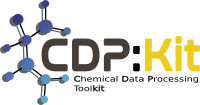 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_SPATIALENTITYALIGNMENT_HPP
30 #define CDPL_CHEM_SPATIALENTITYALIGNMENT_HPP
36 #include <unordered_set>
39 #include <boost/functional/hash.hpp>
259 struct TopMappingCmpFunc
268 struct TopMappingHashFunc
273 return boost::hash_value(
m->getData());
277 struct TopMappingEqCmpFunc
282 return (m1->getData() == m2->getData());
286 typedef Util::ObjectStack<Util::STPairArray> TopMappingCache;
287 typedef std::vector<Math::Vector3D> Vector3DArray;
288 typedef std::vector<double> DoubleArray;
289 typedef std::multiset<Util::STPairArray*, TopMappingCmpFunc> TopMappingSet;
290 typedef typename TopMappingSet::iterator TopMappingSetIterator;
291 typedef std::unordered_set<const Util::STPairArray*, TopMappingHashFunc, TopMappingEqCmpFunc> TopMappingHashSet;
293 TopologicalAlignment topAlignment;
294 TopMappingSet topMappings;
295 TopMappingSetIterator nextTopMappingIter;
300 Math::KabschAlgorithm<double> kabschAlgorithm;
302 DoubleArray firstSetWeights;
304 DoubleArray secondSetWeights;
309 std::size_t minTopMappingSize;
312 TopMappingHashSet seenTopMappings;
313 TopMappingCache topMappingCache;
321 template <
typename T>
323 minTopMappingSize(3), changes(true), exhaustiveMode(true), topMappingCache(5000)
325 currTopMapping = allocTopMapping();
328 template <
typename T>
331 minTopMappingSize = min_size;
335 template <
typename T>
338 return minTopMappingSize;
341 template <
typename T>
348 template <
typename T>
355 template <
typename T>
362 template <
typename T>
369 template <
typename T>
372 topAlignConstrFunc = func;
376 template <
typename T>
380 return topAlignConstrFunc;
383 template <
typename T>
386 topAlignment.setEntityMatchFunction(func);
389 template <
typename T>
393 return topAlignment.getEntityMatchFunction();
396 template <
typename T>
399 topAlignment.setEntityPairMatchFunction(func);
402 template <
typename T>
406 return topAlignment.getEntityPairMatchFunction();
409 template <
typename T>
412 exhaustiveMode = exhaustive;
416 template <
typename T>
419 return exhaustiveMode;
422 template <
typename T>
425 return topAlignment.getNumEntities(first_set);
428 template <
typename T>
431 topAlignment.addEntity(entity, first_set);
435 template <
typename T>
438 topAlignment.clearEntities(first_set);
442 template <
typename T>
446 return topAlignment.getEntitiesBegin(first_set);
449 template <
typename T>
453 return topAlignment.getEntitiesEnd(first_set);
456 template <
typename T>
460 return topAlignment.getEntity(idx, first_set);
463 template <
typename T>
469 template <
typename T>
475 if (firstSetCoords.empty() || secondSetCoords.empty())
478 bool have_weights = !firstSetWeights.empty();
479 std::size_t min_sub_mpg_size = (minTopMappingSize < 3 ? minTopMappingSize : std::size_t(3));
481 while (nextTopMappingIter != topMappings.end()) {
482 currTopMapping = *nextTopMappingIter;
484 std::size_t num_points = currTopMapping->getSize();
486 refPoints.resize(3, num_points,
false);
487 alignedPoints.resize(3, num_points,
false);
490 almntWeights.resize(num_points, 1.0);
495 it != end; ++it, i++) {
497 std::size_t first_idx = it->first;
498 std::size_t sec_idx = it->second;
500 column(refPoints, i) = firstSetCoords[first_idx];
501 column(alignedPoints, i) = secondSetCoords[sec_idx];
504 almntWeights(i) = std::max(firstSetWeights[first_idx], secondSetWeights[sec_idx]);
507 if (exhaustiveMode && (num_points > min_sub_mpg_size)) {
510 for (std::size_t j = 0; j < num_points; j++) {
512 sub_mpg = allocTopMapping();
516 for (std::size_t k = 0; k < num_points; k++)
518 sub_mpg->
addElement(currTopMapping->getElement(k));
520 if (!seenTopMappings.insert(sub_mpg).second) {
524 topMappings.insert(sub_mpg);
529 topMappingCache.put();
533 if (!kabschAlgorithm.align(alignedPoints, refPoints)) {
534 ++nextTopMappingIter;
538 }
else if (!kabschAlgorithm.align(alignedPoints, refPoints, almntWeights)) {
539 ++nextTopMappingIter;
543 transform.assign(kabschAlgorithm.getTransform());
544 ++nextTopMappingIter;
552 template <
typename T>
558 template <
typename T>
562 return *currTopMapping;
565 template <
typename T>
568 firstSetCoords.clear();
569 secondSetCoords.clear();
571 firstSetWeights.clear();
572 secondSetWeights.clear();
575 for (ConstEntityIterator it = getEntitiesBegin(
true), end = getEntitiesEnd(
true); it != end; ++it) {
576 const EntityType& entity = *it;
578 firstSetCoords.push_back(coordsFunc(entity));
581 firstSetWeights.push_back(weightFunc(entity));
584 for (ConstEntityIterator it = getEntitiesBegin(
false), end = getEntitiesEnd(
false); it != end; ++it) {
585 const EntityType& entity = *it;
587 secondSetCoords.push_back(coordsFunc(entity));
590 secondSetWeights.push_back(weightFunc(entity));
594 topAlignment.
