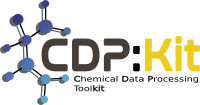 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
27 #ifndef CDPL_MATH_REGULARSPATIALGRID_HPP
28 #define CDPL_MATH_REGULARSPATIALGRID_HPP
30 #include <type_traits>
48 template <
typename MatrixType>
52 static void init(MatrixType& mtx)
58 static bool invert(
const MatrixType& mtx,
M& inv_mtx)
63 template <
typename V,
typename R>
82 template <typename T, typename C = typename TypeTraits<T>::RealType,
typename GD =
Grid<T>,
typename XF =
CMatrix<C, 4, 4> >
101 typedef typename std::conditional<std::is_const<GD>::value,
102 typename GD::ConstReference,
113 dataMode(
POINT), xStep(xs), yStep(ys), zStep(zs)
121 dataMode(
POINT), data(data), xStep(xs), yStep(ys), zStep(zs)
129 dataMode(
POINT), xStep(
s), yStep(
s), zStep(
s)
137 dataMode(
POINT), data(data), xStep(
s), yStep(
s), zStep(
s)
145 dataMode(usg.dataMode), data(usg.data), xStep(usg.xStep), yStep(usg.yStep), zStep(usg.zStep),
146 xform(usg.xform), invXform(usg.invXform) {}
149 dataMode(usg.dataMode), data(std::move(usg.data)), xStep(usg.xStep), yStep(usg.yStep), zStep(usg.zStep),
150 xform(usg.xform), invXform(usg.invXform) {}
176 return data(i, j, k);
181 return data(i, j, k);
211 return data.getMaxSize1();
216 return data.getMaxSize2();
221 return data.getMaxSize3();
256 if (dataMode ==
POINT)
264 if (dataMode ==
POINT)
272 if (dataMode ==
POINT)
278 template <
typename V>
289 template <
typename V>
300 coords[0] = world_coords(0);
301 coords[1] = world_coords(1);
302 coords[2] = world_coords(2);
305 template <
typename V>
308 if (dataMode ==
POINT) {
320 template <
typename V1,
typename V2>
325 transformToLocalCoordinates(world_coords, tmp_local_coords);
327 local_coords[0] = tmp_local_coords[0];
328 local_coords[1] = tmp_local_coords[1];
329 local_coords[2] = tmp_local_coords[2];
332 template <
typename V>
337 transformToLocalCoordinates(pos, local_coords);
342 template <
typename V>
366 template <
typename V1,
typename V2>
371 transformToLocalCoordinates(pos, local_coords);
376 template <
typename V1,
typename V2>
383 indices[0] =
SSizeType(std::floor(x / xStep));
384 indices[1] =
SSizeType(std::floor(y / yStep));
385 indices[2] =
SSizeType(std::floor(z / zStep));
408 template <
typename T1>
417 dataMode = usg.dataMode;
420 invXform = usg.invXform;
429 dataMode = usg.dataMode;
430 data = std::move(usg.data);
432 invXform = usg.invXform;
439 template <
typename E>
446 template <
typename E>
453 template <
typename E>
460 template <
typename T1>
467 template <
typename T1>
474 template <
typename E>
481 template <
typename E>
488 template <
typename E>
498 xform.swap(usg.xform);
499 invXform.swap(usg.invXform);
500 std::swap(xStep, usg.xStep);
501 std::swap(yStep, usg.yStep);
502 std::swap(zStep, usg.zStep);
503 std::swap(dataMode, dataMode);
518 data.
resize(
m, n, o, preserve, v);
522 template <
typename V1,
typename V2>
523 void transformToLocalCoordinates(
const V1& coords,
V2& local_coords)
const
527 world_coords(0) = coords[0];
528 world_coords(1) = coords[1];
529 world_coords(2) = coords[2];
544 template <
typename T,
typename C,
typename GD,
typename XF,
typename V>
549 typedef typename GridType::CoordinatesValueType CoordinatesValueType;
550 typedef typename GridType::SSizeType SSizeType;
551 typedef typename GridType::ValueType ValueType;
554 return CoordinatesValueType();
556 CoordinatesValueType loc_pos[3];
570 CoordinatesValueType xyz0[3];
574 SSizeType inds_p1[3];
577 bool recalc_xyz0 =
false;
579 if (loc_pos[0] < xyz0[0]) {
580 inds_p1[0] = inds[0];
585 inds_p1[0] = inds[0] + 1;
587 if (loc_pos[1] < xyz0[1]) {
588 inds_p1[1] = inds[1];
593 inds_p1[1] = inds[1] + 1;
595 if (loc_pos[2] < xyz0[2]) {
596 inds_p1[2] = inds[2];
601 inds_p1[2] = inds[2] + 1;
607 inds_p1[0] = inds[0] + 1;
608 inds_p1[1] = inds[1] + 1;
609 inds_p1[2] = inds[2] + 1;
612 inds[0] = std::max(SSizeType(0), inds[0]);
613 inds[1] = std::max(SSizeType(0), inds[1]);
614 inds[2] = std::max(SSizeType(0), inds[2]);
616 inds[0] = std::min(SSizeType(grid.
