngleEntryIterator getAnglesEnd() const
void swap(TorsionRule &rule)
void addAngle(const AngleEntry &ang_entry)
const AngleEntry & getAngle(std::size_t idx) const
Definition of the preprocessor macro CDPL_CONFGEN_API.
Definition of the class CDPL::Chem::MolecularGraph.
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
void removeAngle(std::size_t idx)
AngleEntryIterator getAnglesBegin()
The namespace of the Chemical Data Processing Library.
AngleEntryIterator begin()
double getAngle() const
Definition: TorsionRule.hpp:63
ConstAngleEntryIterator end() const
AngleEntry(double ang, double tol1=0.0, double tol2=0.0, double score=0.0)
Definition: TorsionRule.hpp:60
AngleEntryList::const_iterator ConstAngleEntryIterator
Definition: TorsionRule.hpp:91
AngleEntryList::iterator AngleEntryIterator
Definition: TorsionRule.hpp:90