reset();
595 topMappingCache.putAll();
596 seenTopMappings.clear();
599 currTopMapping = allocTopMapping();
601 while (topAlignment.nextAlignment(*currTopMapping)) {
602 if (currTopMapping->getSize() < minTopMappingSize)
605 if (topAlignConstrFunc && !topAlignConstrFunc(*currTopMapping))
608 topMappings.insert(currTopMapping);
609 currTopMapping = allocTopMapping();
612 currTopMapping->clear();
614 nextTopMappingIter = topMappings.begin();
618 template <
typename T>
622 return topMappingCache.get();
625 #endif // CDPL_CHEM_SPATIALENTITYALIGNMENT_HPP
std::function< bool(const EntityType &, const EntityType &, const EntityType &, const EntityType &)> EntityPairMatchFunction
A generic wrapper class used to store a user-defined entity-pair match constraint function.
Definition: TopologicalEntityAlignment.hpp:80
virtual ~SpatialEntityAlignment()
Virtual destructor.
Definition: SpatialEntityAlignment.hpp:107
const EntityWeightFunction & getEntityWeightFunction() const
Returns the function that was registered for the retrieval of entity weights for spatial alignment.
Definition: SpatialEntityAlignment.hpp:364
Definition of the class CDPL::Util::ObjectStack.
const EntityMatchFunction & getEntityMatchFunction() const
Returns the function that was registered for restricting allowed topological entity mappings.
Definition: SpatialEntityAlignment.hpp:391
bool exhaustiveSearchPerformed() const
Definition: SpatialEntityAlignment.hpp:417
Matrix< double > DMatrix
An unbounded dense matrix holding floating point values of type double..
Definition: Matrix.hpp:1814
MatrixColumn< M > column(MatrixExpression< M > &e, typename MatrixColumn< M >::SizeType j)
Definition: MatrixProxy.hpp:730
CVector< double, 3 > Vector3D
A bounded 3 element vector holding floating point values of type double.
Definition: Vector.hpp:1637
const Entity3DCoordinatesFunction & getEntity3DCoordinatesFunction() const
Returns the function that was registered for the retrieval of entity 3D-coordinates.
Definition: SpatialEntityAlignment.hpp:350
TopologicalEntityAlignment.
Definition: TopologicalEntityAlignment.hpp:54
SpatialEntityAlignment.
Definition: SpatialEntityAlignment.hpp:59
std::size_t getNumEntities(bool first_set) const
Returns the number of entities in the specified alignment entity set.
Definition: SpatialEntityAlignment.hpp:423
A dynamic array class providing amortized constant time access to arbitrary elements.
Definition: Array.hpp:92
std::function< bool(const EntityType &, const EntityType &)> EntityMatchFunction
A generic wrapper class used to store a user-defined entity match constraint function.
Definition: TopologicalEntityAlignment.hpp:75
CMatrix< double, 4, 4 > Matrix4D
A bounded 4x4 matrix holding floating point values of type double.
Definition: Matrix.hpp:1854
void clear()
Erases all elements.
Definition: Array.hpp:732
std::size_t getMinTopologicalMappingSize()
Returns the minimum number of topologically mapped entities that is required to enable a subsequent s...
Definition: SpatialEntityAlignment.hpp:336
ConstEntityIterator getEntitiesEnd(bool first_set) const
Returns a constant iterator pointing to the end of the entities stored in the specified set.
Definition: SpatialEntityAlignment.hpp:451
void addElement(const ValueType &value=ValueType())
Inserts a new element at the end of the array.
Definition: Array.hpp:757
void setTopAlignmentConstraintFunction(const TopologicalAlignmentConstraintFunction &func)
Specifies a function for restricting allowed topological entity alignments.
Definition: SpatialEntityAlignment.hpp:370
Vector< double > DVector
An unbounded dense vector holding floating point values of type double.
Definition: Vector.hpp:1687
std::size_t getSize() const
Returns the number of elements stored in the array.
Definition: Array.hpp:696
TopologicalAlignment::ConstEntityIterator ConstEntityIterator
A constant iterator over the stored entities.
Definition: SpatialEntityAlignment.hpp:72
void transform(VectorArray< CVector< T, Dim > > &va, const CMatrix< T1, Dim, Dim > &xform)
Transforms each -dimensional vector in the array with the -dimensional square matrix xform.
