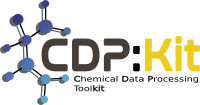 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
32 #ifndef CDPL_CHEM_SMALLESTSETOFSMALLESTRINGS_HPP
33 #define CDPL_CHEM_SMALLESTSETOFSMALLESTRINGS_HPP
89 typedef std::vector<MessagePtr> MessageList;
95 void visitComponentAtom(
const Atom& atom);
99 void createRingFragments();
103 bool sssrComplete()
const;
105 MessagePtr allocMessage();
106 MessagePtr allocMessage(std::size_t, std::size_t, std::size_t, std::size_t);
108 bool processCollision(
const MessagePtr&,
const MessagePtr&, std::size_t,
bool);
109 void processRing(
const MessagePtr&);
110 void processEvenRings();
112 const char* getClassName()
const
114 return "SmallestSetOfSmallestRings";
121 TNode(std::size_t idx):
124 bool send(Controller*);
125 bool receive(Controller*);
127 std::size_t getIndex()
const;
128 std::size_t getNumNeighbors()
const;
130 void initMessages(Controller* ctrl, std::size_t max_num_atoms, std::size_t max_num_bonds);
132 static void connect(TNode*, TNode*, std::size_t);
135 typedef std::vector<TNode*> NodeList;
136 typedef std::vector<std::size_t> BondIndexList;
139 BondIndexList bondIndices;
140 MessageList receiveBuffer;
141 MessageList sendBuffer;
155 void init(std::size_t, std::size_t, std::size_t, std::size_t);
157 void copy(
const MessagePtr&);
158 bool join(
const MessagePtr&,
const MessagePtr&);
160 void addAtom(std::size_t);
161 void addBond(std::size_t);
163 bool containsAtom(std::size_t
const);
164 bool containsBond(std::size_t
const);
166 std::size_t getFirstAtomIndex()
const;
167 std::size_t getFirstBondIndex()
const;
169 std::size_t getMaxBondIndex()
const;
176 void setRemoveFlag();
177 void setEdgeCollisionFlag();
179 bool getRemoveFlag()
const;
180 bool getEdgeCollisionFlag()
const;
182 PathMessage& operator^=(
const PathMessage&);
187 std::size_t firstAtomIdx;
188 std::size_t firstBondIdx;
189 std::size_t maxBondIdx;
194 struct TestMatrixRowCmpFunc
197 bool operator()(
const MessagePtr&,
const MessagePtr&)
const;
200 typedef std::vector<TNode> NodeArray;
201 typedef std::set<MessagePtr, PathMessage::LessCmpFunc> ProcRingSet;
203 MessageCache msgCache;
208 ProcRingSet procRings;
209 MessageList evenRings;
211 MessagePtr linDepTestRing;
212 MessageList linDepTestMtx;
214 std::size_t sssrSize;
219 #endif // CDPL_CHEM_SMALLESTSETOFSMALLESTRINGS_HPP
Definition of the class CDPL::Util::ObjectPool.
Definition of the preprocessor macro CDPL_CHEM_API.
SmallestSetOfSmallestRings()
Constructs an empty SmallestSetOfSmallestRings instance.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
boost::dynamic_bitset BitSet
A dynamic bitset class.
Definition: BitSet.hpp:46
bool operator()(const MessagePtr &, const MessagePtr &) const
Atom.
Definition: Atom.hpp:52
Fragment.
Definition: Fragment.hpp:52
void perceive(const MolecularGraph &molgraph)
Replaces the current set of rings by the SSSR of the molecular graph molgraph.
Implements the perception of ring atoms and bonds in a molecular graph.
Definition: CyclicSubstructure.hpp:50
MolecularGraph.
Definition: MolecularGraph.hpp:52
Definition of the type CDPL::Util::BitSet.
std::shared_ptr< ObjectType > SharedObjectPointer
Definition: ObjectPool.hpp:65
Definition of the class CDPL::Chem::CyclicSubstructure.
std::shared_ptr< Fragment > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Fragment instances.
Definition: Fragment.hpp:61
A data type for the storage of Chem::Fragment objects.
Definition: FragmentList.hpp:49
std::shared_ptr< SmallestSetOfSmallestRings > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated SmallestSetOfSmallestRings instan...
Definition: SmallestSetOfSmallestRings.hpp:64
Implements the perception of the Smallest Set of Smallest Rings (SSSR) of a molecular graphs.
Definition: SmallestSetOfSmallestRings.hpp:58
The namespace of the Chemical Data Processing Library.
Definition: SmallestSetOfSmallestRings.hpp:150
SmallestSetOfSmallestRings(const MolecularGraph &molgraph)
Constructs a SmallestSetOfSmallestRings instance containing the SSSR of the molecular graph molgraph.
Definition of the class CDPL::Chem::FragmentList.