getSize1() - 1), inds[0]);
617 inds[1] = std::min(SSizeType(grid.
getSize2() - 1), inds[1]);
618 inds[2] = std::min(SSizeType(grid.
getSize3() - 1), inds[2]);
620 inds_p1[0] = std::max(SSizeType(0), inds_p1[0]);
621 inds_p1[1] = std::max(SSizeType(0), inds_p1[1]);
622 inds_p1[2] = std::max(SSizeType(0), inds_p1[2]);
624 inds_p1[0] = std::min(SSizeType(grid.
getSize1() - 1), inds_p1[0]);
625 inds_p1[1] = std::min(SSizeType(grid.
getSize2() - 1), inds_p1[1]);
626 inds_p1[2] = std::min(SSizeType(grid.
getSize3() - 1), inds_p1[2]);
628 CoordinatesValueType xd = (loc_pos[0] - xyz0[0]) / grid.
getXStepSize();
629 CoordinatesValueType yd = (loc_pos[1] - xyz0[1]) / grid.
getYStepSize();
630 CoordinatesValueType zd = (loc_pos[2] - xyz0[2]) / grid.
getZStepSize();
632 ValueType c00 = grid(inds[0], inds[1], inds[2]) * (1 - xd) + grid(inds_p1[0], inds[1], inds[2]) * xd;
633 ValueType c01 = grid(inds[0], inds[1], inds_p1[2]) * (1 - xd) + grid(inds_p1[0], inds[1], inds_p1[2]) * xd;
634 ValueType c10 = grid(inds[0], inds_p1[1], inds[2]) * (1 - xd) + grid(inds_p1[0], inds_p1[1], inds[2]) * xd;
635 ValueType c11 = grid(inds[0], inds_p1[1], inds_p1[2]) * (1 - xd) + grid(inds_p1[0], inds_p1[1], inds_p1[2]) * xd;
637 ValueType c0 = c00 * (1 - yd) + c10 * yd;
638 ValueType c1 = c01 * (1 - yd) + c11 * yd;
640 ValueType c = c0 * (1 - zd) + c1 * zd;
657 #endif // CDPL_MATH_REGULARSPATIALGRID_HPP
GD::SizeType SizeType
Definition: RegularSpatialGrid.hpp:105
RegularSpatialGrid & plusAssign(const GridExpression< E > &e)
Definition: RegularSpatialGrid.hpp:482
SizeType getMaxSize1() const
Definition: RegularSpatialGrid.hpp:209
RegularSpatialGrid(const CoordinatesValueType &xs, const CoordinatesValueType &ys, const CoordinatesValueType &zs)
Definition: RegularSpatialGrid.hpp:112
GridDataType & getData()
Definition: RegularSpatialGrid.hpp:398
@ POINT
Definition: RegularSpatialGrid.hpp:93
SizeType getSize() const
Definition: RegularSpatialGrid.hpp:184
Definition of various grid expression types and operations.
