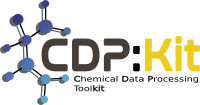 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_MATH_SVBACKSUBSTITUTION_HPP
30 #define CDPL_MATH_SVBACKSUBSTITUTION_HPP
65 template <
typename M1,
typename V1,
typename M2,
typename V2,
typename V3>
73 template <
typename M1,
typename V1,
typename M2,
typename V2,
typename V3>
76 typedef typename V3::value_type
T;
78 typename M1::size_type n = u.size2();
82 for (
typename M1::size_type j = 0; j < n; j++) {
86 s = inner_prod(
column(u, j), b) / w(j);
94 #endif // CDPL_MATH_SVBACKSUBSTITUTION_HPP
MatrixColumn< M > column(MatrixExpression< M > &e, typename MatrixColumn< M >::SizeType j)
Definition: MatrixProxy.hpp:730
Matrix1VectorBinaryTraits< E1, E2, MatrixVectorProduct< E1, E2 > >::ResultType prod(const MatrixExpression< E1 > &e1, const VectorExpression< E2 > &e2)
Definition: MatrixExpression.hpp:833
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
const unsigned int s
Specifies that the stereocenter has s configuration.
Definition: CIPDescriptor.hpp:81
The namespace of the Chemical Data Processing Library.
void svBackSubstitution(const M1 &u, const V1 &w, const M2 &v, const V2 &b, V3 &x)
Solves for a vector where is given by its Singular Value Decomposition [WSVD].
Definition: SVBackSubstitution.hpp:74
Definition of matrix data types.
Definition of vector data types.