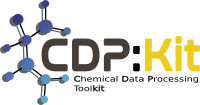 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_TORSIONRULEMATCHER_HPP
30 #define CDPL_CONFGEN_TORSIONRULEMATCHER_HPP
49 typedef std::vector<TorsionRuleMatch> RuleMatchList;
125 bool uniqueMappingsOnly;
126 bool stopAtFirstRule;
127 RuleMatchList matches;
132 #endif // CDPL_CONFGEN_TORSIONRULEMATCHER_HPP
void findAllRuleMappings(bool all)
Bond.
Definition: Bond.hpp:50
SubstructureSearch.
Definition: SubstructureSearch.hpp:64
bool findAllRuleMappings() const
Definition: TorsionRuleMatcher.hpp:47
Definition: TorsionRule.hpp:47
TorsionRuleMatcher(const TorsionLibrary::SharedPointer &lib)
bool findMatches(const Chem::Bond &bond, const Chem::MolecularGraph &molgraph, bool append=false)
MolecularGraph.
Definition: MolecularGraph.hpp:52
std::shared_ptr< TorsionLibrary > SharedPointer
Definition: TorsionLibrary.hpp:49
void stopAtFirstMatchingRule(bool stop)
bool stopAtFirstMatchingRule() const
Definition: TorsionRuleMatch.hpp:55
void findUniqueMappingsOnly(bool unique)
RuleMatchList::const_iterator ConstMatchIterator
Definition: TorsionRuleMatcher.hpp:52
Definition of the preprocessor macro CDPL_CONFGEN_API.
Definition of the class CDPL::ConfGen::TorsionRuleMatch.
ConstMatchIterator begin() const
Returns a constant iterator pointing to the beginning of the stored torsion rule matches.
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition: TorsionCategory.hpp:48
std::size_t getNumMatches() const
Returns the number of stored torsion rule matches found by calls to findMatches().
The namespace of the Chemical Data Processing Library.
ConstMatchIterator getMatchesEnd() const
Returns a constant iterator pointing to the end of the stored torsion rule matches.
A data structure for the common storage of related atom to atom and bond to bond mappings.
Definition: AtomBondMapping.hpp:55
Definition of the class CDPL::Chem::SubstructureSearch.
ConstMatchIterator end() const
Returns a constant iterator pointing to the end of the stored torsion rule matches.
Definition of the class CDPL::ConfGen::TorsionLibrary.
void setTorsionLibrary(const TorsionLibrary::SharedPointer &lib)
bool findUniqueMappingsOnly() const
ConstMatchIterator getMatchesBegin() const
Returns a constant iterator pointing to the beginning of the stored torsion rule matches.
const TorsionLibrary::SharedPointer & getTorsionLibrary() const
const TorsionRuleMatch & getMatch(std::size_t idx) const
Returns a const reference to the stored torsion rule match object at index idx.