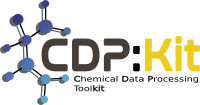 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_SHAPE_SCREENINGSETTINGS_HPP
30 #define CDPL_SHAPE_SCREENINGSETTINGS_HPP
45 class AlignmentResult;
52 static constexpr
double NO_CUTOFF = std::numeric_limits<double>::quiet_NaN();
61 BEST_MATCH_PER_QUERY_CONF
69 PHARMACOPHORE_IMP_CHARGES
78 COLOR_FEATURE_CENTERS = 0x4,
137 std::size_t numRandomStarts;
139 bool singleConfSearch;
142 std::size_t numOptIter;
149 #endif // CDPL_SHAPE_SCREENINGSETTINGS_HPP
ColorFeatureType
Definition: ScreeningSettings.hpp:65
AlignmentMode
Definition: ScreeningSettings.hpp:73
const ScoringFunction & getScoringFunction() const
static const ScreeningSettings DEFAULT
Definition: ScreeningSettings.hpp:51
@ NO_FEATURES
Definition: ScreeningSettings.hpp:67
ScreeningMode
Definition: ScreeningSettings.hpp:57
double getScoreCutoff() const
bool optimizeOverlap() const
void setScoringFunction(const ScoringFunction &func)
void setMaxNumOptimizationIterations(std::size_t max_iter)
bool greedyOptimization() const
ScreeningMode getScreeningMode() const
void singleConformerSearch(bool single_conf)
void optimizeOverlap(bool optimize)
void setColorFeatureType(ColorFeatureType type)
void greedyOptimization(bool greedy)
void allCarbonMode(bool all_c)
std::size_t getMaxNumOptimizationIterations() const
void setNumRandomStarts(std::size_t num_starts)
@ PHARMACOPHORE_EXP_CHARGES
Definition: ScreeningSettings.hpp:68
std::function< double(const AlignmentResult &)> ScoringFunction
Definition: ScreeningSettings.hpp:54
@ BEST_MATCH_PER_QUERY
Definition: ScreeningSettings.hpp:60
void setOptimizationStopGradient(double grad_norm)
#define CDPL_SHAPE_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
std::size_t getNumRandomStarts() const
void setScoreCutoff(double cutoff)
ColorFeatureType getColorFeatureType() const
The namespace of the Chemical Data Processing Library.
Definition of the preprocessor macro CDPL_SHAPE_API.
Definition: AlignmentResult.hpp:45
void setScreeningMode(ScreeningMode mode)
AlignmentMode getAlignmentMode() const
bool allCarbonMode() const
void setAlignmentMode(AlignmentMode mode)
double getOptimizationStopGradient() const
bool singleConformerSearch() const
Definition: ScreeningSettings.hpp:48
@ BEST_OVERALL_MATCH
Definition: ScreeningSettings.hpp:59