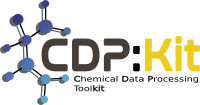 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_UTIL_STREAMDATAREADER_HPP
30 #define CDPL_UTIL_STREAMDATAREADER_HPP
71 template <
typename DataType,
typename ReaderImpl>
88 operator const void*()
const;
97 input(is), recordIndex(0), initStreamPos(is.tellg()), state(is.good()), streamScanned(false) {}
104 void scanDataStream();
106 typedef std::vector<std::istream::pos_type> RecordStreamPosTable;
109 std::size_t recordIndex;
110 std::istream::pos_type initStreamPos;
113 RecordStreamPosTable recordPositions;
121 template <
typename DataType,
typename ReaderImpl>
127 if ((state =
static_cast<ReaderImpl*
>(
this)->readData(input, obj, overwrite))) {
129 this->invokeIOCallbacks(1.0);
135 template <
typename DataType,
typename ReaderImpl>
141 return read(obj, overwrite);
144 template <
typename DataType,
typename ReaderImpl>
150 if ((state =
static_cast<ReaderImpl*
>(
this)->skipData(input))) {
152 this->invokeIOCallbacks(1.0);
158 template <
typename DataType,
typename ReaderImpl>
161 return static_cast<ReaderImpl*
>(
this)->moreData(input);
164 template <
typename DataType,
typename ReaderImpl>
170 template <
typename DataType,
typename ReaderImpl>
175 if (idx > recordPositions.size())
180 if (idx == recordPositions.size())
181 input.seekg(0, std::ios_base::end);
183 input.seekg(recordPositions[idx]);
188 template <
typename DataType,
typename ReaderImpl>
193 return recordPositions.size();
196 template <
typename DataType,
typename ReaderImpl>
199 return (state ?
this : 0);
202 template <
typename DataType,
typename ReaderImpl>
208 template <
typename DataType,
typename ReaderImpl>
214 streamScanned =
true;
216 std::size_t saved_rec_index = recordIndex;
221 input.seekg(0, std::ios_base::end);
223 std::istream::pos_type end_pos = input.tellg();
225 input.seekg(initStreamPos);
227 while (hasMoreData()) {
228 std::istream::pos_type record_pos = input.tellg();
231 if (!(state =
static_cast<ReaderImpl*
>(
this)->skipData(input)))
234 recordPositions.push_back(record_pos);
237 this->invokeIOCallbacks(record_pos /
double(end_pos));
240 this->invokeIOCallbacks(1.0);
242 if (saved_rec_index < recordPositions.size()) {
243 recordIndex = saved_rec_index;
246 input.seekg(recordPositions[recordIndex]);
250 #endif // CDPL_UTIL_STREAMDATAREADER_HPP
std::size_t getNumRecords()
Returns the total number of available data records.
Definition: StreamDataReader.hpp:189
bool operator!() const
Definition: StreamDataReader.hpp:203
Base::DataReader< DataType > & read(DataType &obj, bool overwrite=true)
Reads the data record at the current record index and stores the read data in obj.
Definition: StreamDataReader.hpp:123
Reaction DataType
The type of the read data objects.
Definition: DataReader.hpp:79
void setRecordIndex(std::size_t idx)
Sets the index of the current data record to idx.
Definition: StreamDataReader.hpp:171
Definition of the class CDPL::Base::DataReader.
std::size_t getRecordIndex() const
Definition: StreamDataReader.hpp:165
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
An interface for reading data objects of a given type from an arbitrary data source.
Definition: DataReader.hpp:73
Definition of exception classes.
The namespace of the Chemical Data Processing Library.
Base::DataReader< DataType > & skip()
Skips the data record at the current record index.
Definition: StreamDataReader.hpp:146
Base::DataReader< DataType > & read(std::size_t idx, DataType &obj, bool overwrite=true)
Reads the data record at index idx and stores the read data in obj.
Definition: StreamDataReader.hpp:137
StreamDataReader(std::istream &is)
Constructs a StreamDataReader instance that will read from the input stream is.
Definition: StreamDataReader.hpp:96
A helper class that implements Base::DataReader for std::istream based data readers.
Definition: StreamDataReader.hpp:73
bool hasMoreData()
Tells if there are any data records left to read.
Definition: StreamDataReader.hpp:159