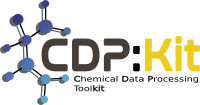 |
Chemical Data Processing Library C++ API - Version 1.1.1
|
Go to the documentation of this file.
29 #ifndef CDPL_CHEM_REACTION_HPP
30 #define CDPL_CHEM_REACTION_HPP
54 class ConstComponentAccessor;
55 class ComponentAccessor;
351 ConstComponentAccessor(
const ComponentAccessor& accessor):
352 reaction(accessor.reaction), compRole(accessor.compRole) {}
353 ConstComponentAccessor(
const Reaction* rxn,
unsigned int role):
354 reaction(rxn), compRole(role) {}
356 const Molecule& operator()(std::size_t idx)
const;
358 bool operator==(
const ConstComponentAccessor& accessor)
const;
361 const Reaction* reaction;
362 unsigned int compRole;
368 friend class Reaction;
369 friend class ConstComponentAccessor;
372 ComponentAccessor(Reaction* rxn,
unsigned int role):
373 reaction(rxn), compRole(role) {}
375 Molecule& operator()(std::size_t idx)
const;
377 bool operator==(
const ComponentAccessor& accessor)
const;
381 unsigned int compRole;
387 #endif // CDPL_CHEM_REACTION_HPP
ComponentIterator begin()
Returns a mutable iterator pointing to the beginning of the reaction components.
ComponentIterator removeComponent(const ComponentIterator &it)
Removes the reaction component specified by the iterator it.
Definition of the preprocessor macro CDPL_CHEM_API.
virtual Molecule & addComponent(unsigned int role)=0
Creates a new reaction component with the specified role.
Definition of the class CDPL::Util::IndexedElementIterator.
#define CDPL_CHEM_API
Tells the compiler/linker which classes, functions and variables are part of the library API.
GridEquality< E1, E2 >::ResultType operator==(const GridExpression< E1 > &e1, const GridExpression< E2 > &e2)
Definition: GridExpression.hpp:339
virtual bool containsComponent(const Molecule &mol) const =0
Tells whether the specified molecule is a component of this reaction.
A STL compatible random access iterator for container elements accessible by index.
Definition: IndexedElementIterator.hpp:125
static void registerCopyPostprocessingFunction(const CopyPostprocessingFunction &func)
virtual std::size_t getNumComponents() const =0
Returns the number of reaction components.
virtual void copy(const Reaction &rxn)=0
Replaces the current set of reaction components and properties by a copy of the components and proper...
Molecule.
Definition: Molecule.hpp:49
virtual void removeComponent(std::size_t idx)=0
Removes the reaction component at the specified index.
virtual Molecule & getComponent(std::size_t idx)=0
Returns a non-const reference to the reaction component at index idx.
ConstComponentIterator getComponentsEnd(unsigned int role) const
Returns a constant iterator pointing to the end of the reaction components with the specified role.
ConstComponentIterator begin() const
Returns a constant iterator pointing to the beginning of the reaction components.
virtual const Molecule & getComponent(std::size_t idx, unsigned int role) const =0
Returns a const reference to the reaction component at index idx in the list of components with the s...
ConstComponentIterator getComponentsBegin() const
Returns a constant iterator pointing to the beginning of the reaction components.
std::shared_ptr< Reaction > SharedPointer
A reference-counted smart pointer [SHPTR] for dynamically allocated Reaction instances.
Definition: Reaction.hpp:55
virtual const Molecule & getComponent(std::size_t idx) const =0
Returns a const reference to the reaction component at index idx.
void invokeCopyPostprocessingFunctions(const Reaction &src_rxn)
virtual std::size_t getNumComponents(unsigned int role) const =0
Returns the number of reaction components with the specified role.
ConstComponentIterator getComponentsEnd() const
Returns a constant iterator pointing to the end of the reaction components.
Util::IndexedElementIterator< Molecule, ComponentAccessor > ComponentIterator
A mutable random access iterator used to iterate over the components of the reaction.
Definition: Reaction.hpp:71
virtual unsigned int getComponentRole(const Molecule &mol) const =0
Returns the reaction role of the specified component.
A class providing methods for the storage and lookup of object properties.
Definition: PropertyContainer.hpp:75
ComponentIterator getComponentsBegin(unsigned int role)
Returns a mutable iterator pointing to the beginning of the reaction components with the specified ro...
virtual SharedPointer clone() const =0
Creates a copy of the current reaction state.
The namespace of the Chemical Data Processing Library.
ConstComponentIterator getComponentsBegin(unsigned int role) const
Returns a constant iterator pointing to the beginning of the reaction components with the specified r...
Reaction.
Definition: Reaction.hpp:52
ComponentIterator getComponentsBegin()
Returns a mutable iterator pointing to the beginning of the reaction components.
virtual void removeComponent(std::size_t idx, unsigned int role)=0
Removes the reaction component at index idx in the list of components with the specified role.
ConstComponentIterator end() const
Returns a constant iterator pointing to the end of the reaction components.
virtual ~Reaction()
Virtual destructor.
Definition: Reaction.hpp:78
ComponentIterator getComponentsEnd(unsigned int role)
Returns a mutable iterator pointing to the end of the reaction components with the specified role.
Util::IndexedElementIterator< const Molecule, ConstComponentAccessor > ConstComponentIterator
A constant random access iterator used to iterate over the components of the reaction.
Definition: Reaction.hpp:66
virtual std::size_t getComponentIndex(const Molecule &mol) const =0
Returns the index of the specified reaction component.
virtual void clear()=0
Removes all components and clears all properties of the reaction.
Definition of the class CDPL::Base::PropertyContainer.
virtual Molecule & getComponent(std::size_t idx, unsigned int role)=0
Returns a non-const reference to the reaction component at index idx in the list of components with t...
ComponentIterator getComponentsEnd()
Returns a mutable iterator pointing to the end of the reaction components.
virtual void swapComponentRoles(unsigned int role1, unsigned int role2)=0
Swaps the reaction roles of the component sets specified by role1 and role2.
virtual void removeComponents(unsigned int role)=0
Removes all components with the specified role.
std::function< void(Reaction &, const Reaction &)> CopyPostprocessingFunction
Definition: Reaction.hpp:73
ComponentIterator end()
Returns a mutable iterator pointing to the end of the reaction components.