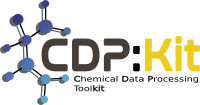 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_SUBSTRUCTUREHISTOGRAMCALCULATOR_HPP
30 #define CDPL_CHEM_SUBSTRUCTUREHISTOGRAMCALCULATOR_HPP
61 typedef std::vector<Pattern> PatternList;
74 bool all_matches =
true,
bool unique_matches =
true);
99 bool all_matches =
true,
bool unique_matches =
true);
125 template <
typename T>
131 typedef std::function<void(std::size_t)> HistoUpdateFunction;
133 template <
typename T>
134 class HistoUpdateFunctor
138 HistoUpdateFunctor(
T& histo):
141 void operator()(std::size_t
id)
150 void doCalculate(
const MolecularGraph& molgraph,
const HistoUpdateFunction& func);
152 void init(
const MolecularGraph& molgraph);
154 void processPattern(
const Pattern& ptn,
const HistoUpdateFunction& func);
155 bool processMatch(
const AtomBondMapping& mapping,
const Pattern& ptn,
const HistoUpdateFunction& func);
157 typedef std::pair<Util::BitSet, Util::BitSet> AtomBondMask;
158 typedef std::map<std::size_t, AtomBondMask> PriorityToAtomBondMaskMap;
160 const MolecularGraph* molGraph;
161 PatternList patterns;
162 SubstructureSearch substructSearch;
163 PriorityToAtomBondMaskMap matchedSubstructMasks;
164 AtomBondMask testingAtomBondMask;
171 template <
typename T>
174 doCalculate(molgraph, HistoUpdateFunctor<T>(histo));
177 #endif // CDPL_CHEM_SUBSTRUCTUREHISTOGRAMCALCULATOR_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
std::shared_ptr< SubstructureHistogramCalculator > SharedPointer
Definition: SubstructureHistogramCalculator.hpp:64
Definition of the preprocessor macro CDPL_CHEM_API.
void calculate(const MolecularGraph &molgraph, T &histo)
Definition: SubstructureHistogramCalculator.hpp:172
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
const MolecularGraph::SharedPointer & getStructure() const
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
Pattern(const MolecularGraph::SharedPointer &structure, std::size_t id, std::size_t priority=0, bool all_matches=true, bool unique_matches=true)
SubstructureHistogramCalculator(const SubstructureHistogramCalculator &gen)
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
bool processUniqueMatchesOnly() const
void removePattern(std::size_t idx)
PatternList::iterator PatternIterator
Definition: SubstructureHistogramCalculator.hpp:67
ConstPatternIterator end() const
std::size_t getNumPatterns() const
Definition of the class CDPL::Chem::MolecularGraph.
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
ConstPatternIterator begin() const
const Pattern & getPattern(std::size_t idx) const
SubstructureHistogramCalculator.
Definition: SubstructureHistogramCalculator.hpp:55
The namespace of the Chemical Data Processing Library.
void addPattern(const MolecularGraph::SharedPointer &structure, std::size_t id, std::size_t priority=0, bool all_matches=true, bool unique_matches=true)
void removePattern(const PatternIterator &ptn_it)
Definition: SubstructureHistogramCalculator.hpp:70
PatternIterator getPatternsEnd()
Definition of the class CDPL::Chem::SubstructureSearch.
bool processAllMatches() const
PatternList::const_iterator ConstPatternIterator
Definition: SubstructureHistogramCalculator.hpp:66
PatternIterator getPatternsBegin()
SubstructureHistogramCalculator & operator=(const SubstructureHistogramCalculator &gen)
std::size_t getID() const
SubstructureHistogramCalculator()
ConstPatternIterator getPatternsEnd() const
ConstPatternIterator getPatternsBegin() const
void addPattern(const Pattern &ptn)
std::size_t getPriority() const