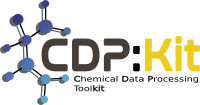 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_SLICE_HPP
28 #define CDPL_MATH_SLICE_HPP
43 template <
typename S,
typename D>
54 start(0), stride(0), size(0) {}
57 start(start), stride(stride), size(size)
65 return (start + i * stride);
90 return (start ==
s.start && stride ==
s.stride && size ==
s.size);
103 std::swap(start,
s.start);
104 std::swap(stride,
s.stride);
105 std::swap(size,
s.size);
119 inline Slice<std::size_t, std::ptrdiff_t>
120 slice(std::size_t start, std::ptrdiff_t stride, std::size_t size)
127 #endif // CDPL_MATH_SLICE_HPP
bool operator!=(const Slice &s) const
Definition: Slice.hpp:93
Thrown to indicate that a value is out of range.
Definition: Base/Exceptions.hpp:114
MatrixSlice< E > slice(MatrixExpression< E > &e, const typename MatrixSlice< E >::SliceType &s1, const typename MatrixSlice< E >::SliceType &s2)
Definition: MatrixProxy.hpp:788
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
bool isEmpty() const
Definition: Slice.hpp:83
Thrown to indicate that an index is out of range.
Definition: Base/Exceptions.hpp:152
Slice(SizeType start, DifferenceType stride, SizeType size)
Definition: Slice.hpp:56
const unsigned int S
Specifies that the atom has S configuration.
Definition: AtomConfiguration.hpp:63
S SizeType
Definition: Slice.hpp:50
Definition of exception classes.
const unsigned int s
Specifies that the stereocenter has s configuration.
Definition: CIPDescriptor.hpp:81
DifferenceType getStride() const
Definition: Slice.hpp:73
bool operator==(const Slice &s) const
Definition: Slice.hpp:88
SizeType getStart() const
Definition: Slice.hpp:68
The namespace of the Chemical Data Processing Library.
D DifferenceType
Definition: Slice.hpp:51
void swap(Slice &s)
Definition: Slice.hpp:98
const unsigned int D
Specifies Hydrogen (Deuterium).
Definition: AtomType.hpp:62
Definition of various preprocessor macros for error checking.
Slice()
Definition: Slice.hpp:53
friend void swap(Slice &s1, Slice &s2)
Definition: Slice.hpp:108
SizeType operator()(SizeType i) const
Definition: Slice.hpp:62
SizeType getSize() const
Definition: Slice.hpp:78