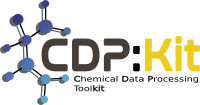 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_TAUTOMERGENERATOR_HPP
30 #define CDPL_CHEM_TAUTOMERGENERATOR_HPP
35 #include <unordered_set>
130 typedef MoleculeCache::SharedObjectPointer MoleculePtr;
133 void initHashCalculator();
141 bool addNewTautomer(
const MoleculePtr& mol_ptr);
142 bool outputTautomer(
const MoleculePtr& mol_ptr);
144 std::uint64_t calcConTabHashCode(
const MolecularGraph& molgraph,
bool arom_bonds);
150 typedef std::array<std::size_t, 3> BondDescriptor;
151 typedef std::vector<MoleculePtr> MoleculeList;
152 typedef std::vector<TautomerizationRule::SharedPointer> TautRuleList;
153 typedef std::vector<BondDescriptor> BondDescrArray;
154 typedef std::vector<std::size_t> SizeTArray;
155 typedef std::unordered_set<std::uint64_t> HashCodeSet;
156 typedef std::array<std::size_t, 6> StereoCenter;
157 typedef std::vector<StereoCenter> StereoCenterList;
164 bool remResDuplicates;
166 TautRuleList tautRules;
167 MoleculeList currGeneration;
168 MoleculeList nextGeneration;
169 StereoCenterList atomStereoCenters;
170 StereoCenterList bondStereoCenters;
171 HashCodeSet intermTautHashCodes;
172 HashCodeSet outputTautHashCodes;
176 BondDescrArray tautomerBonds;
183 #endif // CDPL_CHEM_TAUTOMERGENERATOR_HPP
void removeResonanceDuplicates(bool remove)
std::shared_ptr< TautomerizationRule > SharedPointer
Definition: TautomerizationRule.hpp:53
Definition of the class CDPL::Util::ObjectPool.
void regardStereochemistry(bool regard)
Definition of the class CDPL::Chem::TautomerizationRule.
Definition of the preprocessor macro CDPL_CHEM_API.
const CallbackFunction & getCallbackFunction() const
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
bool resonanceDuplicatesRemoved() const
TautomerGenerator.
Definition: TautomerGenerator.hpp:59
Mode
Definition: TautomerGenerator.hpp:63
bool isotopesRegarded() const
Definition of the class CDPL::Chem::HashCodeCalculator.
void addTautomerizationRule(const TautomerizationRule::SharedPointer &rule)
TautomerGenerator & operator=(const TautomerGenerator &gen)
TautomerGenerator()
Constructs the TautomerGenerator instance.
TautomerGenerator(const TautomerGenerator &gen)
std::size_t getNumTautomerizationRules() const
MolecularGraph.
Definition: MolecularGraph.hpp:52
std::function< bool(MolecularGraph &)> CallbackFunction
Definition: TautomerGenerator.hpp:72
void removeTautomerizationRule(std::size_t idx)
bool stereochemistryRegarded() const
@ TOPOLOGICALLY_UNIQUE
Definition: TautomerGenerator.hpp:65
std::shared_ptr< TautomerGenerator > SharedPointer
Definition: TautomerGenerator.hpp:70
const TautomerizationRule::SharedPointer & getTautomerizationRule(std::size_t idx) const
@ GEOMETRICALLY_UNIQUE
Definition: TautomerGenerator.hpp:66
The namespace of the Chemical Data Processing Library.
std::function< void(MolecularGraph &)> CustomSetupFunction
Definition: TautomerGenerator.hpp:73
CIPConfigurationLabeler.
Definition: CIPConfigurationLabeler.hpp:55
void setCallbackFunction(const CallbackFunction &func)
void generate(const MolecularGraph &molgraph)
Generates all unique tautomers of the molecular graph molgraph.
Definition of the class CDPL::Chem::CIPConfigurationLabeler.
Definition of the class CDPL::Chem::AromaticSubstructure.
HashCodeCalculator.
Definition: HashCodeCalculator.hpp:57
void setCustomSetupFunction(const CustomSetupFunction &func)
CDPL_CHEM_API void setAromaticityFlags(MolecularGraph &molgraph, bool overwrite)
Implements the perception of aromatic atoms and bonds in a molecular graph.
Definition: AromaticSubstructure.hpp:52
virtual ~TautomerGenerator()
Definition: TautomerGenerator.hpp:82
Definition of the class CDPL::Chem::BasicMolecule.
void regardIsotopes(bool regard)