Definition: VectorArrayFunctions.hpp:51
StorageType::const_iterator ConstElementIterator
A constant random access iterator used to iterate over the elements of the array.
Definition: Array.hpp:125
void setMinTopologicalMappingSize(std::size_t min_size)
Specifies the minimum number of topologically mapped entities that is required to enable a subsequent...
Definition: SpatialEntityAlignment.hpp:329
VectorArray< Vector3D > Vector3DArray
An array of Math::Vector3D objects.
Definition: VectorArray.hpp:84
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
T EntityType
The actual entity type.
Definition: SpatialEntityAlignment.hpp:67
Array< STPair > STPairArray
An array of pairs of unsigned integers of type std::size_t.
Definition: Array.hpp:582
void setEntity3DCoordinatesFunction(const Entity3DCoordinatesFunction &func)
Specifies a function for the retrieval of entity 3D-coordinates.
Definition: SpatialEntityAlignment.hpp:342
void reset()
Definition: SpatialEntityAlignment.hpp:464
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Implementation of the Kabsch algorithm.
const TopologicalAlignmentConstraintFunction & getTopAlignmentConstraintFunction() const
Returns the function that was registered for restricting allowed topological entity alignments.
Definition: SpatialEntityAlignment.hpp:378
const EntityType & getEntity(std::size_t idx, bool first_set) const
Returns a non-const reference to the stored entity at index idx in the specified set.
Definition: SpatialEntityAlignment.hpp:458
void clearEntities(bool first_set)
Removes all entities in the specified alignment entity set.
Definition: SpatialEntityAlignment.hpp:436
void setEntityWeightFunction(const EntityWeightFunction &func)
Specifies a function for the retrieval of entity weights for spatial alignment.
Definition: SpatialEntityAlignment.hpp:356
The namespace of the Chemical Data Processing Library.
std::function< double(const EntityType &)> EntityWeightFunction
A generic wrapper class used to store a user-defined entity alignment weight function.
Definition: SpatialEntityAlignment.hpp:87
void addEntity(const EntityType &entity, bool first_set)
Adds an entity to the specified alignment entity set.
Definition: SpatialEntityAlignment.hpp:429
SpatialEntityAlignment()
Constructs the SpatialEntityAlignment instance.
Definition: SpatialEntityAlignment.hpp:322
ConstEntityIterator getEntitiesBegin(bool first_set) const
Returns a constant iterator pointing to the beginning of the entities stored in the specified set.
Definition: SpatialEntityAlignment.hpp:444
boost::indirect_iterator< typename EntitySet::const_iterator, const EntityType > ConstEntityIterator
A constant iterator over the stored entities.
Definition: TopologicalEntityAlignment.hpp:70
void setEntityMatchFunction(const EntityMatchFunction &func)
Specifies a function for restricting allowed topological entity mappings in the search for alignment ...
Definition: SpatialEntityAlignment.hpp:384
void setEntityPairMatchFunction(const EntityPairMatchFunction &func)
Specifies a function for checking the compatibility of entity-pairs in the search for alignment solut...
Definition: SpatialEntityAlignment.hpp:397
Definition of matrix data types.
const Math::Matrix4D & getTransform() const
Returns the alignment transformation matrix that was calculated in the last successful call to nextAl...
Definition: SpatialEntityAlignment.hpp:553
TopologicalAlignment::EntityMatchFunction EntityMatchFunction
A generic wrapper class used to store a user-defined topological entity match constraint function.
Definition: SpatialEntityAlignment.hpp:92
TopologicalAlignment::EntityPairMatchFunction EntityPairMatchFunction
A generic wrapper class used to store a user-defined entity-pair match constraint function.
Definition: SpatialEntityAlignment.hpp:97
bool nextAlignment()
Searches for the next alignment solution.
Definition: SpatialEntityAlignment.hpp:470
void performExhaustiveSearch(bool exhaustive)
Definition: SpatialEntityAlignment.hpp:410
const EntityPairMatchFunction & getEntityPairMatchFunction() const
Returns the function that was registered for checking the compatibility of entity-pairs.
Definition: SpatialEntityAlignment.hpp:404
Definition of the class CDPL::Chem::TopologicalEntityAlignment.
std::function< bool(const Util::STPairArray &)> TopologicalAlignmentConstraintFunction
A generic wrapper class used to store a user-defined predicate to restrict allowed topological entity...
Definition: SpatialEntityAlignment.hpp:77
Definition of vector data types.
std::function< const Math::Vector3D &(const EntityType &)> Entity3DCoordinatesFunction
A generic wrapper class used to store a user-defined entity 3D-coordinates function.
Definition: SpatialEntityAlignment.hpp:82
const Util::STPairArray & getTopologicalMapping() const
Returns the topological entity mapping resulting from the last successful call to nextAlignment().
Definition: SpatialEntityAlignment.hpp:560