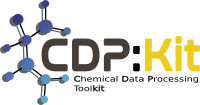 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_SYBYLATOMTYPE_HPP
30 #define CDPL_CHEM_SYBYLATOMTYPE_HPP
42 namespace SybylAtomType
53 const unsigned int C_3 = 1;
58 const unsigned int C_2 = 2;
63 const unsigned int C_1 = 3;
68 const unsigned int C_ar = 4;
78 const unsigned int N_3 = 6;
83 const unsigned int N_2 = 7;
88 const unsigned int N_1 = 8;
93 const unsigned int N_ar = 9;
98 const unsigned int N_am = 10;
108 const unsigned int N_4 = 12;
113 const unsigned int O_3 = 13;
118 const unsigned int O_2 = 14;
138 const unsigned int S_3 = 18;
143 const unsigned int S_2 = 19;
148 const unsigned int S_O = 20;
158 const unsigned int P_3 = 22;
163 const unsigned int F = 23;
168 const unsigned int H = 24;
183 const unsigned int LP = 27;
188 const unsigned int Du = 28;
198 const unsigned int Any = 30;
203 const unsigned int Hal = 31;
208 const unsigned int Het = 32;
213 const unsigned int Hev = 33;
218 const unsigned int Li = 34;
223 const unsigned int Na = 35;
228 const unsigned int Mg = 36;
233 const unsigned int Al = 37;
238 const unsigned int Si = 38;
243 const unsigned int K = 39;
248 const unsigned int Ca = 40;
263 const unsigned int Mn = 43;
268 const unsigned int Fe = 44;
278 const unsigned int Cu = 46;
283 const unsigned int Cl = 47;
288 const unsigned int Br = 48;
293 const unsigned int I = 49;
298 const unsigned int Zn = 50;
303 const unsigned int Se = 51;
308 const unsigned int Mo = 52;
313 const unsigned int Sn = 53;
323 const unsigned int B = 54;
328 const unsigned int Pt = 55;
338 #endif // CDPL_CHEM_SYBYLATOMTYPE_HPP
const unsigned int Mo
Specifies Molybdenum.
Definition: SybylAtomType.hpp:308
const unsigned int Zn
Specifies Zinc.
Definition: SybylAtomType.hpp:298
const unsigned int K
Specifies Potassium.
Definition: SybylAtomType.hpp:243
const unsigned int Cu
Specifies Copper.
Definition: SybylAtomType.hpp:278
const unsigned int P_3
Specifies sp3 Phosphorous.
Definition: SybylAtomType.hpp:158
const unsigned int Du
Specifies a dummy atom.
Definition: SybylAtomType.hpp:188
const unsigned int UNKNOWN
Atom with no matching Sybyl atom type.
Definition: SybylAtomType.hpp:48
const unsigned int Fe
Specifies Iron.
Definition: SybylAtomType.hpp:268
const unsigned int Cl
Specifies Chlorine.
Definition: SybylAtomType.hpp:283
const unsigned int Pt
Specifies Platinum.
Definition: SybylAtomType.hpp:328
const unsigned int Sn
Specifies Tin.
Definition: SybylAtomType.hpp:313
const unsigned int Any
Specifies any atom.
Definition: SybylAtomType.hpp:198
const unsigned int MAX_DEFAULT_TYPE
Marks the end of the default Sybyl atom types.
Definition: SybylAtomType.hpp:318
const unsigned int Na
Specifies Sodium.
Definition: SybylAtomType.hpp:223
const unsigned int Mg
Specifies Magnesium.
Definition: SybylAtomType.hpp:228
const unsigned int Li
Specifies Lithium.
Definition: SybylAtomType.hpp:218
const unsigned int N_pl3
Specifies a trigonal planar Nitrogen.
Definition: SybylAtomType.hpp:103
const unsigned int O_t3p
Specifies Oxygen in the Transferable Intermolecular Potential (TIP3P) water model.
Definition: SybylAtomType.hpp:133
const unsigned int Hal
Specifies any halogen.
Definition: SybylAtomType.hpp:203
const unsigned int N_3
Specifies sp3 Nitrogen.
Definition: SybylAtomType.hpp:78
const unsigned int Cr_oh
Specifies Chromium (octahedral).
