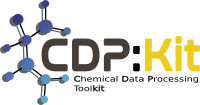 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_SYMMETRYCLASSCALCULATOR_HPP
30 #define CDPL_CHEM_SYMMETRYCLASSCALCULATOR_HPP
62 static constexpr
unsigned int DEF_ATOM_PROPERTY_FLAGS =
70 static constexpr
unsigned int DEF_BOND_PROPERTY_FLAGS =
166 void calcSVMNumbers();
171 AtomNode* allocNode(std::uint64_t class_id);
173 typedef std::vector<AtomNode*> NodeList;
181 void addNbrNode(AtomNode*);
183 void setSVMNumber(std::uint64_t);
185 void calcNextSVMNumber();
186 void updateSVMNumber();
187 void updateSVMHistory();
189 void setNextSymClassID(std::uint64_t);
193 std::size_t getSymClassID()
const;
194 void setSymClassID(std::uint64_t class_id);
209 typedef std::vector<std::uint64_t> SVMNumberList;
211 std::uint64_t symClassID;
212 std::uint64_t nextSymClassID;
213 std::uint64_t nbrSymClassIDProd;
214 std::uint64_t svmNumber;
215 std::uint64_t nextSVMNumber;
216 SVMNumberList svmNumberHistory;
223 unsigned int atomPropertyFlags;
224 unsigned int bondPropertyFlags;
228 NodeList sortedAtomNodes;
233 #endif // CDPL_CHEM_SYMMETRYCLASSCALCULATOR_HPP
Definition of the class CDPL::Util::ObjectStack.
Definition: SymmetryClassCalculator.hpp:197
const unsigned int FORMAL_CHARGE
Specifies the formal charge of an atom.
Definition: Chem/AtomPropertyFlag.hpp:73
const unsigned int AROMATICITY
Specifies the membership of a bond in aromatic rings.
Definition: BondPropertyFlag.hpp:73
Definition of the preprocessor macro CDPL_CHEM_API.
void calculate(const MolecularGraph &molgraph, Util::STArray &class_ids)
Perceives the topological symmetry classes of the atoms in the molecular graph molgraph.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
Definition: SymmetryClassCalculator.hpp:203
void includeImplicitHydrogens(bool include)
Allows to specify whether implicit hydrogen atoms shall be ignored or treated in the same way as expl...
SymmetryClassCalculator()
Constructs the SymmetryClassCalculator instance.
unsigned int getAtomPropertyFlags() const
Returns the set of atomic properties that gets considered in the perception of topological symmetry c...
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the class CDPL::Util::Array.
Definition of constants in namespace CDPL::Chem::AtomPropertyFlag.
bool operator()(const AtomNode *, const AtomNode *) const
unsigned int getBondPropertyFlags() const
Returns the set of bond properties that gets considered in the perception of topological symmetry cla...
const unsigned int ORDER
Specifies the order of a bond.
Definition: BondPropertyFlag.hpp:63
bool operator()(const AtomNode *, const AtomNode *) const
const unsigned int TYPE
Specifies the generic type or element of an atom.
Definition: Chem/AtomPropertyFlag.hpp:63
const unsigned int ISOTOPE
Specifies the isotopic mass of an atom.
Definition: Chem/AtomPropertyFlag.hpp:68
void setAtomPropertyFlags(unsigned int flags)
Allows to specify the set of atomic properties that has to be considered in the perception of topolog...
The namespace of the Chemical Data Processing Library.
Array< std::size_t > STArray
An array of unsigned integers of type std::size_t.
Definition: Array.hpp:567
bool implicitHydrogensIncluded() const
Tells whether implicit hydrogen atoms are ignored or treated in the same way as explicit ones.
SymmetryClassCalculator(const MolecularGraph &molgraph, Util::STArray &class_ids)
Constructs the SymmetryClassCalculator instance and perceives the topological symmetry classes of the...
const unsigned int AROMATICITY
Specifies the membership of an atom in aromatic rings.
Definition: Chem/AtomPropertyFlag.hpp:93
void setBondPropertyFlags(unsigned int flags)
Allows to specify the set of bond properties that has to be considered in the perception of topologic...
const unsigned int H_COUNT
Specifies the hydrogen count of an atom.
Definition: Chem/AtomPropertyFlag.hpp:78
Definition of constants in namespace CDPL::Chem::BondPropertyFlag.
SymmetryClassCalculator.
Definition: SymmetryClassCalculator.hpp:55