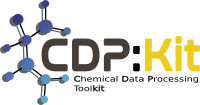 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_SPARSECONTAINERELEMENT_HPP
28 #define CDPL_MATH_SPARSECONTAINERELEMENT_HPP
40 template <
typename C,
typename K =
typename C::KeyType>
47 typedef typename ContainerType::ValueType
ValueType;
48 typedef typename ContainerType::SizeType
SizeType;
51 typedef typename ContainerType::ArrayType
ArrayType;
103 template <
typename D>
114 template <
typename D>
122 template <
typename D>
141 cntnr.getData().erase(key);
144 std::pair<typename ArrayType::iterator, bool> pos = cntnr.getData().insert(
typename ArrayType::value_type(key, v));
147 pos.first->second = v;
153 typename ArrayType::const_iterator it = cntnr.getData().find(key);
155 if (it == cntnr.getData().end())
166 template <
typename C>
172 #endif // CDPL_MATH_SPARSECONTAINERELEMENT_HPP
const unsigned int p
Specifies that the stereocenter has p configuration.
Definition: CIPDescriptor.hpp:121
Definition: TypeTraits.hpp:171
K KeyType
Definition: SparseContainerElement.hpp:46
SparseContainerElement & operator/=(const D &d)
Definition: SparseContainerElement.hpp:104
ContainerType::SizeType SizeType
Definition: SparseContainerElement.hpp:48
C ContainerType
Definition: SparseContainerElement.hpp:45
Definition: SparseContainerElement.hpp:42
SparseContainerElement & operator=(const SparseContainerElement &p)
Definition: SparseContainerElement.hpp:57
SparseContainerElement & operator*=(const D &d)
Definition: SparseContainerElement.hpp:94
ContainerType::ValueType ValueType
Definition: SparseContainerElement.hpp:47
bool operator==(const D &d) const
Definition: SparseContainerElement.hpp:115
ContainerType::ConstReference ConstReference
Definition: SparseContainerElement.hpp:50
ContainerType::ArrayType ArrayType
Definition: SparseContainerElement.hpp:51
bool operator!=(const D &d) const
Definition: SparseContainerElement.hpp:123
Definition of type traits.
SparseContainerElement(ContainerType &c, KeyType key)
Definition: SparseContainerElement.hpp:53
The namespace of the Chemical Data Processing Library.
SparseContainerElement & operator-=(const D &d)
Definition: SparseContainerElement.hpp:84
const unsigned int K
Specifies Potassium.
Definition: AtomType.hpp:157
ValueType & Reference
Definition: SparseContainerElement.hpp:49
SparseContainerElement & operator+=(const D &d)
Definition: SparseContainerElement.hpp:74
const unsigned int D
Specifies Hydrogen (Deuterium).
Definition: AtomType.hpp:62
Definition of various preprocessor macros for error checking.
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
SparseContainerElement & operator=(const D &d)
Definition: SparseContainerElement.hpp:66