Definition: SybylAtomType.hpp:258
const unsigned int O_2
Specifies sp2 Oxygen.
Definition: SybylAtomType.hpp:118
const unsigned int O_spc
Specifies Oxygen in the Single Point Charge (SPC) water model.
Definition: SybylAtomType.hpp:128
const unsigned int C_3
Specifies sp3 Carbon.
Definition: SybylAtomType.hpp:53
const unsigned int S_2
Specifies sp2 Sulfur.
Definition: SybylAtomType.hpp:143
const unsigned int C_ar
Specifies an aromatic Carbon.
Definition: SybylAtomType.hpp:68
const unsigned int H_t3p
Specifies Hydrogen in the Transferable intermolecular Potential (TIP3P) water model.
Definition: SybylAtomType.hpp:178
const unsigned int H
Specifies Hydrogen.
Definition: SybylAtomType.hpp:168
const unsigned int N_am
Specifies Nitrogen in amides.
Definition: SybylAtomType.hpp:98
const unsigned int Br
Specifies Bromine.
Definition: SybylAtomType.hpp:288
const unsigned int Co_oh
Specifies Cobalt (octahedral).
Definition: SybylAtomType.hpp:273
const unsigned int Se
Specifies Selenium.
Definition: SybylAtomType.hpp:303
const unsigned int F
Specifies Fluorine.
Definition: SybylAtomType.hpp:163
const unsigned int C_1
Specifies sp Carbon.
Definition: SybylAtomType.hpp:63
const unsigned int Hev
Specifies any heavy atom (non-Hydrogen).
Definition: SybylAtomType.hpp:213
const unsigned int B
Specifies Boron.
Definition: SybylAtomType.hpp:323
const unsigned int Het
Specifies a heteroatom (N, O, S or P).
Definition: SybylAtomType.hpp:208
const unsigned int S_O
Specifies Sulfur in sulfoxides.
Definition: SybylAtomType.hpp:148
const unsigned int Mn
Specifies Manganese.
Definition: SybylAtomType.hpp:263
const unsigned int Ca
Specifies Calcium.
Definition: SybylAtomType.hpp:248
const unsigned int S_O2
Specifies Sulfur in sulfones.
Definition: SybylAtomType.hpp:153
const unsigned int O_3
Specifies sp3 Oxygen.
Definition: SybylAtomType.hpp:113
const unsigned int H_spc
Specifies Hydrogen in the Single Point Charge (SPC) water model.
Definition: SybylAtomType.hpp:173
const unsigned int Cr_th
Specifies Chromium (tetrahedral).
Definition: SybylAtomType.hpp:253
const unsigned int Si
Specifies Silicon.
Definition: SybylAtomType.hpp:238
const unsigned int O_co2
Specifies Oxygen in carboxylate and phosphate groups.
Definition: SybylAtomType.hpp:123
The namespace of the Chemical Data Processing Library.
const unsigned int MAX_TYPE
Marks the end of all supported atom types.
Definition: SybylAtomType.hpp:333
const unsigned int N_2
Specifies sp2 Nitrogen.
Definition: SybylAtomType.hpp:83
const unsigned int C_2
Specifies sp2 Carbon.
Definition: SybylAtomType.hpp:58
const unsigned int N_ar
Specifies an aromatic Nitrogen.
Definition: SybylAtomType.hpp:93
const unsigned int Du_C
Specifies a dummy Carbon.
Definition: SybylAtomType.hpp:193
const unsigned int LP
Specifies a lone pair.
Definition: SybylAtomType.hpp:183
const unsigned int C_cat
Specifies a Carbocation (C+), used only in a guanidinium group.
Definition: SybylAtomType.hpp:73
const unsigned int I
Specifies Iodine.
Definition: SybylAtomType.hpp:293
const unsigned int S_3
Specifies sp3 Sulfur.
Definition: SybylAtomType.hpp:138
const unsigned int N_4
Specifies a positively charged sp3 Nitrogen.
Definition: SybylAtomType.hpp:108
const unsigned int Al
Specifies Aluminum.
Definition: SybylAtomType.hpp:233
const unsigned int N_1
Specifies sp Nitrogen.
Definition: SybylAtomType.hpp:88