void swap(RegularSpatialGrid &usg)
Definition: RegularSpatialGrid.hpp:495
void getCoordinates(SSizeType i, SSizeType j, SSizeType k, V &coords) const
Definition: RegularSpatialGrid.hpp:290
void getLocalContainingCell(const V1 &pos, V2 &indices) const
Definition: RegularSpatialGrid.hpp:377
void setDataMode(DataMode mode)
Definition: RegularSpatialGrid.hpp:154
SizeType getSize3() const
Definition: Math/Grid.hpp:313
bool invert(const MatrixExpression< E > &e, MatrixContainer< C > &c)
Definition: Matrix.hpp:1766
RegularSpatialGrid & operator-=(const GridExpression< E > &e)
Definition: RegularSpatialGrid.hpp:454
SizeType getSize2() const
Definition: RegularSpatialGrid.hpp:194
RegularSpatialGrid & operator=(RegularSpatialGrid &&usg)
Definition: RegularSpatialGrid.hpp:427
const CoordinatesTransformType & getCoordinatesTransform() const
Definition: RegularSpatialGrid.hpp:403
void resize(SizeType m, SizeType n, SizeType o, bool preserve=true, const ValueType &v=ValueType())
Definition: Math/Grid.hpp:446
bool isEmpty() const
Definition: Math/Grid.hpp:293
void setYStepSize(const CoordinatesValueType &ys)
Definition: RegularSpatialGrid.hpp:244
Grid & minusAssign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:420
T ValueType
Definition: RegularSpatialGrid.hpp:96
Definition: Matrix.hpp:1152
CoordinatesValueType getXExtent() const
Definition: RegularSpatialGrid.hpp:254
Reference operator()(SizeType i, SizeType j, SizeType k)
Definition: RegularSpatialGrid.hpp:174
SizeType getMaxSize() const
Definition: Math/Grid.hpp:318
RegularSpatialGrid & operator+=(const GridExpression< E > &e)
Definition: RegularSpatialGrid.hpp:447
void clear(const ValueType &v=ValueType())
Definition: Math/Grid.hpp:441
XF CoordinatesTransformType
Definition: RegularSpatialGrid.hpp:99
void getContainingCell(const V1 &pos, V2 &indices) const
Definition: RegularSpatialGrid.hpp:367
RegularSpatialGrid(RegularSpatialGrid &&usg)
Definition: RegularSpatialGrid.hpp:148
GD::DifferenceType DifferenceType
Definition: RegularSpatialGrid.hpp:107
RegularSpatialGrid(const RegularSpatialGrid &usg)
Definition: RegularSpatialGrid.hpp:144
#define CDPL_MATH_CHECK(expr, msg, e)
Definition: Check.hpp:36
void swap(Grid &g)
Definition: Math/Grid.hpp:426
RegularSpatialGrid(const GridDataType &data, const CoordinatesValueType &s)
Definition: RegularSpatialGrid.hpp:136
void setXStepSize(const CoordinatesValueType &xs)
Definition: RegularSpatialGrid.hpp:239
const unsigned int R
Specifies that the atom has R configuration.
Definition: AtomConfiguration.hpp:58
SizeType getSize1() const
Definition: Math/Grid.hpp:303
T interpolateTrilinear(const RegularSpatialGrid< T, C, GD, XF > &grid, const V &pos, bool local_pos)
Definition: RegularSpatialGrid.hpp:545
Grid & plusAssign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:413
std::ptrdiff_t SSizeType
Definition: RegularSpatialGrid.hpp:106
void getCoordinates(SizeType i, V &coords) const
Definition: RegularSpatialGrid.hpp:279
DataMode
Definition: RegularSpatialGrid.hpp:90
CoordinatesTransformType::MatrixTemporaryType InvCoordinatesTransformType
Definition: RegularSpatialGrid.hpp:100
Definition: Matrix.hpp:854
ConstReference operator()(SizeType i, SizeType j, SizeType k) const
Definition: RegularSpatialGrid.hpp:179
CoordinatesValueType getXStepSize() const
Definition: RegularSpatialGrid.hpp:224
ConstReference operator()(SizeType i) const
Definition: RegularSpatialGrid.hpp:169
const unsigned int M
A generic type that covers any element that is a metal.
Definition: AtomType.hpp:637
Definition of type traits.
Matrix1VectorBinaryTraits< E1, E2, MatrixVectorProduct< E1, E2 > >::ResultType prod(const MatrixExpression< E1 > &e1, const VectorExpression< E2 > &e2)
Definition: MatrixExpression.hpp:833
Definition: RegularSpatialGrid.hpp:84
CoordinatesValueType getYStepSize() const
Definition: RegularSpatialGrid.hpp:229
RegularSpatialGrid & assign(const GridExpression< E > &e)
Definition: RegularSpatialGrid.hpp:475
std::enable_if< IsScalar< T >::value, RegularSpatialGrid >::type & operator/=(const T1 &t)
Definition: RegularSpatialGrid.hpp:468
Definition: Vector.hpp:1053
SizeType getSize2() const
Definition: Math/Grid.hpp:308
SizeType getMaxSize3() const
Definition: RegularSpatialGrid.hpp:219
virtual ~RegularSpatialGrid()
Definition: RegularSpatialGrid.hpp:152
RegularSpatialGrid & minusAssign(const GridExpression< E > &e)
Definition: RegularSpatialGrid.hpp:489
Reference operator()(SizeType i)
Definition: RegularSpatialGrid.hpp:164
void transform(VectorArray< CVector< T, Dim > > &va, const CMatrix< T1, Dim, Dim > &xform)
Transforms each -dimensional vector in the array with the -dimensional square matrix xform.
Definition: VectorArrayFunctions.hpp:51
SizeType getMaxSize2() const
Definition: RegularSpatialGrid.hpp:214
void getLocalCoordinates(const V1 &world_coords, V2 &local_coords) const
Definition: RegularSpatialGrid.hpp:321
const unsigned int m
Specifies that the stereocenter has m configuration.
