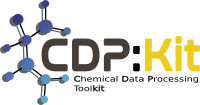 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_VIS_RENDERER2D_HPP
30 #define CDPL_VIS_RENDERER2D_HPP
156 virtual void drawRectangle(
double x,
double y,
double width,
double height) = 0;
181 virtual void drawLine(
double x1,
double y1,
double x2,
double y2) = 0;
230 virtual void drawEllipse(
double x,
double y,
double width,
double height) = 0;
254 virtual void drawText(
double x,
double y,
const std::string& txt) = 0;
274 #endif // CDPL_VIS_RENDERER2D_HPP
virtual void drawEllipse(double x, double y, double width, double height)=0
Draws an ellipse with the given width and height around the center position (x, y).
virtual void drawRectangle(double x, double y, double width, double height)=0
Draws an axis aligned rectangle of the specified width and height whose upper-left corner is located ...
An interface that provides methods for low level 2D drawing operations.
Definition: Renderer2D.hpp:86
Definition of the class CDPL::Math::VectorArray.
virtual void setBrush(const Brush &brush)=0
Sets the brush to be used in subsequent drawing operations.
virtual void setPen(const Pen &pen)=0
Sets the pen to be used in subsequent drawing operations.
Specifies how to draw lines and outlines of shapes.
Definition: Pen.hpp:53
virtual void drawPolyline(const Math::Vector2DArray &points)=0
Draws the polyline defined by points.
virtual void saveState()=0
Saves the current renderer state.
virtual void restoreState()=0
Restores the last renderer state saved by a call to saveState().
virtual void drawText(double x, double y, const std::string &txt)=0
Draws the specified text at the position (x, y).
Specifies a font for drawing text.
Definition: Font.hpp:54
virtual void drawLine(double x1, double y1, double x2, double y2)=0
Draws a line segment from (x1, y1) to (x2, y2).
Provides a container for painting operations, enabling arbitrary graphical shapes to be constructed a...
Definition: Path2D.hpp:78
virtual void drawPolygon(const Math::Vector2DArray &points)=0
Draws the polygon defined by points.
virtual void transform(const Math::Matrix3D &xform)=0
Multiplies the current affine transformation matrix by xform.
virtual void setTransform(const Math::Matrix3D &xform)=0
Sets the applied affine transformation matrix to xform.
#define CDPL_VIS_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
virtual ~Renderer2D()
Virtual destructor.
Definition: Renderer2D.hpp:92
CMatrix< double, 3, 3 > Matrix3D
A bounded 3x3 matrix holding floating point values of type double.
Definition: Matrix.hpp:1849
virtual void setClipPath(const Path2D &path)=0
The namespace of the Chemical Data Processing Library.
virtual void setFont(const Font &font)=0
Sets the font to be used in subsequent text drawing operations.
virtual void drawPoint(double x, double y)=0
Draws a point at the position (x, y).
Specifies the fill pattern and fill color of shapes.
Definition: Brush.hpp:50
virtual void drawLineSegments(const Math::Vector2DArray &points)=0
Draws the sequence of disjoint line segments defined by points.
Definition of matrix data types.
VectorArray< Vector2D > Vector2DArray
An array of Math::Vector2D objects.
Definition: VectorArray.hpp:79
Definition of the preprocessor macro CDPL_VIS_API.
virtual void clearClipPath()=0
Disables clipping.
virtual void drawPath(const Path2D &path)=0
Draws the given path.