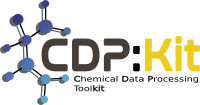 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CONFGEN_TORSIONCATEGORY_HPP
30 #define CDPL_CONFGEN_TORSIONCATEGORY_HPP
50 typedef std::vector<TorsionCategory> CategoryList;
51 typedef std::vector<TorsionRule> RuleList;
132 void checkCategoryIndex(std::size_t idx,
bool it)
const;
134 void checkRuleIndex(std::size_t idx,
bool it)
const;
137 std::string matchPatternStr;
139 unsigned int bondAtom1Type;
140 unsigned int bondAtom2Type;
142 CategoryList categories;
147 #endif // CDPL_CONFGEN_TORSIONCATEGORY_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
CategoryIterator getCategoriesEnd()
const std::string & getName() const
ConstRuleIterator getRulesEnd() const
std::size_t getNumRules(bool recursive=false) const
RuleList::iterator RuleIterator
Definition: TorsionCategory.hpp:56
unsigned int getBondAtom2Type() const
Definition of the class CDPL::ConfGen::TorsionRule.
void removeRule(std::size_t idx)
TorsionCategory & addCategory()
void setBondAtom2Type(unsigned int type)
virtual ~TorsionCategory()
Definition: TorsionCategory.hpp:61
Definition: TorsionRule.hpp:47
TorsionCategory & addCategory(const TorsionCategory &cat)
void removeRule(const RuleIterator &it)
CategoryList::const_iterator ConstCategoryIterator
Definition: TorsionCategory.hpp:55
void setMatchPattern(const Chem::MolecularGraph::SharedPointer &ptn)
void setName(const std::string &name)
CategoryList::iterator CategoryIterator
Definition: TorsionCategory.hpp:54
void setMatchPatternString(const std::string &ptn_str)
const Chem::MolecularGraph::SharedPointer & getMatchPattern() const
RuleIterator getRulesEnd()
void removeCategory(std::size_t idx)
const TorsionRule & getRule(std::size_t idx) const
std::size_t getNumCategories(bool recursive=false) const
Definition of the preprocessor macro CDPL_CONFGEN_API.
Definition of the class CDPL::Chem::MolecularGraph.
#define CDPL_CONFGEN_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
ConstCategoryIterator getCategoriesEnd() const
Definition: TorsionCategory.hpp:48
CategoryIterator getCategoriesBegin()
The namespace of the Chemical Data Processing Library.
void setBondAtom1Type(unsigned int type)
void swap(TorsionCategory &cat)
RuleIterator getRulesBegin()
ConstCategoryIterator getCategoriesBegin() const
TorsionRule & addRule(const TorsionRule &rule)
const std::string & getMatchPatternString() const
RuleList::const_iterator ConstRuleIterator
Definition: TorsionCategory.hpp:57
TorsionRule & getRule(std::size_t idx)
void removeCategory(const CategoryIterator &it)
const TorsionCategory & getCategory(std::size_t idx) const
unsigned int getBondAtom1Type() const
ConstRuleIterator getRulesBegin() const
TorsionCategory & getCategory(std::size_t idx)