Definition: CIPDescriptor.hpp:116
Definition of grid data types.
RegularSpatialGrid(const GridDataType &data, const CoordinatesValueType &xs, const CoordinatesValueType &ys, const CoordinatesValueType &zs)
Definition: RegularSpatialGrid.hpp:120
bool containsLocalPoint(const V &pos) const
Definition: RegularSpatialGrid.hpp:343
Thrown to indicate that some requested calculation has failed.
Definition: Base/Exceptions.hpp:230
C CoordinatesValueType
Definition: RegularSpatialGrid.hpp:97
const unsigned int T
Specifies Hydrogen (Tritium).
Definition: AtomType.hpp:67
Definition of exception classes.
const unsigned int s
Specifies that the stereocenter has s configuration.
Definition: CIPDescriptor.hpp:81
SizeType getMaxSize() const
Definition: RegularSpatialGrid.hpp:204
CoordinatesValueType getZStepSize() const
Definition: RegularSpatialGrid.hpp:234
const unsigned int r
Specifies that the stereocenter has r configuration.
Definition: CIPDescriptor.hpp:76
Definition: Expression.hpp:120
SelfType ClosureType
Definition: RegularSpatialGrid.hpp:108
friend void swap(RegularSpatialGrid &usg1, RegularSpatialGrid &usg2)
Definition: RegularSpatialGrid.hpp:506
std::shared_ptr< SelfType > SharedPointer
Definition: RegularSpatialGrid.hpp:110
GD GridDataType
Definition: RegularSpatialGrid.hpp:98
RegularSpatialGrid< double > DRegularSpatialGrid
An unbounded dense regular grid in 3D space holding floating point values of type double.
Definition: RegularSpatialGrid.hpp:653
Grid & assign(const GridExpression< E > &e)
Definition: Math/Grid.hpp:405
The namespace of the Chemical Data Processing Library.
Definition: Matrix.hpp:1607
const GridDataType & getData() const
Definition: RegularSpatialGrid.hpp:393
RegularSpatialGrid & operator=(const GridExpression< E > &e)
Definition: RegularSpatialGrid.hpp:440
void resize(SizeType m, SizeType n, SizeType o, bool preserve=true, const ValueType &v=ValueType())
Definition: RegularSpatialGrid.hpp:516
std::conditional< std::is_const< GD >::value, typename GD::ConstReference, typename GD::Reference >::type Reference
Definition: RegularSpatialGrid.hpp:103
SizeType getSize3() const
Definition: RegularSpatialGrid.hpp:199
RegularSpatialGrid(const CoordinatesValueType &s)
Definition: RegularSpatialGrid.hpp:128
std::enable_if< IsScalar< T >::value, RegularSpatialGrid >::type & operator*=(const T1 &t)
Definition: RegularSpatialGrid.hpp:461
DataMode getDataMode() const
Definition: RegularSpatialGrid.hpp:159
bool isEmpty() const
Definition: RegularSpatialGrid.hpp:388
void getLocalCoordinates(SSizeType i, SSizeType j, SSizeType k, V &coords) const
Definition: RegularSpatialGrid.hpp:306
bool containsPoint(const V &pos) const
Definition: RegularSpatialGrid.hpp:333
Definition of matrix data types.
RegularSpatialGrid & operator=(const RegularSpatialGrid &usg)
Definition: RegularSpatialGrid.hpp:415
SizeType getSize1() const
Definition: RegularSpatialGrid.hpp:189
RegularSpatialGrid< float > FRegularSpatialGrid
An unbounded dense regular grid in 3D space holding floating point values of type float.
Definition: RegularSpatialGrid.hpp:648
const SelfType ConstClosureType
Definition: RegularSpatialGrid.hpp:109
CoordinatesValueType getZExtent() const
Definition: RegularSpatialGrid.hpp:270
SizeType getSize() const
Definition: Math/Grid.hpp:298
@ CELL
Definition: RegularSpatialGrid.hpp:92
void setZStepSize(const CoordinatesValueType &zs)
Definition: RegularSpatialGrid.hpp:249
Definition of vector data types.
const unsigned int C
Specifies Carbon.
Definition: AtomType.hpp:92
void setCoordinatesTransform(const T1 &xform)
Definition: RegularSpatialGrid.hpp:409
GD::ConstReference ConstReference
Definition: RegularSpatialGrid.hpp:104
CoordinatesValueType getYExtent() const
Definition: RegularSpatialGrid.hpp:262
void clear(const ValueType &v=ValueType())
Definition: RegularSpatialGrid.hpp:511