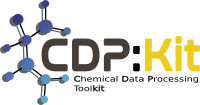 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_BIOMOL_RESIDUEDICTIONARY_HPP
30 #define CDPL_BIOMOL_RESIDUEDICTIONARY_HPP
33 #include <unordered_map>
37 #include <boost/iterator/transform_iterator.hpp>
63 Entry(
const std::string& code,
const std::string& rep_code,
const std::string& rep_by_code,
bool obsolete,
84 std::string replacesCode;
85 std::string replacedByCode;
93 typedef std::unordered_map<std::string, Entry> EntryLookupTable;
98 typedef boost::transform_iterator<std::function<
const Entry&(
const EntryLookupTable::value_type&)>,
99 EntryLookupTable::const_iterator>
134 static const std::string&
getName(
const std::string& code);
138 static unsigned int getType(
const std::string& code);
144 EntryLookupTable entries;
149 #endif // CDPL_BIOMOL_RESIDUEDICTIONARY_HPP
std::shared_ptr< MolecularGraph > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated MolecularGraph instances.
Definition: MolecularGraph.hpp:58
ConstEntryIterator getEntriesEnd() const
static bool isObsolete(const std::string &code)
const std::string & getReplacedByCode() const
static const std::string & getReplacedCode(const std::string &code)
static bool isStdResidue(const std::string &code)
static void set(const SharedPointer &dict)
const std::string & getName() const
bool containsEntry(const std::string &code) const
const std::string & getReplacedCode() const
static unsigned int getType(const std::string &code)
static Chem::MolecularGraph::SharedPointer getStructure(const std::string &code)
unsigned int getType() const
static const std::string & getReplacedByCode(const std::string &code)
Definition: ResidueDictionary.hpp:58
void removeEntry(const std::string &code)
ConstEntryIterator getEntriesBegin() const
Definition of the class CDPL::Chem::MolecularGraph.
Entry(const std::string &code, const std::string &rep_code, const std::string &rep_by_code, bool obsolete, const std::string &name, unsigned int type, const StructureRetrievalFunction &struc_ret_func)
const std::string & getCode() const
std::size_t getNumEntries() const
A global dictionary for the lookup of meta-data associated with the residues in biological macromolec...
Definition: ResidueDictionary.hpp:54
Chem::MolecularGraph::SharedPointer getStructure() const
const Entry & getEntry(const std::string &code) const
static const std::string & getName(const std::string &code)
std::function< Chem::MolecularGraph::SharedPointer(const std::string &)> StructureRetrievalFunction
Definition: ResidueDictionary.hpp:61
Definition of the preprocessor macro CDPL_BIOMOL_API.
void addEntry(const Entry &entry)
The namespace of the Chemical Data Processing Library.
std::shared_ptr< ResidueDictionary > SharedPointer
Definition: ResidueDictionary.hpp:96
ConstEntryIterator begin() const
ConstEntryIterator end() const
boost::transform_iterator< std::function< const Entry &(const EntryLookupTable::value_type &)>, EntryLookupTable::const_iterator > ConstEntryIterator
Definition: ResidueDictionary.hpp:100
#define CDPL_BIOMOL_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
static const SharedPointer & get()