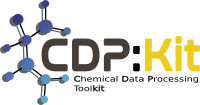 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_GRID_SPATIALGRID_HPP
28 #define CDPL_GRID_SPATIALGRID_HPP
45 template <
typename T,
typename CVT = T>
74 #endif // CDPL_GRID_SPATIALGRID_HPP
SpatialGrid & operator=(const SpatialGrid &grid)
Definition: SpatialGrid.hpp:62
virtual const ValueType & operator()(std::size_t i) const =0
AttributedGrid & operator=(const AttributedGrid &grid)
Definition: AttributedGrid.hpp:58
SpatialGrid< double > DSpatialGrid
Definition: SpatialGrid.hpp:70
virtual ValueType & operator()(std::size_t i)=0
Definition of a grid data type.
T ValueType
Definition: SpatialGrid.hpp:51
Definition: Vector.hpp:1053
SpatialGrid.
Definition: SpatialGrid.hpp:47
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
virtual void getCoordinates(std::size_t i, CoordinatesType &coords) const =0
Math::CVector< CVT, 3 > CoordinatesType
Definition: SpatialGrid.hpp:53
The namespace of the Chemical Data Processing Library.
AttributedGrid.
Definition: AttributedGrid.hpp:46
CVT CoordinatesValueType
Definition: SpatialGrid.hpp:52
std::shared_ptr< SpatialGrid > SharedPointer
Definition: SpatialGrid.hpp:50
Definition of vector data types.
SpatialGrid< float > FSpatialGrid
Definition: SpatialGrid.hpp:69